Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial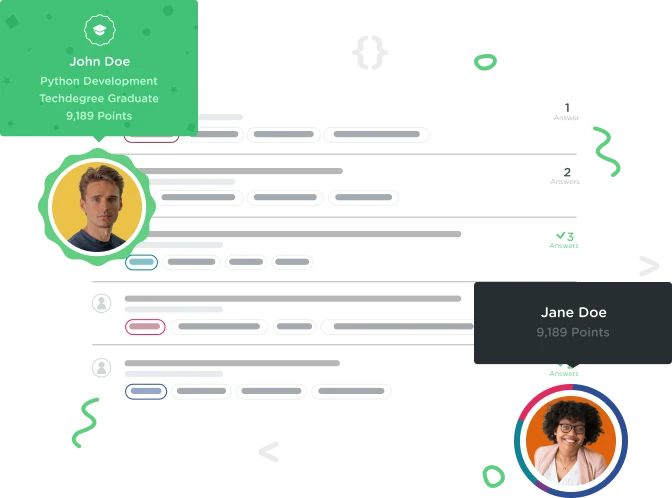

Johannes Scribante
19,175 PointsFlask macro hide_email code challenge. Syntax, structure error maybe?
Busy on the Flask Track and at this point we are adding macros to our templates.
The challenge you are required to make a macro called hide_email passing the macro a User which has an email attribute.
The macro is to hide the email so that, if given an email for example, test@example.com it should only show t***@example.com.
My code manages to give out k******@teamtreehouse.com
when given kenneth@teamtreehouse.com. But when I check my work I get Bummer got k******@teamtreehouse.com
.
Did I perhaps make a spelling mistake, syntax or structure error?
Btw, if you know of a better way to write please share, I would like to think there is a more effective way to write this code?
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
class User:
email = None
user = User()
user.email = 'kenneth@teamtreehouse.com'
return render_template('user.html', user=user)
{% macro hide_email(user) %}
{{ user.email.split('@')[0][0] }}
{% for letter in user.email.split('@')[0][1:] %}
{{ '*' }}
{% endfor %}
{{ '@' + user.email.split('@')[1] }}
{% endmacro %}
2 Answers

Johannes Scribante
19,175 PointsSo I managed to solve the problem :) Part of the learning process is reading the docs and finding alternate ways even though your current one seems to work.
So instead of trying to fit all of the logic into the macro.html
file and making the logic only usable in a single place in your code, you should look at ways to apply DRY (Don't Repeat Yourself). So the question is how to do that?
Well Flask in the docs (http://flask.pocoo.org/docs/0.12/templating/#registering-filters) allows you to build reusable filter to manipulate your data.
Therefore to be able to hide the email.
In app.py
@app.template_filter(hide_email_filter)
def hide_email_filter(email_string):
# add your logic here to hide the email e.g. t***@example.com
return hidden_email
In macro.html, this is how you would apply your filter to you data.
{% macro hide_email(user) %}
{{ user.email | hide_email_filter }}
{% endmacro %}

Bleza Takouda
5,129 PointsI came across the same issue. Thanks you for the hint.

Stephen Hutchinson
8,426 PointsTo ensure everything is on a single line with no line breaks:
{% macro hide_email(user) %}
{{ user.email.split('@')[0][0] }}{% for char in user.email.split('@')[0][1:] %}{{'*'}}{% endfor %}{{ '@' + user.email.split('@')[1] }}
{% endmacro %}
Not sure how to ensure that there are no spaces or breaks
Mackenzie Brennan
8,420 PointsMackenzie Brennan
8,420 PointsSo I also had this same problem. The reason you're getting an error is because jinja is adding whitespace before and after every block. You can prevent the whitespace by adding a dash on the side of the block that you don't what it. For example., {{- '' -}} would prevent whitespace on either side of the ''. After adding the dashes into each block my code was able to pass.