Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial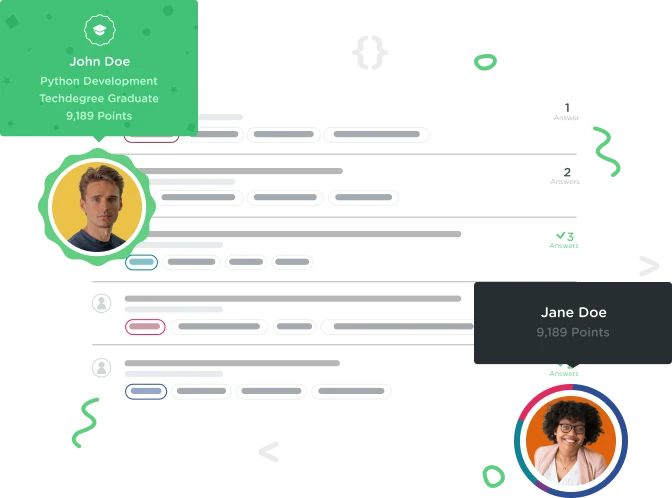

Kenneth Phillips
Courses Plus Student 10,188 PointsfixedVideoTitle()
Here is the error:
./QuickFix.java:22: error: incompatible types: Object cannot be converted to Video newMap.put(newTitle, entry.getValue()); ^ Note: Some messages have been simplified; recompile with -Xdiags:verbose to get full output 1 error
I don't understand this because the value of the set is a video.
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.List;
import java.util.HashMap;
import java.util.Map;
public class QuickFix {
public void addForgottenVideo(Course course) {
List<Video> newList = course.getVideos();
// TODO(1): Create a new video called "The Beginning Bits"
Video video = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
newList.add(1, video);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Map<String, Video> newMap = new HashMap<String, Video>(videosByTitle(course));
for (Map.Entry entry : newMap.entrySet()) {
if (entry.getKey() == oldTitle) {
newMap.remove(entry.getKey(), entry.getValue());
newMap.put(newTitle, entry.getValue());
}
}
}
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> videoMap = new HashMap<String, Video>();
for (Video video : course.getVideos()) {
videoMap.put(video.getTitle(), video);
}
return videoMap;
}
}
1 Answer

Umesh Ravji
42,386 PointsHi Kenneth, you can cast the entry.getValue()
object to type Video
but then you will encounter an ConcurrentModificationException
.. read more about it here: http://stackoverflow.com/questions/12177889/java-util-concurrentmodificationexception-while-accessing-hashmap
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Video video = this.videosByTitle(course).get(oldTitle);
// Update video title here. May want to check to see if video object isn't null first.
}
You just wrote a method videosByTitle(Course course)
which returns a Map
(mapping titles to videos), so use that method to find the video you need, rather than iterating over entire collection.
Kenneth Phillips
Courses Plus Student 10,188 PointsKenneth Phillips
Courses Plus Student 10,188 PointsI don't know how to use videosByTitle without iterating over the collection. I don't know how to remove the oldvidoetitle and video and then put in the new video title and put the video back in without an error.
Umesh Ravji
42,386 PointsUmesh Ravji
42,386 PointsI'll change the code a little to hopefully make it more clearer.
videosByTitle(Course course)
to get theMap
(that maps titles to video objects).video
object that needs to be updated using theget()
method of theMap
.video
object, update it'stitle
using thesetTitle(String title)
method. There is no need to remove or add anyvideo
objects, simply update thetitle
property of the existingvideo
object.