Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial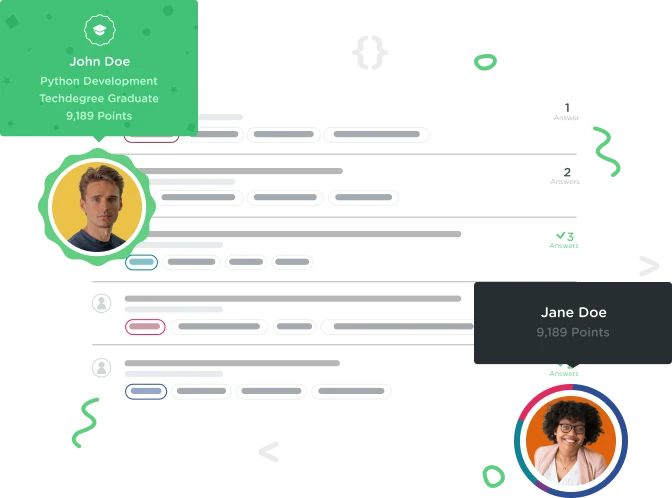
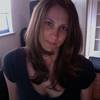
Shelley Hicklin
22,460 PointsFirst challenge step 3 asks: Override the Scan method to return true if any value in the array is a repeat of the value
What I have:
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
for(int i=0; i<sequence.Length; i++)
{
if(sequence[i] = sequence[i-1])
{
return true;
break;
}
}
}
}
}```
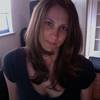
Shelley Hicklin
22,460 PointsAnswer is in the next challenge LOL
4 Answers
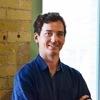
tjgrist
6,553 PointsFirst, you need two == signs to compare.
You might be getting an IndexOutOfRange Exception with your current code. You need to check the entire sequence but you have to stop checking at the last index - 1.
Here's what you're missing:
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
for(int i=1; i < sequence.Length ; i++)
{
if(sequence[i] == sequence[i - 1])
{
return true;
}
}
return false;
}
}
}

Melodie Joseph
3,372 PointsHi, silly question here but why do we initialize i = 1 and not to 0? Thanks!

Jose Perez
1,338 PointsI have a question o how would this work? can you please help me?
If we have an array as follow:
[1, 2, 3, 1, 5, 6]
if we start a index 1 and compare to index 0 and then increase every time. What will happen reaching index 3 and compare to index 2, it will not catch the 1 in index 0 since it will once compare to the one before it?
can you please help understand this?

Karol Pisula
2,578 Pointswhy there is no else
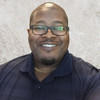
Donte Taylor
4,457 PointsQuestion for TJ Grist or anyone else that can provide some insight: For your "return false" statement on the outside of the for loop, wouldn't "false" be returned even if "true" was returned in the for loop? The reason I'm asking is because the exercise description mentions returning "true" if any value in the array is a repeat of the value. Thanks.
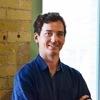
tjgrist
6,553 PointsDonte, no it wouldn't. If true is returned, the loop breaks and does not continue executing. Therefore, the false is not returned after true is returned.

Trung Nguyen
Courses Plus Student 2,224 Points@Melodie Joseph you start from the second element of the array, and then compare it with the first one Remember, i = 1 is the second element, the first one is 0
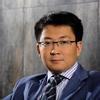
Andrei Li
34,475 PointsThank you @TJ Grist but for me, it hasn't worked. Compiler says about a problem with int and bool. Here is my solution.
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
bool isSame = false;
for(int i = 1 ; i < sequence.Length; i++)
{
if(sequence[i] == sequence[i-1])
{
isSame=true;
return isSame;
}
}
return isSame;
}
}
}

Daniel Yates
7,653 PointsThere's no need to return isSame within the for loop as you're already doing that afterwards.
Shelley Hicklin
22,460 PointsShelley Hicklin
22,460 PointsThe compiler error I'm getting is: RepeatDetector.cs(9,26): error CS0029: Cannot implicitly convert type
int' to
bool'