Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial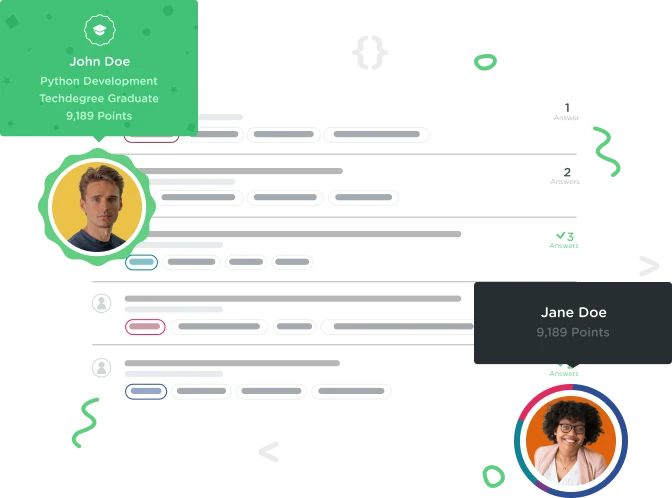
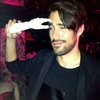
Adrian Torrente Tenreiro
12,540 PointsFIZZBUZZ Cant make it work (
the "return" are right correct? Any tips?
func fizzBuzz(n: Int) -> String
{
let resulto = (n % 3, n % 5)
switch resulto
{
case (0, _): return "Fizz"
case (_, 0): return "Buzz"
case (0, 0): return "FizzBuzz"
default: ()
}
return "\(n)"
}
2 Answers
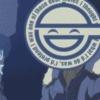
Jonathan Ruiz
2,998 PointsHi Adrian for this code challenge you don't need to use a switch statement. The main thing is to write out the logical operations and in the correct order as well. The first thing you want to write would be the if statement to check if a number is divisible by 3 and 5. This way you can print FizzBuzz.
If you don't check this as the first if statement you might not have that line of code executed even though a number would be dividable by both 3 and 5.
func fizzBuzz(n: Int) -> String {
if (n % 3 == 0) && (n % 5 == 0) {
return "FizzBuzz"
} else if (n % 3 == 0) {
return "Fizz"
} else if (n % 5 == 0) {
return "Buzz"
}
return "\(n)"
}
Hope this helps
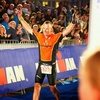
Steve Hunter
57,712 PointsHi Adrian,
As Jonathan said, this is just down to the order your tests are in. Do your 'fizzbuzz' test first and your switch statement is fine:
func fizzBuzz(n: Int) -> String {
let resulto = (n % 3, n % 5)
switch resulto {
case (0, 0): return "FizzBuzz"
case (0, _): return "Fizz"
case (_, 0): return "Buzz"
default: return "\(n)"
}
}
Otherwise, your line of execution stops at the case(0, _)
even though that particular number also passes the case (0, 0)
test. So, if you filter out your fizzbuzz results first, you can then worry about the individual fizz and buzz ones. You can also do the return "\(n)"
thing in your default
case.
I hope that helps,
Steve.
Adrian Torrente Tenreiro
12,540 PointsAdrian Torrente Tenreiro
12,540 PointsWorks in Xcode but it doesnt seem to work in the TH but i had similar issues before