Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial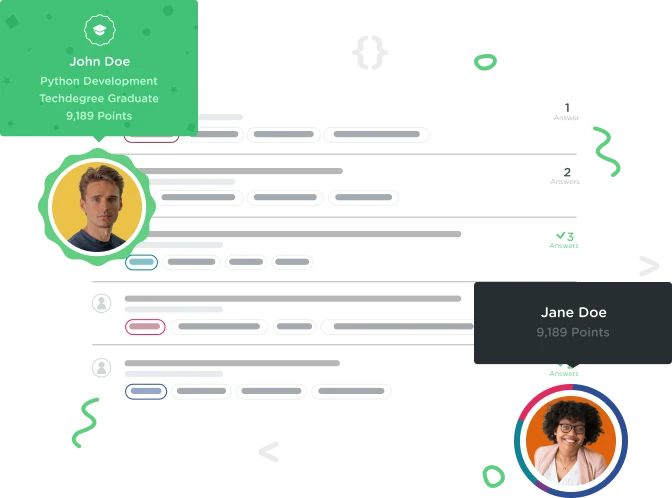

sharad pareek
279 PointsFirst, define a public constructor that expects a String argument named color. (Don't worry about adding any code inside
It shows bummer that you make the public string same as the class... I dont know what does it mean.
class GoKart {
private String color = "red";
public String getColor() {
return color;
}
}
5 Answers
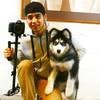
Jose Melendez
4,740 PointsA constructor must have the same name as the class. In this case the class name is GoKart (look at the very first line of code on the example you provided) so the name of the constructor should be GoKart.
-
The constructor should be public. So the constructor should look like this
public GoKart(){ }
-
In the directions, it says that the constructor should expect a string argument named color. The argument goes inside the empty parenthesis next to GoKart. Start be defining what type of data will be in the argument, in this case it will be a String (with a capital 'S') and the argument will be named 'color'. So your code should now look like this.
public GoKart(String color){ }
The code above is the final answer. Hope that helped :)
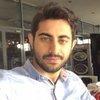
HIDAYATULLAH ARGHANDABI
21,058 Pointspublic GoKart(String color){
}

Pamela Pinheiro
533 Pointsclass GoKart { private String color = "red";
public color (String color) { this.color= color; }
public String getColor() { return color; }
}

vincent batteast
51,094 Pointsok thanks but I'm having trouble with this next part
class GoKart { private String Color;
public GoKart (String Color) { private this.Color = Color; }
public String getColor() { return Color; }
}

william williams
2,912 Pointsclass GoKart {
private String color = "red";
public GoKart(String color){
this.color = color;
}
public String getColor() {
return color;
}
}
and the next:
class GoKart {
private String color = "";
public GoKart(String color){
this.color = color;
}
public String getColor() {
return color;
}
}
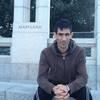
Muhammad Asif
Courses Plus Student 557 Pointsclass GoKart { private String color; public color(String color) { this.color = color; }
public String getColor() { return color; }
}
Passavudh Sabpisal
4,474 PointsPassavudh Sabpisal
4,474 PointsAwesome explaination :)