Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial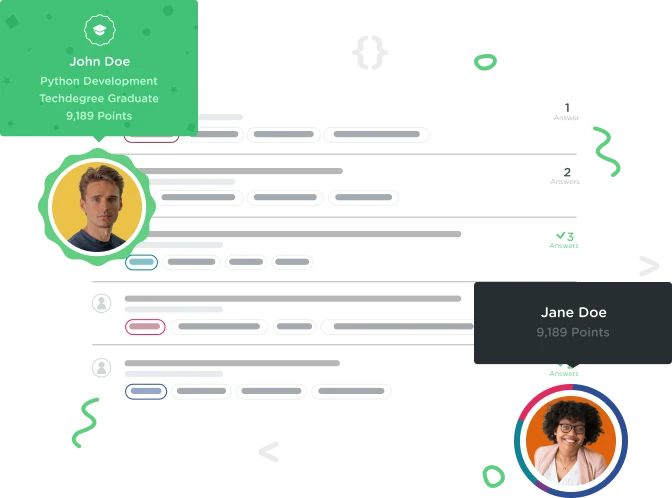
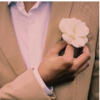
Muaaz Matwadia
Full Stack JavaScript Techdegree Graduate 19,327 PointsFix
How do I do this?
const languages = ['Python', 'JavaScript', 'PHP', 'Ruby', 'Java', 'C'];
const section = document.getElementsByTagName('section')[0];
// Accepts a language name and returns a wikipedia link
function linkify(language) {
const a = document.createElement('a');
const url = 'https://wikipedia.org/wiki/' + language + '_(programming_language)';
a.href = url;
return a;
}
// Creates and returns a div
function createDiv(language) {
const div = document.createElement('div');
const h2 = document.createElement('h2');
const p = document.createElement('p');
const link = linkify(language);
h2.textContent = language;
p.textContent = 'Information about ' + language;
link.appendChild(p);
div.appendChild(h2);
// Your code below
// end
return div;
}
for (let i = 0; i < languages.length; i += 1) {
let div = createDiv(languages[i]);
section.appendChild(div);
}
<!DOCTYPE html>
<html>
<head>
<title>Programming Languages</title>
<style>
body {
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>Programming Languages</h1>
<section></section>
<script src="app.js"></script>
</body>
</html>
2 Answers

Sander Heieren
12,613 PointsLet me know if you still haven't figured it out, and I will help you :)
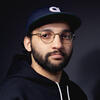
Thomas Davidson
7,243 PointsRead through the code carefully, find out which variable is storing the link, then use appendChild to add it into the dom.
Only one line of code needed to solve this one and it goes between the two comments.
It's good practice to read other peoples code and figure out how things are put together.
Hope that helps!
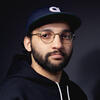
Thomas Davidson
7,243 Pointsdiv.appendChild(link)
Why this works
The for loop goes through each item of the languages variable and runs the create div function.
In this function on line 17 you can see that the link variable is created using another function to generate each link.
The challenge wants the link to appear after the headline which is currently stored as the text content of h2.
So h2 is appended to the div element first After which you must append the link variable with the above line of code
The div element is then returned and appended to the section variable which refrences an element within the dom.
Muaaz Matwadia
Full Stack JavaScript Techdegree Graduate 19,327 PointsMuaaz Matwadia
Full Stack JavaScript Techdegree Graduate 19,327 PointsYip,couldnt get it still would apprecite your help thanks!
Sander Heieren
12,613 PointsSander Heieren
12,613 PointsAzzie Fuzzie , What else do you need to append to div in order for it fully functioning?
Muaaz Matwadia
Full Stack JavaScript Techdegree Graduate 19,327 PointsMuaaz Matwadia
Full Stack JavaScript Techdegree Graduate 19,327 PointsOh I did it but wrote the code in the wrong place.Thank you guys so much Sander Heieren and Thomas Davidson really appriciate it.Thanks guys!