Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial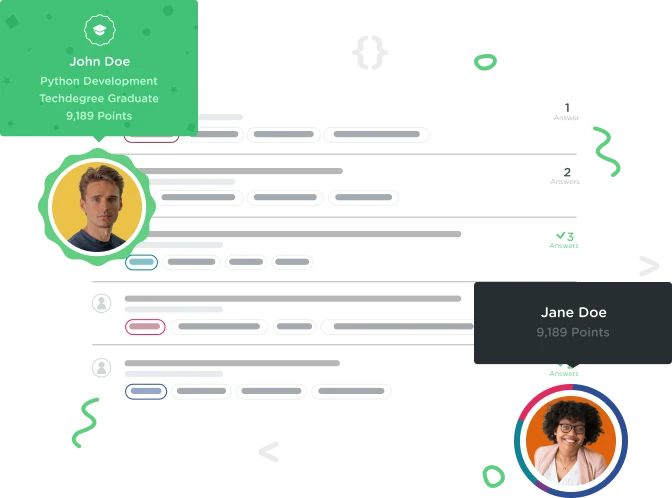

Sharon Scheel
3,206 Pointsfizzbuzz extra credit
I got this to run almost perfectly with the code below. When it runs, the if value in the center does not replace the divided value; the name prints above the number. I tried commenting out one of the values and it ran perfectly with any two values. I have also tried changing the order of the if values to no avail. Why is it running perfectly with 2 values but not with three values?
for (var number = 100; number; number = number - 1) {
if (number % 15 == 0) {
console.log("fizzbuzz");
}
if (number % 3 == 0) {
console.log("fizz");
}
if (number % 5 == 0) {
console.log("buzz");
}
else {
console.log(number);
}
}
Thanks so much for any help someone can give me on this.
11 Answers
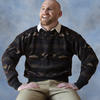
Kevin Kenger
32,834 PointsHey Sharon,
When you want to execute multiple conditions, use else if
.
for (var number = 100; number; number = number - 1) {
if (number % 15 == 0) {
console.log("fizzbuzz");
}
else if (number % 3 == 0) {
console.log("fizz");
}
else if (number % 5 == 0) {
console.log("buzz");
}
else {
console.log(number);
}
}
This way, if one condition is false, it will move on to the next one. I'm pretty sure that because of the way you had your code set up, multiple if blocks were being run, instead of one block with multiple conditions. That's why you were seeing both multiples of 3 as well as the word "fizz."
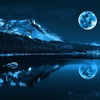
Daniel Banta
4,857 PointsWhy is this extra credit question presented before the instructor has covered the code necessary to complete it? Or am I missing something?

Sharon Scheel
3,206 PointsThank you, thank, thank you!! That was so simple, wish the instructor had mentioned 'else if' in the video.
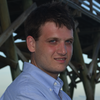
Steven Byington
13,584 PointsAnother way to write out the code for the same effect would be wrapping an if/else statement inside another if statement. Here is my code:
for (var n=1; n<=100; n++) {
if (n%5 === 0){
if (n%3 ===0) {
console.log("fizzbuzz");
}
else {
console.log("buzz");
}
}
else if (n%3 === 0){
console.log("fizz");
}
else {
console.log(n);
}
}

Hung Pham Viet
9,104 PointsHere is mine using WHILE loop:
var number = 100;
while (number) {
if (number % 15 == 0) {
console.log("fizzbuzz");
} else if (number % 3 == 0) {
console.log("fizz");
} else if (number % 5 == 0) {
console.log("buzz");
} else {
console.log(number);
}
number = number - 1;
}

Sharon Scheel
3,206 PointsThank you so much for taking the time to explain all this. I really appreciate it. Regarding the order of the numbers 3 and 5 that occurred when I was changing the order to see if it would make any difference. I forgot to change it back before I copy and pasted it into to my message. I did correct the order before I received Keven's response so I was able to run my code successfully; although as someone else later pointed out I forgot change fizz and buzz to correspond with the correct number. Have a great day!
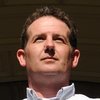
Phil Pickering
1,343 PointsJust adding my version of FizzBuzz into the conversation :)
for (var i = 1; i <= 100; i++) {
if (i%3 === 0 && i%5 === 0) {
console.log("FizzBuzz");
} else if (i%3 === 0) {
console.log("Fizz");
} else if (i%5 === 0) {
console.log("Buzz");
} else {
console.log(i);
}
}
Couple of things I've used that I found outside of "class":
- i++ is shorthand for i = i + 1
- && is the logical operand, AND. Both expressions need to evaluate as true for the condition to be true.
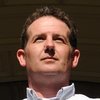
Phil Pickering
1,343 PointsSorry, playing around and came up with an alternative version which consists of solely if statements (no if else statements):
for (var number = 1; number <= 100; number++) {
var output = "";
if (number%3 === 0) {
output = output + "Fizz";
}
if (number%5 === 0) {
output = output + "Buzz";
}
if (!output) {
output = number;
}
console.log(output);
}
This version starts with an empty string (output) then uses concatenation to add "Fizz" or "Buzz" to the string. It doesn't need another if statement to check for "FizzBuzz"... that case has already been covered.
Finally, if the output string is still empty (i.e. it's a falsy), the current number is assigned to output.
Falade Mayowa
6,213 Pointsfor (var count= 100; count> 0; count--) { if (count% 15 == 0) { console.log("fizzbuzz"); }
else if (count% 5 == 0) {
console.log("buzz");
}
else if (count% 3 == 0) {
console.log("fizz");
}
else {
console.log(count);
}
}
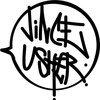
Vince Usher
7,159 Pointsfor(i=0;i<100;)console.log((++i%3?'':'Fizz')+(i%5?'':'Buzz')||i)
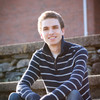
Andrew Lincoln
1,732 Pointsfor (var number = 100; number > 0; number--) {
if (number % 15 == 0) {
console.log("fizzbuzz");
}
else if (number % 5 == 0) {
console.log("buzz");
}
else if (number % 3 == 0) {
console.log("fizz");
}
else {
console.log(number);
}
}
Had to fix up Kevin's code. This should work properly. When your loop finds a number divisible by 15. As soon as it hits the first 'if' block, and the condition is true, your console will print out "fizzbuzz" and it will break out of the 'if else/if ' block all together and continue with the loop. It will ignore everything subsequent of the 'if else/if' block as it should.
If the first condition is false, it checks the next condition which is if the number is divisible by '5', if that is true it will break out of the 'if' block and log "fizz" out to the console... this goes for all cases of an "if, else/if" statement.
If you had a made a conditional check of '3' before you made a conditional check of '5', when the condition of '3' is true, it will print "buzz" and break out of the if else/if block and it will never check that last condition of '5'.
Order of execution is crucial as this is a procedural programming paradigm.

Jason Anello
Courses Plus Student 94,610 PointsHi Andrew,
What was wrong with Kevin's code? I don't see anything wrong with it.
Re: order of execution
The mod 15 check does need to go first but it doesn't matter which order you put the mod 3 and mod 5 check. The program should run slightly faster though if you put the mod 3 check before the mod 5 check.
The problem does require that you output "fizz" for multiples of 3 and you're having it output "buzz" instead. You have your "fizz" and "buzz" reversed.
anotherdude
7,053 Pointsanotherdude
7,053 PointsMay I post a follow-up question?
I tried to get it counting up from 1 to 100 ... (had to come here to understand the "mod 15" thingy)
What I wonder is: How to get the console.log-output started at 1, instead of 0?
Because even if I write
for (var counter =1; ...
... the console still starts with 0, putting out a "fizzbuzz" ...
Here's what I wrote:
... any hint is appreciated ;) Thanks
Thanks Jason - tried it again today & all works fine with
var counter = 1
... actually my comment is unnecessary then. can I just delete it?Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 Pointsanotherdude,
I tried your code but changed it to
var counter = 1
and it started at 1 as expected.