Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial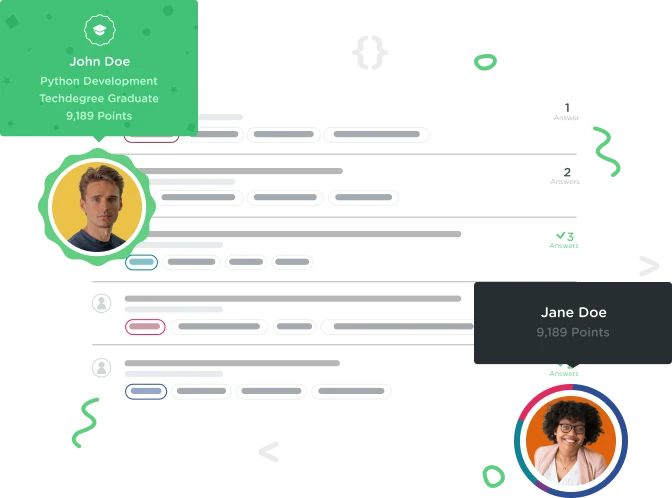
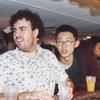
Brent Liang
Courses Plus Student 2,944 PointsFizzBuzz code challenge
Error message shows up saying that the last line will never be executed, and also the cases in switch are not exhaustive. How should I fix?
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch n%3 - n%5{
case 0: return "FizzBuzz"
case -4 ... -1: return "Fizz"
case 1...2: return "Buzz"}
// End code
return "\(n)"
}
2 Answers
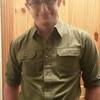
Lucas Smith
11,616 PointsGreat catch with the default case there Jared!
The only thing that I would add is that a default case needs to be at the bottom of any switch statement, just to make sure that in the event that one of the cases isn't met, that the switch will still terminate if need be. The code challenge DOES say that you can ignore the default case, but I think that that may simply be some poor word choice in the instructions since that is a requirement for switches.
As for the message that the
return "(\n)"
call will never be executed, that is correct. Since a switch is designed to always have at least one case satisfied, and all of the cases in this switch are set to return a value, the function will be returned from by the end of the switch which will preclude that last return statement from executing. A simple work around for that would be to change the default case to:
default: break
This hops out of the switch, and allows the function to reach the final return statement in the function. Quick note about this, you can just avoid this all together by making the default case the last return statement.
I hope this helps! Happy coding!
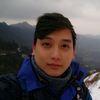
Jared Chu
4,090 PointsThis code shoud be:
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch n%3 - n%5{
case 0: return "FizzBuzz"
case -4 ... -1: return "Fizz"
case 1 ... 2: return "Buzz"
default: return ""
}
// End code
return "\(n)"
}
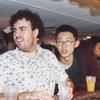
Brent Liang
Courses Plus Student 2,944 PointsYep it works, Thanks Jared!
Brent Liang
Courses Plus Student 2,944 PointsBrent Liang
Courses Plus Student 2,944 PointsWow Lucas such a detailed answer!
Thank you so much!!