Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial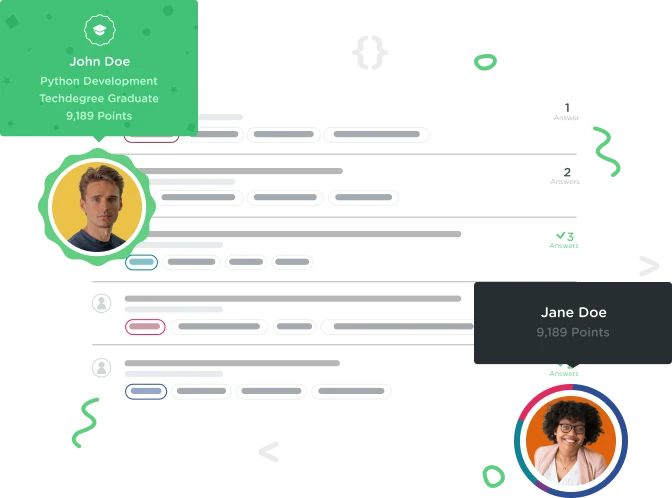

Duke Branding
3,606 PointsFizz Buzz!
Just curious, but i wonder if anyone else has used a different method than i did for the Fizz Buzz challenge.
Here's what i did:
for ( i=1; i <= 100; i++ )
{
if ( i % 3 === 0 )
{
console.log("fizz");
}
else if ( i % 5 === 0 )
{
console.log("buzz");
}
else
{
console.log(i);
}
}
6 Answers

Andrew Mosley
12,328 PointsIf you're trying for smallest amount of code this works:
for(i=0;i<100;)console.log((++i%3?'':'Fizz')+(i%5?'':'Buzz')||i)

Andrew Mosley
12,328 PointsYou'll notice if you pass your loop into the console that you won't actually see "Fizz Buzz" on multiples of 15. That's because your loop is structured to call "fizz" first and then increment the value of i.
Try something like this:
for ( i=1; i <= 100; i++ ) { if (i % 15 === 0) { console.log("Fizz Buzz")} else if ( i % 3 === 0 ) { console.log("fizz"); } else if ( i % 5 === 0 ) { console.log("buzz"); } else { console.log(i); } }

Duke Branding
3,606 Pointsi chose a different way to do the assigment. Thanks for pointing out my oversight!
var i = 1;
var inc = function(){ i++}
do
{
if ( i % 15 === 0 )
{
console.log("fizz buzz 15");
inc();
}
else if ( i % 5 === 0 )
{
console.log("buzz 5");
inc();
}
else if ( i % 3 === 0 )
{
console.log("fizz 3")
inc();
}
else
{
console.log(i);
inc();
}
}
while ( i < 101 );

Duke Branding
3,606 PointsAndrew Mosley , i completely skipped over that part of the assignment! You're right!

James Gill
Courses Plus Student 34,936 PointsA little off-topic, but FizzBuzz is a good, small demo of how elegant Python is compared to JS:
for numbers in range(1,101):
if numbers % 3 == 0 and numbers % 5 == 0:
print "FizzBuzz"
elif numbers % 3 == 0:
print "Fizz"
elif numbers % 5 == 0:
print "Buzz"
else:
print numbers
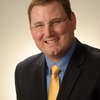
Mike Rolih
9,766 PointsWondering why the instructor didn't mention the concept of the "else if ()" subset to the if/else loop? Anyway, here is what I wrote and it worked just fine:
for (var i = 1; i < 100; i++) { if (i % 3 === 0 && i % 5 ===0) { console.log("fizzbuzz"); } else if (i % 3 ===0) { console.log("fizz"); } else if (i % 5 === 0) { console.log("buzz"); } else { console.log(i); } }