Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial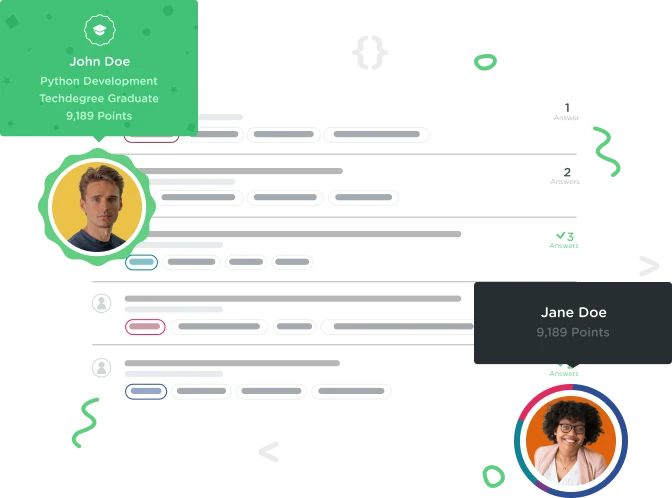

Alejandro Reyes
Courses Plus Student 1,927 PointsFizzBuzz in C
This is a normal FizzBuzz, if the number is divisible by 3 print "Fizz", if divisible by 5 print "Buzz" if divisible by both print "FizzBuzz", I think the code is fine but why is the output (lldb) and not the numbers? please help
include <stdio.h>
int main(int argc, const char * argv[]) {
int num;
for (num = 0; num <= 100; num++)
if (num % 3 == 0) {
printf("Fizz %d", num);
}
else if (num % 5 == 0) {
printf("Buzz %d", num);
}
else if (num % 3 == 0 && num % 5 == 0) {
printf("FizzBuzz %d", num);
}
else {
printf("%d", num);
}
return 0;
}
3 Answers
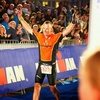
Steve Hunter
57,712 PointsThe statements are mutually exclusive. If the first test passes no others are run.
So, you're expecting, say, 15 to give you a "FizzBuzz" output. It won't. It'll hit the first test and be divisible by three and progress no further.
Move the joint condition to the beginning; make that the first test. Test for both, then test for the individual ones.
Understand? Make sense?
Steve
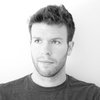
Matthew Rigdon
8,223 PointsFirst, I must say I am not that familiar with C. That being said, there does appear to be an error in the code logic. Right now, the code first checks for remainders if you divide by three. Remember, it is possible that a number is divisible by 3 and 5. There will be some scenarios, like the number 15, that will have your first line of code print "Fizz" before it even checks for "FizzBuzz." Else if code will only run if all the prior lines returned false.
This is how I would personally structure the code: (The code is not in C, but just written for general understanding)
for (int i = 1; i <=100; i++)
{
if (i % 3 = 0 and i % 5 = 0)
{ print('FizzBuzz')}
else if (i % 3 = 0)
{ print ('Fizz')}
else if (i % 5 = 0)
{ print ('Buzz')}
else
{ print (i)}
}
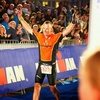
Steve Hunter
57,712 PointsAnd your For loop isn't bounded by curly braces. After num++) open a brace, {
Then before the return 0, close it }
Alejandro Reyes
Courses Plus Student 1,927 PointsAlejandro Reyes
Courses Plus Student 1,927 PointsThank you, I got it now, but the output was (lldb) what was that? a bug? and I had to do; if (num % 15 == 0) { printf("FizzBuzz") }, instead of;
if(num % 3 == 0) && (num % 5 == 0) { printf("FizzBuzz") }
I couldnt use the && operator on the last, it gave an unexpected identifier error on the second appearance of the word "num", why is this?
Thank you!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsThe lldb thing was just the code running. Don't worry; you saw it due to an error in your code.
I don't understand why you are testing
num % 15 == 0
- why have you added that test?And the error you are seeing may be because there is a semicolon missing after the
printf
statement.Matthew Rigdon
8,223 PointsMatthew Rigdon
8,223 Pointsnum % 15 == 0 is the same as testing for num % 3 == 0 && num % 5 == 0. It essentially is testing for "FizzBuzz." As the for the second appearance of "num:" when you wrote int num; at the beginning of your code, you established the num variable as a int that will eventually be given a number.
However, when you write a for-loop statement, the first part of your code ... for ( variable, condition, increase/decrease) actually is making a variable that is only used in the for loop. However, you already used num early in your code so it is throwing an error. You should delete the int num; at the beginning of the code since it is not needed.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsGood point; thank you.
Not sure about the
num
thing to be honest. The local variable in the for loop should work fine. Anyway, I've been staring at code & posts all day and need to stop! Work in the morning. Oh, the joy.Steve...