Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial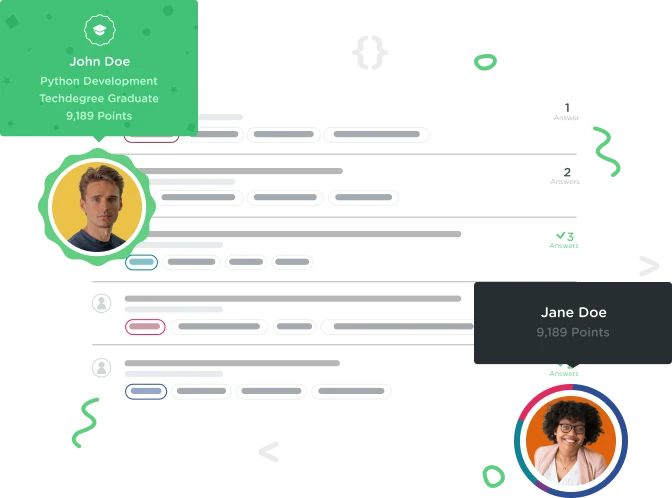
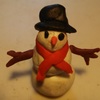
Joni Carlson
11,940 PointsFlask Basics Challenge Task 1 of 5
Can anyone find the error in this code? It does not pass the challenge and I have tired about 15 variations with no effect.
from flask import Flask
app = Flask(name)
@app.route('/multiply/<int:num1>/<int:num2>') @app.route('/multiply/<float:num1>/<float:num2>') @app.route('/multiply/<int:num1>/<float:num2>') @app.route('/multiply/<float:num1>/<int:num2>')
def multiply(num1, num2): #return 5 * 5 return '{} * {} = {}'.format(num1, num2, num1*num2) #return str(num1*num2) app.run(debug=True, port=8000, host='0.0.0.0')
from flask import Flask
app = Flask(__name__)
@app.route('/multiply/<int:num1>/<int:num2>')
@app.route('/multiply/<float:num1>/<float:num2>')
@app.route('/multiply/<int:num1>/<float:num2>')
@app.route('/multiply/<float:num1>/<int:num2>')
def multiply(num1, num2):
#return 5 * 5
return '{} * {} = {}'.format(num1, num2, num1*num2)
#return str(num1*num2)
app.run(debug=True, port=8000, host='0.0.0.0')
6 Answers
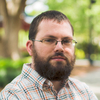
Kenneth Love
Treehouse Guest Teacher- You shouldn't have a blank line between your decorators and the function they decorate
- You no longer have a route for just
/multiply
. That shouldn't ever go away - You need to always return the product of the numbers, not the mathematical sentence
- IIRC,
num1
andnum2
should default to 5
If you're getting a timeout or it's just sitting there, that's because of the app.run()
at the bottom. I don't think I'm able to catch and remove those, but I'll look into it tomorrow.
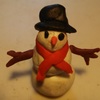
Joni Carlson
11,940 PointsI changed it to this, but it is still not running from flask import Flask
app = Flask(name)
@app.route('/multiply') @app.route('/multiply/<int:num1>/<int:num2>') @app.route('/multiply/<float:num1>/<float:num2>') @app.route('/multiply/<int:num1>/<float:num2>') @app.route('/multiply/<float:num1>/<int:num2>') def multiply(num1, num2): return str(num1*num2)
The error I am getting is Didn't return a 200 at /multiply
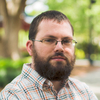
Kenneth Love
Treehouse Guest TeacherYou need default values for num1 and num2.
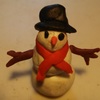
Joni Carlson
11,940 PointsHere is this iteration of my code from flask import Flask from flask import render_template
app = Flask(name)
@app.route('/multiply/') @app.route('/multiply/<int:num1>/<int:num2>') @app.route('/multiply/<float:num1>/<float:num2>') @app.route('/multiply/<int:num1>/<float:num2>') @app.route('/multiply/<float:num1>/<int:num2>') def multiply(num1=5, num2=5): return str(num1*num2)
I have tried also the following: def multiply(num1="5", num2="5"):
def multiply(num1=int("5"), num2=int('5"):
return num1*num2
Can you just send me the actual code? I am just too stuck.
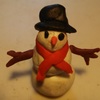
Joni Carlson
11,940 PointsHere is this iteration of my code from flask import Flask from flask import render_template
app = Flask(name)
@app.route('/multiply/') @app.route('/multiply/<int:num1>/<int:num2>') @app.route('/multiply/<float:num1>/<float:num2>') @app.route('/multiply/<int:num1>/<float:num2>') @app.route('/multiply/<float:num1>/<int:num2>') def multiply(num1=5, num2=5): return str(num1*num2)
I have tried also the following: def multiply(num1="5", num2="5"):
def multiply(num1=int("5"), num2=int('5"):
return num1*num2
Can you just send me the actual code? I am just too stuck.
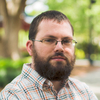
Kenneth Love
Treehouse Guest TeacherYou've almost got it. You have to return a string, so return str(num1*num2)
. And the defaults need to be ints, not strings like your last examples. I'm just on my phone right now but I can post better code answers tomorrow
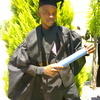
THAPELO MOKOKO
9,457 Pointsfrom flask import Flask
app = Flask(name)
@app.route("/") def hello(): return "Hello, World!"
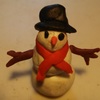
Joni Carlson
11,940 PointsTurns out that the extra / on the multiply route was the problem.
Joni Carlson
11,940 PointsJoni Carlson
11,940 PointsI tried with this lines added at various times: @app.route('/multiply') or without app.run(debug=True, port=8000, host-'0.0.0.0')