Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial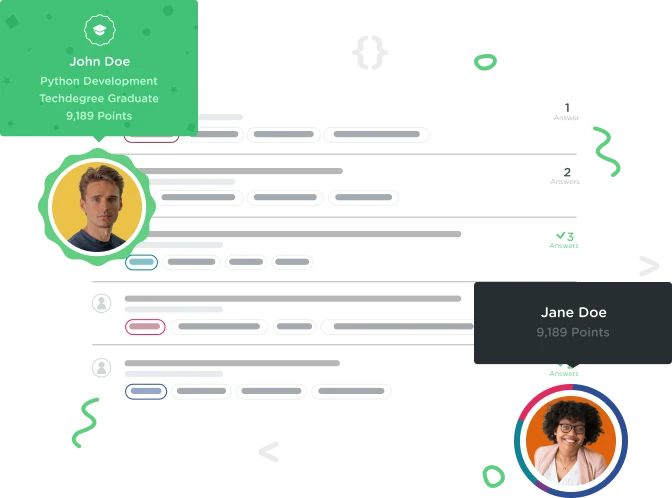

Jos Feseha
4,011 PointsFizzBuzz - I don't know what is wrong with this code , please help
I don't know what is the problem with this code ....
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
for n in 1...100{
if (n % 3 == 0) && (n % 5 == 0) {
return "FizzBuzz"
}
else if (n % 3 == 0) {
return "Fizz"
}
else (n % 5 == 0) {
return "Buzz"
}
else {
return (n)
}
}
// End code
return "\(n)"
}
1 Answer

robertrinca
Courses Plus Student 11,316 PointsWell, just looking at your code and not the challenge, there are a couple of issues:
On line 10, you are missing an "if".
And then on 14, the syntax is incorrect and the function needs to return a string, so that should be:
return "(n)"
That will get rid of the errors, but the function is flawed in that it is always stepping through the same numbers 1 through 100 no matter what value you pass into it.
So, your code fixed should look like this:
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
for n in 1...100{
if (n % 3 == 0) && (n % 5 == 0) {
return "FizzBuzz"
}
else if (n % 3 == 0) {
return "Fizz"
}
else if (n % 5 == 0) {
return "Buzz"
}
else {
return "\(n)"
}
}
// End code
return "\(n)"
}
But the function won't do anything except return 1 every time it's called, so I'd drop the for loop and the last else statement, and go with something like this:
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
if (n % 3 == 0) && (n % 5 == 0) {
return "FizzBuzz"
} else if (n % 3 == 0) {
return "Fizz"
} else if (n % 5 == 0) {
return "Buzz"
}
// End code
return "\(n)"
}
That function actually works and can be called like:
let result = fizzBuzz(n: 15)
and it actually functions.
Hope this helps.
Jos Feseha
4,011 PointsJos Feseha
4,011 Pointsohh yes definitely, it helped a lot and worked perfectly . Thank You So much !