Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial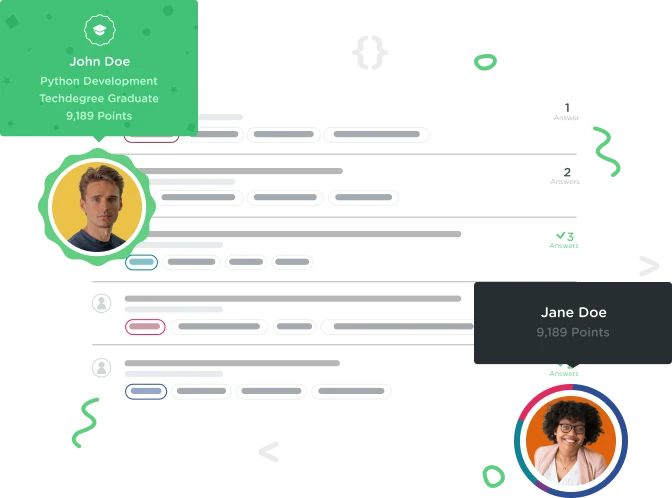

nader adam
Courses Plus Student 548 Pointsfizz buzz
nter your FizzBuzz solution here! Before you hit submit, make the following changes:
Step 1: Enter your code in between the comments shown below. The code is going inside a "function" that will help verify your solution.
Step 2: Change your variable/constant name that you are checking in each step to n. For example if (n % 3 == 0). Note: You don't need to create n, it is already provided.
Step 3: Change all your print statements to return statements. For example: print("FizzBuzz") becomes return "FizzBuzz".
While these are very specific directions, they allow me to verify your solution precisely over a large number of possible answers.
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
// End code
return "\(n)"
}
1 Answer
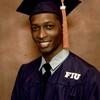
Dane Parchment
Treehouse Moderator 11,077 PointsHave you tried to problem itself? What specifically are you confused about? FizzBuzz is a famous programming question because it stumps even veteran programmers while being a very straightforward an easy to implement program. So I guess I will walk you through it, assuming that you either haven't tried it yourself or might be having problems with it.
So first and foremost, we need to figure out what FizzBuzz is asking and how to go about doing it.
We know that in order to solve this we need to check and see if the value entered is divisible by either 3, 5, or both. So let's set up a little skeleton code in order to solve this:
I have created a switch statement for ease of readability in order to determine what the divisibility of n of both 3 and 5, I have set it up in the form of a tuple (an ordered list of elements in this case a tuple of two booleans: (n % 3, n % 5) -> (bool , bool), if that is confusing you then don't worry, that is just the mathematical definition of a tuple, but it isn't really important that you understand the definition yet.
func fizzBuzz(n: Int) -> String {
switch(n % 3 == 0, n % 5 == 0) {
}
return "\(n)"
}
Now that I have that out of the way, we need to start testing our cases, for the first case let's see if the right value is true and left value is false. This is the case if the number is divisible by 3 and not 5 and as such we should return Fizz:
func fizzBuzz(n: Int) -> String {
switch(n % 3 == 0, n % 5 == 0) {
case(true, false):
return "Fizz"
}
return "\(n)"
}
Now let's cover Buzz
func fizzBuzz(n: Int) -> String {
switch(n % 3 == 0, n % 5 == 0) {
case(true, false):
return "Fizz"
case(false, true):
return "Buzz"
}
return "\(n)"
}
Finally we take care of the FizzBuzz portion
func fizzBuzz(n: Int) -> String {
switch(n % 3 == 0, n % 5 == 0) {
case(true, false):
return "Fizz"
case(false, true):
return "Buzz"
case(true, true):
return "FizzBuzz"
}
return "\(n)"
}
Now if none of the following happen (number is not divisible by either, lets just return the n:
func fizzBuzz(n: Int) -> String {
switch(n % 3 == 0, n % 5 == 0) {
case(true, false):
return "Fizz"
case(false, true):
return "Buzz"
case(true, true):
return "FizzBuzz"
default:
return "\(n)"
}
}
And we are done, if you need help with anything or didn't understand something let me know.