Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial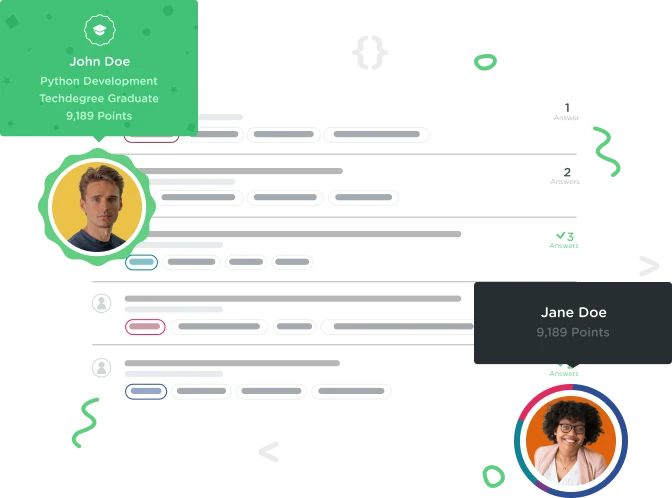
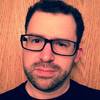
Chris Shaffer
12,030 PointsFizzBuzz Challenge could be far more educational
The FizzBuzz can be solved with a simple set of variables and an if/else if statement:
func fizzBuzz(n: Int) -> String {
var fizz = n % 3 == 0
var buzz = n % 5 == 0
let fb = fizz && buzz
if fb {
return "FizzBuzz"
} else if fizz && !buzz {
return "Fizz"
} else if !fizz && buzz {
return "Buzz"
}
return "\(n)"
}
Unfortunately, this doesn't take advantage of any of the other useful conditional and check statements we've learned.
I've coming from a strong JS background, so I immediately looked at this and said, "It's way too easy, ", we could be using for, switch and arrays to solve this but the challenge itself has none of those requirements.
I would suggest adding the caveat that the numbers checked need to be in a certain range and that we need to check against them before returning the value or Fizz/Buzz/FizzBuzz.
For example, simply adding the requirement that the range be 1 - 100 would require the use of the range operator and probably a for statement.
Adding an additional requirement that the numbers be stored in an array would incorporate the append method.
These concepts were easy for me to grasp coming from JS, but would be much more challenging for a person not familiar; it is far more educational to be challenged to combine all of these problem solving methods rather than a simple if/else statement.
1 Answer
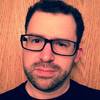
Chris Shaffer
12,030 PointsBecause without that you are not controlling for situations that would essentially be FizzBuzz.
While itβs not likely the first if statement would be passed, this is simply a best practice.
In this case, itβs probably overkill but it would always pass a unit test. Without that there are values that might not.
Herman Kwan
5,062 PointsHerman Kwan
5,062 PointsWhy is !buzz and !fizz necessary? Couldn't it have been much easier just doing
else if fizz { }