Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial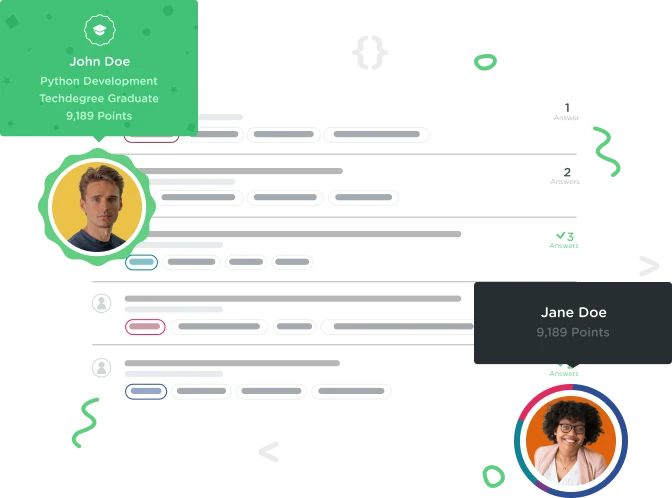
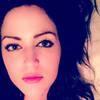
Nikki Bearman
3,389 PointsFizzBuzz solution - any idea what I've done wrong?
I've been stuck on the FizzBuzz challenge in the Swift 2.0 course. You are supposed to return "Fizz" for any number of a given range (in my case 1...100) when a number is divisible by 3, "Buzz" when a number is divisible by 5 and "FizzBuzz" when a number is divisible by both 3 and 5.
I've been stuck on this for quite some time as I can't work out where I've gone wrong and nothing I do seems to work in the challenge area, despite the code (minus the first and last lines which were pre-supplied) working fine for me in the Xcode playground and returning the expected results.
Can anyone help by giving me some idea of where I've gone wrong? I imagine it's something super obvious but I've gone over it so many times it's beginning to blur!
Thanks in advance for any help.
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
for n in 1...100 {
if (n % 3 == 0) && (n % 5 == 0) {
return "FizzBuzz"
} else if (n % 3 == 0) {
return "Fizz"
} else if (n % 5 == 0) {
return "Buzz"
} else {
return n
}
}
// End code
return "\(n)"
}
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Nikki,
You've added in 2 extra things which is throwing off the challenge tester.
The instructions mention not to worry about the default case of returning the number. You can take out your else statement because the starter code already has code at the end to return the number.
It also mentions not to use a loop. The number is going to be passed into this function and your code only needs to check that one particular number.
Removing those things you should have:
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
if (n % 3 == 0) && (n % 5 == 0) {
return "FizzBuzz"
} else if (n % 3 == 0) {
return "Fizz"
} else if (n % 5 == 0) {
return "Buzz"
}
// End code
return "\(n)"
}

Sam Chaudry
25,519 PointsHi Nikki
I checked your code through and seems to be working fine, it's the Treehouse compiler playing up. When checking for the "Buzz", check for the remainder of 5 not 7 - this code should help you pass the challenge:
func fizzBuzz(n: Int) -> String { // Enter your code between the two comment markers if (n % 3 == 0) && (n % 5 == 0) { return "FizzBuzz" } else if (n % 3 == 0) { return "Fizz" } else if (n % 5 == 0) { return "Buzz" } else { return "(n)" } // End code //return "(n)" }
Nikki Bearman
3,389 PointsNikki Bearman
3,389 PointsThanks so much Jason, I misunderstood the instructions a little but following your directions it finally let me pass! Thank you for your help.