Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial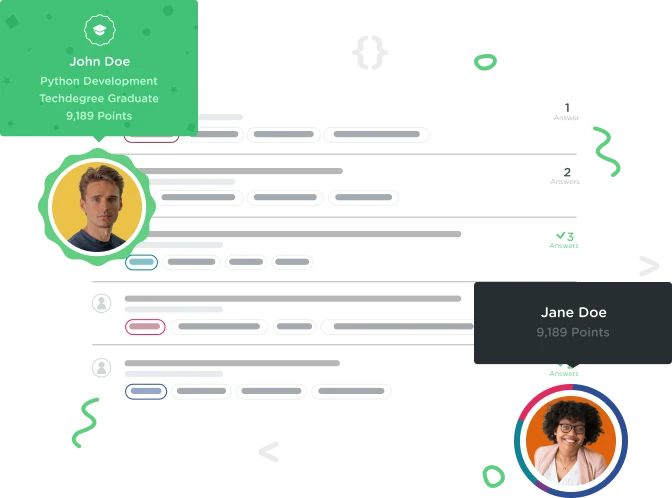

Josh Keenan
20,315 PointsFlask restful DB not creating tables
So I am working on a project for uni and using flask again, developing a restful API, and on my first trial run of it I keep getting the same error:
peewee.OperationalError: no such table: user
I am unsure why the table isn't being created but the Db is, been throwing get and post requests at it but getting internal server errors.
app.py
from flask import Flask
import models
from resources.users import users_api
app = Flask(__name__)
app.register_blueprint(users_api)
@app.route('/')
def hello_world():
return 'Hello World!'
if __name__ == '__main__':
models.initialize()
app.run(debug=True)
models.py
from peewee import *
DATABASE = SqliteDatabase('users.sqlite')
class User(Model):
username = CharField(unique=True)
email = CharField(unique=True)
class Meta:
database = DATABASE
def initialize():
DATABASE.connect()
DATABASE.create_tables([User], safe=True) # prevents creation of tables every time it's run
DATABASE.close()
/resources/users.py
import json
from flask import Blueprint, jsonify
from flask_restful import Resource, Api, reqparse, fields, marshal_with, marshal, inputs
import models
user_fields = {
'id': fields.Integer,
'username': fields.String,
'email': fields.String
}
class UserList(Resource):
def __init__(self):
self.reqparse = reqparse.RequestParser()
self.reqparse.add_argument(
'username',
required=True,
help='no username provided',
location=['form', 'json']
)
super().__init__()
def get(self):
users = [marshal(user, user_fields) for user in models.User.select()]
return {'Users': users}
def post(self):
args = self.reqparse.parse_args()
models.User.create(**args)
users = [marshal(user, user_fields) for user in models.User.select()]
return {'Users': users}
class User(Resource):
def get(self):
return jsonify({'user': 'email'})
def post(self):
return jsonify({'user': 'email'})
def put(self):
return jsonify({'user': 'email'})
def delete(self):
return jsonify({'user': 'email'})
users_api = Blueprint('resources.users', __name__)
api = Api(users_api)
api.add_resource(
UserList,
'/api/v1/users',
endpoint='users'
)
api.add_resource(
User,
'/api/v1/users/<int:id>',
endpoint='user'
)
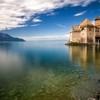
nakalkucing
12,964 PointsHi Josh Keenan! Do you mind posting a Snapshot of your workspace? It would make debugging easier. Thx. :)
Josh Keenan
20,315 PointsJosh Keenan
20,315 PointsChris Freeman