Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial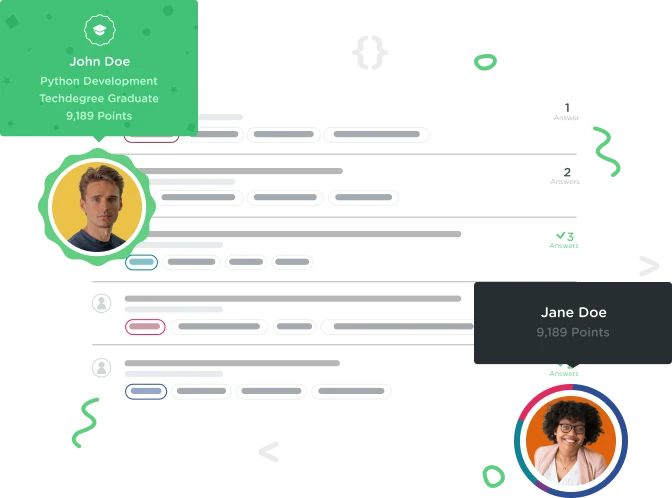

silashunter
Courses Plus Student 162 PointsFix my 2 problems in my outrageous version of Guessing game !
I didn't watch Kenneth's solution yet, so don't write here coding spoilers. I was making this alone for hours. Pretty proud about myself, but now I'm really stuck. Check 2 problems described in comments.
# pics random num between 1 and 10 - done
# asks user to guess - done
# when incorrect, tell user: too high, or too low - done
# let him guess again - done
# when it is right num, congratulate him - done
# limit his number of guesses - done
# tell him how many guesses he made - done
# differ from last try input - done
# differ from out of range inputs - done
# 1st problem:
# handle non integer inputs - !!!!!! - fail only on first input. It said: line 65, in <module> if conToInt == rnd:NameError: name 'conToInt' is not defined
# I defined it, firstly with None (not worked), secondly with 0 (worked, but it made run IF condition about Below range print. I don't want it.
# 2nd problem:
# nofity user about duplicite inputs - !!!!! - list fail on second input, why !
# it said: line 45, in <module> triedList = triedList.append(inp) AttributeError: 'NoneType' object has no attribute 'append'
import random
maxTry = 500
fromNum = 9 # range included
toNum = 999 # range included
print('Guess a number between {} and {} (both included) !'.format(fromNum, toNum))
print('You may guess {} times.'.format(maxTry))
rnd = random.randint(fromNum, toNum)
count = 0
# 1st problem: conToInt = 0
triedList = []
while count < maxTry:
inp = input('> ')
count = count + 1
conToStr = str(count)
lastInd = len(conToStr)
finalInt = int(conToStr[lastInd - 1])
# 2nd problem: triedList = triedList.append(inp)
try:
conToInt = int(inp)
except:
print("Hey ! A number, that's you have to put in !")
print('You wasted an attempt for this mistake ...')
if count == 11:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 1:
print('This was your {} attempt.'.format(conToStr + 'st'))
if count == 12:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 2:
print('This was your {} attempt.'.format(conToStr + 'nd'))
if count == 13:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 3:
print('This was your {} attempt.'.format(conToStr + 'rd'))
if finalInt >= 4:
print('This was your {} attempt.'.format(conToStr + 'th'))
if finalInt == 0:
print('This was your {} attempt.'.format(conToStr + 'th'))
if conToInt == rnd:
print('Congratulations ! You have got it !!!')
break
if conToInt < fromNum:
print('Hey ! You are out of the range (below) !!')
if count != maxTry:
print('You wasted an attempt for this mistake ... Try again !')
if count == maxTry:
print('You wasted an attempt for this mistake ... GAME OVER')
elif conToInt < rnd:
print('Incorrect: too low. Try again !')
if conToInt > toNum:
print('Hey ! You are out of the range (above) !!')
if count != maxTry:
print('You wasted an attempt for this mistake ... Try again !')
if count == maxTry:
print('You wasted an attempt for this mistake ... GAME OVER')
elif conToInt > rnd:
print('Incorrect: too high. Try again !')
5 Answers

Josiah McClellan
Courses Plus Student 7,869 PointsFirst problem:
When the try
block fails, you don't make any assignment to conToInt
, so the previous value is still there. When that value is an initial value of None, the code breaks. When that value is an initial value of 0, it prints the "below range" message because 0 is below the range. Otherwise, the value is the same as the last guess. There are many ways to solve this, but the quickest way would be to put a continue
statement as the last line of your except
block.
Second problem:
triedList.append
modifies the list in-place, instead of returning a copy with the new data. The first time you call triedList = triedList.append(inp)
, it sets the value of triedList
to the return of .append
, which is None
. The second time the line is run, triedList
is already None
, hence the error 'NoneType' object has no attribute 'append'
. Simply removing the first part of the line and leaving triedList.append(inp)
will solve that problem.
My thoughts: It's good to alternate between learning new things, and pushing your abilities with the things you already know. You should be proud for creating this game with such basic techniques, but now it is time for you to learn some more advanced python (or javascript, or ruby, whereever else this journey might take you). For instance, once you learn to use a switch block, you can say goodbye to those huge sections of if/elif/else statements, and I bet you won't miss them. Have fun!

silashunter
Courses Plus Student 162 PointsAbout 1st problem:
Not work as it should be

silashunter
Courses Plus Student 162 PointsSo quiet in python section. Nobody is coding in python ?
If I ask this trivial question (trivial to other coders) on Stackoverflow, they will give me minus points on my topic and it blocks my account from asking any new questions. It happend me once.
I can only ask decent ones according to their stupid rules. It's not place for beginners.
Anyone to help me with it please ? Cmon

silashunter
Courses Plus Student 162 Points2nd problem solved. I just changed line of my code up way in order.
triedList = []
while count < maxTry:
inp = input('> ')
count = count + 1
conToStr = str(count)
lastInd = len(conToStr)
finalInt = int(conToStr[lastInd - 1])
if inp in triedList:
print('You have it already put it before !')
triedList.append(inp)
try:
conToInt = int(inp)
except:
print("Hey ! A number, that's you have to put in !")
print('You wasted an attempt for this mistake ...')
#continue
1st problem solved too ! But in very bad way. I guess, in real world programming an apps, this is not acceptable.
Simply I had to:
put all code into try except blocks
make it duplicite for except part, because it's not possible to use continue outside of except block, after executing other part of code (outside of both blocks, as it was in first version on the top of this page - this is very important issue, I need to solve = again: HOW USE CONTINUE KEYWORD OUTSIDE OF EXCEPT BLOCK, PLACED UNDER REGULAR CODE; BUT EXECUTE IT WHEN EXCEPT BLOCK WILL OCCUR(after TRY block failure)
define input conversion to 1, since I'will get that undefined error, only in first non int input
add continue to end of except part
Is there better way to solve it?
# pics random num between 1 and 10 - done
# asks user to guess - done
# when incorrect, tell user: too high, or too low - done
# let him guess again - done
# when it is right num, congratulate him - done
# limit his number of guesses - done
# tell him how many guesses he made - done
# differ from last try input - done
# differ from out of range inputs - done
# nofity user about duplicite inputs - done
# handle non integer inputs - done (note special case for very first input)
import random
maxTry = 500
fromNum = 1 # range included
toNum = 999 # range included
print('Guess a number between {} and {} (both included) !'.format(fromNum, toNum))
print('You may guess {} times.'.format(maxTry))
rnd = random.randint(fromNum, toNum)
count = 0
triedList = []
while count < maxTry:
inp = input('> ')
count = count + 1
conToStr = str(count)
lastInd = len(conToStr)
finalInt = int(conToStr[lastInd - 1])
if inp in triedList:
print('You have it already put it before !')
triedList.append(inp)
try:
conToInt = int(inp)
if count == 11:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 1:
print('This was your {} attempt.'.format(conToStr + 'st'))
if count == 12:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 2:
print('This was your {} attempt.'.format(conToStr + 'nd'))
if count == 13:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 3:
print('This was your {} attempt.'.format(conToStr + 'rd'))
if finalInt >= 4:
print('This was your {} attempt.'.format(conToStr + 'th'))
if finalInt == 0:
print('This was your {} attempt.'.format(conToStr + 'th'))
if conToInt == rnd:
print('Congratulations ! You have got it !!!')
break
if conToInt < fromNum:
print('Hey ! You are out of the range (below) !!')
if count != maxTry:
print('You wasted an attempt for this mistake ... Try again !')
if count == maxTry:
print('You wasted an attempt for this mistake ... GAME OVER')
elif conToInt < rnd:
print('Incorrect: too low. Try again !')
if conToInt > toNum:
print('Hey ! You are out of the range (above) !!')
if count != maxTry:
print('You wasted an attempt for this mistake ... Try again !')
if count == maxTry:
print('You wasted an attempt for this mistake ... GAME OVER')
elif conToInt > rnd:
print('Incorrect: too high. Try again !')
except:
print("Hey ! A number, that's you have to put in !")
print('You wasted an attempt for this mistake ...')
conToInt = 1
if count == 11:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 1:
print('This was your {} attempt.'.format(conToStr + 'st'))
if count == 12:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 2:
print('This was your {} attempt.'.format(conToStr + 'nd'))
if count == 13:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 3:
print('This was your {} attempt.'.format(conToStr + 'rd'))
if finalInt >= 4:
print('This was your {} attempt.'.format(conToStr + 'th'))
if finalInt == 0:
print('This was your {} attempt.'.format(conToStr + 'th'))
if conToInt == rnd:
print('Congratulations ! You have got it !!!')
break
if conToInt < fromNum:
print('Hey ! You are out of the range (below) !!')
if count != maxTry:
print('You wasted an attempt for this mistake ... Try again !')
if count == maxTry:
print('You wasted an attempt for this mistake ... GAME OVER')
elif conToInt < rnd:
print('Incorrect: too low. Try again !')
if conToInt > toNum:
print('Hey ! You are out of the range (above) !!')
if count != maxTry:
print('You wasted an attempt for this mistake ... Try again !')
if count == maxTry:
print('You wasted an attempt for this mistake ... GAME OVER')
elif conToInt > rnd:
print('Incorrect: too high. Try again !')
continue

Josiah McClellan
Courses Plus Student 7,869 PointsIf I understand you, my solution to problem 1 wasn't what you wanted because you want it to print 'This was your {} attempt.' even if they didn't enter a number. Here is one way to do that with what you know. Instead of putting continue
directly in the except
block, try this:
try:
conToInt = int(inp)
except:
contToInt = None
print("Hey ! A number, that's you have to put in !")
print('You wasted an attempt for this mistake ...')
...then after you print the attempt number, but before you try to use conToInt, do this:
if conToInt == None: continue
You also won't need to give conToInt an initial value since it will always get a value.
If you want to know how to make the whole thing even shorter and simpler, just ask. I don't know if you want me to tell you, so I'm only answering your two problems.

silashunter
Courses Plus Student 162 PointsThanks for reply, I was waiting for you. Come more often, it seems nobody cares :( I'm happy for any help.
Btw. Kenneth is away, or just avoiding me ? ^^
Your solution didn't work because there is other line down the code, which has problem with comparing Nonetype and INT
AND I was wrong, abou that long duplicite code. It is wrong. I cannot define first try to 1. Why nobody tried my code .....-.-

Josiah McClellan
Courses Plus Student 7,869 PointsOops, I wrote contToInt
instead of conToInt
above, that's why it didn't work. Here is what I meant, and it works:
import random
maxTry = 500
fromNum = 9 # range included
toNum = 999 # range included
print('Guess a number between {} and {} (both included) !'.format(fromNum, toNum))
print('You may guess {} times.'.format(maxTry))
rnd = random.randint(fromNum, toNum)
count = 0
triedList = []
while count < maxTry:
inp = input('> ')
count = count + 1
conToStr = str(count)
lastInd = len(conToStr)
finalInt = int(conToStr[lastInd - 1])
triedList.append(inp)
try:
conToInt = int(inp)
except:
conToInt = None
print("Hey ! A number, that's you have to put in !")
print('You wasted an attempt for this mistake ...')
if count == 11:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 1:
print('This was your {} attempt.'.format(conToStr + 'st'))
if count == 12:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 2:
print('This was your {} attempt.'.format(conToStr + 'nd'))
if count == 13:
print('This was your {} attempt.'.format(conToStr + 'th'))
elif finalInt == 3:
print('This was your {} attempt.'.format(conToStr + 'rd'))
if finalInt >= 4:
print('This was your {} attempt.'.format(conToStr + 'th'))
if finalInt == 0:
print('This was your {} attempt.'.format(conToStr + 'th'))
if conToInt == None:
continue
if conToInt == rnd:
print('Congratulations ! You have got it !!!')
break
if conToInt < fromNum:
print('Hey ! You are out of the range (below) !!')
if count != maxTry:
print('You wasted an attempt for this mistake ... Try again !')
if count == maxTry:
print('You wasted an attempt for this mistake ... GAME OVER')
elif conToInt < rnd:
print('Incorrect: too low. Try again !')
if conToInt > toNum:
print('Hey ! You are out of the range (above) !!')
if count != maxTry:
print('You wasted an attempt for this mistake ... Try again !')
if count == maxTry:
print('You wasted an attempt for this mistake ... GAME OVER')
elif conToInt > rnd:
print('Incorrect: too high. Try again !')
But there are still many things you can do to improve your code. For instance, when you reach the 111th attempt, it prints 111st instead. You can fix that, and make your code smaller and more readable, and eliminate three variables from the while
block by defining a function, like this:
def printAttempt(count):
countStr = str(count)
suffix = 'th'
if count%100 < 10 or count%100 > 20:
if countStr[-1] == '1': suffix = 'st'
if countStr[-1] == '2': suffix = 'nd'
if countStr[-1] == '3': suffix = 'rd'
print ('This was your {} attempt'.format( countStr + suffix ))
Then, instead of that first big block of if/elif statements, you can just call printAttempt(count)
I think you've done great with what you know, but if you take some more classes and learn some new features, everything will get much easier. Then you can make an even cooler project. I am leaving treehouse for a while after today, because I need to save money, but I wish you good luck in your studies.

silashunter
Courses Plus Student 162 PointsI don't want use foreign concepts to me, which I didn't learn yet.
I was trying it this way, but it doesn't work. Why ?
if str(count)[-1:-3] == 11:
print('This was your {} attempt.'.format(conToStr + 'th'))
This didn't work either. But it should. I really don't get it:
if str(count)[-1] == 1 and str(count)[-2] == 1:
print('This was your {} attempt.'.format(conToStr + 'th'))

silashunter
Courses Plus Student 162 Pointsthis forum is so dead I wonder why is this paysite...
I can't wait 24 hours for every next answer ...

silashunter
Courses Plus Student 162 PointsI have made it ! It's hilarious, muahaha !
import random
maxTry = 500
fromNum = 1 # range included
toNum = 999 # range included
print('Guess a number between {} and {} (both included) !'.format(fromNum, toNum))
print('You may guess {} times.'.format(maxTry))
rnd = random.randint(fromNum, toNum)
count = 0
triedList = []
while count < maxTry:
inp = input('> ')
count = count + 1
conToStr = str(count)
lastInd = len(conToStr)
finalInt = int(conToStr[lastInd - 1])
if inp in triedList:
print('You have it already put it before !')
triedList.append(inp)
try:
conToInt = int(inp)
except:
conToInt = None
print("Hey ! A number, that's you have to put in !")
print('You wasted an attempt for this mistake ...')
# managing ordinal numbers during count changing
# 1, 11
if count == 1: # 1
print('This was your {} attempt.'.format(conToStr + 'st'))
elif count == 11: # 11
print('This was your {} attempt.'.format(conToStr + 'th'))
elif lastInd == 2 and conToStr[-1] == '1' and conToStr[-2] != '1': # 21, 31, ...
print('This was your {} attempt.'.format(conToStr + 'st'))
elif lastInd > 2 and conToStr[-1] == '1' and conToStr[-2] != '1': # 101, 201, ...
print('This was your {} attempt.'.format(conToStr + 'st'))
elif lastInd > 2 and conToStr[-1] == '1' and conToStr[-2] == '1': # 111, 211, ...
print('This was your {} attempt.'.format(conToStr + 'th'))
# 2, 12
if count == 2: # 2
print('This was your {} attempt.'.format(conToStr + 'nd'))
elif count == 12: # 12
print('This was your {} attempt.'.format(conToStr + 'th'))
elif lastInd == 2 and conToStr[-1] == '2' and conToStr[-2] != '1': # 22, 32, ...
print('This was your {} attempt.'.format(conToStr + 'nd'))
elif lastInd > 2 and conToStr[-1] == '2' and conToStr[-2] != '1': # 102, 202, ...
print('This was your {} attempt.'.format(conToStr + 'nd'))
elif lastInd > 2 and conToStr[-1] == '2' and conToStr[-2] == '1': # 112, 212, ...
print('This was your {} attempt.'.format(conToStr + 'th'))
# 3, 13
if count == 3: # 3
print('This was your {} attempt.'.format(conToStr + 'rd'))
elif count == 13: # 13
print('This was your {} attempt.'.format(conToStr + 'th'))
elif lastInd == 2 and conToStr[-1] == '3' and conToStr[-2] != '1': # 23, 33, ...
print('This was your {} attempt.'.format(conToStr + 'rd'))
elif lastInd > 2 and conToStr[-1] == '3' and conToStr[-2] != '1': # 103, 203, ...
print('This was your {} attempt.'.format(conToStr + 'rd'))
elif lastInd > 2 and conToStr[-1] == '3' and conToStr[-2] == '1': # 113, 213, ...
print('This was your {} attempt.'.format(conToStr + 'th'))
# all other
if count not in [1, 11, 2, 12, 3, 13] and conToStr[-1] not in ['1', '2', '3']:
print('This was your {} attempt.'.format(conToStr + 'th'))
if conToInt == None:
continue
if conToInt == rnd:
print('Congratulations ! You have got it !!!')
break
if conToInt < fromNum:
print('Hey ! You are out of the range (below) !!')
if count != maxTry:
print('You wasted an attempt for this mistake ... Try again !')
if count == maxTry:
print('You wasted an attempt for this mistake ... GAME OVER')
elif conToInt < rnd:
print('Incorrect: too low. Try again !')
if conToInt > toNum:
print('Hey ! You are out of the range (above) !!')
if count != maxTry:
print('You wasted an attempt for this mistake ... Try again !')
if count == maxTry:
print('You wasted an attempt for this mistake ... GAME OVER')
elif conToInt > rnd:
print('Incorrect: too high. Try again !')
Josiah McClellan: Sorry, I though that suffix, is a python keyword like str.suffix. Sorry for that Jesus..... Now I see, it is normal beginners concept Me stupid
silashunter
Courses Plus Student 162 Pointssilashunter
Courses Plus Student 162 PointsWhere is Kenneth
panic
\o/