Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial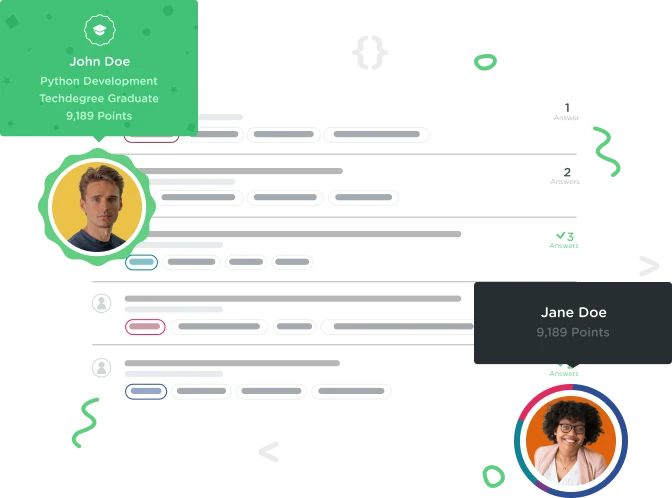

Chris McKay
2,401 PointsFizzBuzz Swift Challenge
Below is my solution for the FizzBuzz Challenge:
import UIKit
var number = 30
let fizz = number % 3
let buzz = number % 5
if fizz == 0 && buzz == 0 {
println("FizzBuzz")
} else if fizz == 0{
println("Fizz")
} else if buzz == 0 {
println("Buzz")
}
else {
println("Try Again!")
}
If I were to move the "FizzBuzz" check to the end rather than the beginning, it will never return as true. How would I force it to override the others without moving it to the top of the chain?
5 Answers

Daniel Mattingley
1,493 PointsHi Chris,
There isn't a way to make a subsequent "else if" statement take precedence over a preceding true "if" statement - the order of the "if" and "else if" is important.
If you really want to move the "FizzBuzz" to the end however, there are a couple of ways you can code this:
if fizz == 0 && buzz != 0 {
println("Fizz")
} else if buzz == 0 && fizz != 0 {
println("Buzz")
} else if fizz == 0 && buzz == 0 {
println("FizzBuzz")
} else {
println("Try Again!")
}
This is testing at each stage to check that the FizzBuzz condition is not met, before exiting the "if" loop.
Alternatively:
if fizz == 0 {
print("Fizz")
}
if buzz == 0 {
print("Buzz")
}
if fizz != 0 && buzz != 0 {
print("Try Again!")
}
println()
This means each "if" statement of the code is checked, regardless of whether the preceding condition is met or not. By using "print" rather than "println", Fizz and Buzz are printed next to each other in the console (i.e. as FizzBuzz) if both conditions are met. Then the println() function at the end means if you are testing a series of numbers, the results are all on different lines.
Hope that helps!
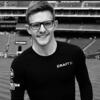
agreatdaytocode
24,757 PointsHere is one:
func fizzbuzz(i: Int) -> String {
let result = (i % 3, i % 5)
switch result {
case (0, _):
return "Fizz"
case (_, 0):
return "Buzz"
case (0, 0):
return "FizzBuzz"
default:
return String(i)
}
}
for number in 1...100 {
println(fizzbuzz(number))
}
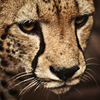
Will Macleod
Courses Plus Student 18,780 Pointsvar number = arc4random_uniform(1000000)
//var number = 8
var divThree = number % 3
var divFive = number % 5
if (divThree == 0) && (divFive == 0) {
println("FishBash")
} else if divFive == 0 {
println("Bash")
} else if divThree == 0 {
println("Fish")
} else {
println("Not Divisible")
}

Chris McKay
2,401 PointsHey Alex,
That is the same result as my code. My point was that if you change the order to :
if divFive == 0 {
println("Bash")
} else if divThree == 0 {
println("Fish")
} else if (divThree == 0) && (divFive == 0) {
println("FishBash")
} else {
println("Not Divisible")
}
FishBash would not work if var = 30. Hence the question: How would I write this to make sure that irrespective of the order, the && check would supersede the others.
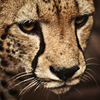
Will Macleod
Courses Plus Student 18,780 Pointsvar number = 30
var divThree = number % 3
var divFive = number % 5
if (divThree == 0) && (divFive == 0) {
println("FishBash")
} else if divFive == 0 {
println("Bash")
} else if divThree == 0 {
println("Fish")
} else {
println("Not Divisable")
}
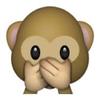
Philip Ondrejack
4,287 PointsAfter playing around with it for awhile, I stumbled on this...
for i in 1...100 {
switch i {
case _ where i % 3 == 0 && i % 5 == 0:
println("fizzbuzz")
case _ where i % 3 == 0:
println("fizz")
case _ where i % 5 == 0:
println("buzz")
default:
println(i)
}
}
Chris McKay
2,401 PointsChris McKay
2,401 PointsThanks Daniel! Your solutions make a lot of sense.