Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial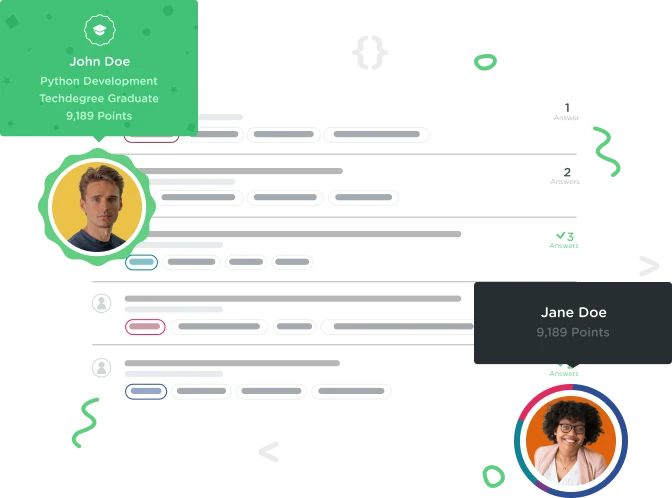
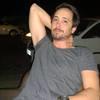
Russell Kree
1,956 PointsFlat out lost
I totally understand the for loop concept and how using a double value to hold each value of the array added to the value (+=) then divide that by the length of the array (frog.Length) but I just cant code it. I keep getting a barrage of errors.
Cn someone paste their correct code in the answer please.
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
for(int i = 0; i < frogs.Length; i++)
{
Frog frog = frogs[i];
double sum += frog;
double average = sum / frogs.Length;
}
return average;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
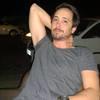
Russell Kree
1,956 PointsHere is my code.
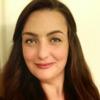
Jennifer Nordell
Treehouse TeacherRussell Kree I'm with Steven Parker on this one. I'd like to see what you're trying that's failing before I just post an answer. But here's one thing I notice. You state this: "... double value to hold each value of the array ...". Except the values of that array are integers as specified by TongueLength in the Frog class. But double is going to be what is returned
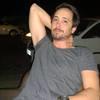
Russell Kree
1,956 PointsThe code i input was:
for(int i = 0; i < frogs.Length; i++) { Frog frog = frogs[i]; double sum += frog; double average = sum / frogs.Length; } return average; }
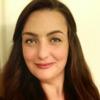
Jennifer Nordell
Treehouse TeacherRussell Kree Yes, I must've been posting my comment as you posted your code above.
6 Answers
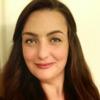
Jennifer Nordell
Treehouse TeacherOk then, here we go!
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double totalLength = 0.0;
for(var i = 0; i < frogs.Length; i++) {
totalLength += frogs[i].TongueLength;
}
return totalLength / frogs.Length;
}
}
}
So this function takes an array of frogs (which sounds weird I know, but still true). And it returns a double. I started by declaring a variable to hold the total length of all the frogs' tongues. I set this to 0.0 and made it a double. Then I set up my for loop. I begin with the i = 0 and count up until the end of the frog array. Every iteration I get the length of the tongue of the frog at that index. And I take that and add it to totalLength. This gives a running total of tongueLength. When the for loop has reached its end I take the totalLength and divide it by the number of frogs. This will give us an average tongue length for all the frogs passed in. This is the value I return. Hope this helps to clarify things!
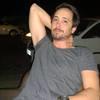
Russell Kree
1,956 PointsAh ok I understand. Thanks so much hey! Thing that was throwing me off is that generally when you create on instance you declare it right? I didnt understand the syntax in parameters being the declaration.
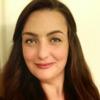
Jennifer Nordell
Treehouse TeacherRussell Kree Generally speaking, you're correct. Except that you can sort of think of the parameter that the function takes as simultaneously being a variable declaration. Now here they used frogs as an array of Frog. But we really could have named that array anything. We could have named it myFrogs, or yourFrogs, or sillyFrogs as long as the Frog[] is there. What we'll have is an array of type Frog coming into our function. Then we can use that variable the same way we would any other.
But here's where we get to scope. Once that function has been run and exits, our array of frogs (no matter what it was named) disappears and we no longer have access to it. Which is why, most times, we have some sort of return statement. Quite often we want to get back some calculation from our function. There are times when a function won't return anything, but generally it's when we have some sort of printing out to do (at least in my experience).
Hope that helps
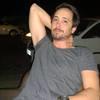
Russell Kree
1,956 PointsThanks a lot for the help hey.
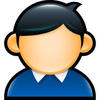
Daniel Hildreth
16,170 PointsJennifer Nordell I know I've used the += operands a few times before. I know that it is adding in it. However, I never seem to remember the long way of doing it to re-explain that method of short hand operands. In the example you used from the code challenge, is it saying "totalLength = totalLength + frogs[i].TongueLength?" I always seem to forget how these operands work, even though I semi understand the concept of them (if that makes any sense).
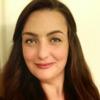
Jennifer Nordell
Treehouse TeacherDaniel Hildreth Yes. That's exactly correct. Here might be a simpler example just to reinforce how it works.
int y = 5;
y = y + 2;
y += 2;
So y starts out as 5. On the first line we add 2 to y and then assign the result back into y. y
is now equal to 7. The second line does the same thing. It takes the value of y, adds 2 to it, and puts the result back into y. At this point our y value would be 9. The +=
is just shorthand. There's nothing to say you have to use it. Hope this clears things up!

Habib Miranda
7,320 PointsThis may b a silly question, but where did the .TongueLength come from on the line inside the loop body? Everything else makes sense, but I can't figure that little bit out!
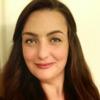
Jennifer Nordell
Treehouse TeacherHabib Miranda Hi there! TongueLength is a property defined in the Frog class. And we're looking at an array of frogs of type Frog. So each frog has a property named TongueLength
. Take another look at the Frog class
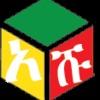
Ashenafi Ashebo
15,021 PointsThanks, Jennifer!
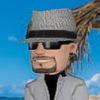
Thomas Beaudry
29,084 PointsHi Jennifer,
You are an awesome coder, how did you get so good??? I've noticed you seem to know just about every language there is, so what is your secret???

Emma Wyman
13,250 PointsThank you! This question was so vague, I didn't even know where to begin.
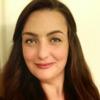
Jennifer Nordell
Treehouse TeacherHere's your hint #1. It's generally speaking a bad idea to declare a variable inside a loop. Hint #2. You're trying to make a new frog every time through your loop and setting the frog to an integer. Again, the values stored in that array are TonugeLength s (which are integers).
See if you can make some adjustments and get the ball rolling a bit towards a solution
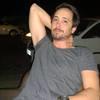
Russell Kree
1,956 PointsStill lost.
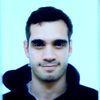
Filipe Conceição
4,090 Points"double totalLength = 0.0;"
you can replace this with:
var totalLength = 0.0;
it will be double cause you're declaring it with 0.0.
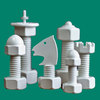
Steven Parker
241,783 PointsMy opinion about variables in loops is that it's fine if the variable is used only inside the loop. But anything used outside the loop must be declared outside the loop (or it won't even compile).
I might offer these additional hints:
- never use a compound assigment (like +=) on anything until it has been given a starting value
- be sure you are summing the TongueLength properties of the frogs
- concentrate on creating a sum inside the loop, then convert it to an average when the loop ends
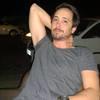
Russell Kree
1,956 PointsStill lost.

Michael Thompson
7,427 PointsI just got done with this challenge and basically had to copy and paste 90% of the answer as this objective made basically no sense to me. Where did frogs.Length come from? How was I supposed to know how to get the average tongue length? I have NO idea how that for loop equates to adding up the tongue lengths in an array of tongue lengths and then gives the average. Can anybody break this down? I am getting lost and frustrated at how incredibly fast paced this is going and how I'm just being thrown all these terms at lightening speed. I don't get why some words have _ before them. Where frogs.Length came from. What is the purpose of one word being followed by .Another word(frogs.Length for example). So much information just being "taught" at such a willy nilly breakneck speed that concepts are going by without even realizing they were addressed in the first place.
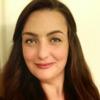
Jennifer Nordell
Treehouse TeacherHi there, Michael! Yes, I have seen quite a few questions about this particular challenge. The frogs.Length
came in when we were passed an array of frogs. Every array has a property called Length
. This was covered at around 5:03 of this video.
In the for loop, I start with with the total length being 0 because I haven't yet looked at any frogs. Every time through the loop, I take out a frog, measure its tongue and add it to the total length. The averaging part doesn't come until after I've gotten all the tongue lengths of all the frogs. I also could have written the addition line inside the for
loop like this:
totalLength = totalLength + frogs[i].TongueLength;
Both lines do exactly the same thing. They take the current value of totalLength
and add the current frog's tongue length to it and then assign it back into totalLength
. Getting the average is a mathematical calculation that you probably learned long ago. It is simply a sum of numbers divided by the total numbers present. For instances, if we have 5 frogs, then we would get their total length and divide by 5. This will give us the average tongue length.
So the next line, I take the total length of all the frogs and divide it by the number of frogs.
As for the underscore, when you see it, it's generally meant to denote a private field. This used to be the convention. It is now a topic of debate. That being said, Microsoft discourages the use of underscores in naming conventions. You can find documentation on that here. But this is still something that you might come across if you go work for a company where they really want you to use that naming convention. The underscore isn't all that important, but it's supposed to be a visual indicator that this is a private field. The problem is that many people have problems seeing the underscore.
As for the one word following another, just as Frog
has a property of TongueLength
, because we made it ourselves, the built-in C# object for Array has a property called Length
. The Length
property of an array holds an integer that represents the number of elements in the array.
Here is another explanation to the same problem that I posted elsewhere if that helps.
I feel like maybe you'd do well to go back and review courses you've already looked at before. I do! The thing about programming is that it's cumulative. You have to take all the tiny parts you've learned so that you can put them together to solve a puzzle and build something great. Reviewing material is highly encouraged. We all get lost. We all get stuck. I wrote something on this a while ago that might interest you. Take a look at this blog post.
I'd be happy to answer any more questions you have, if I can.
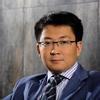
Andrei Li
34,486 PointsThis is my code. Seems to be working and correct. Treehouse should say that this is a game we don't know the input info. This part is hidden from the user. We have to play and make a correct code!
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double total = 0;
for(int i=0; i<frogs.Length; i++)
{
total += frogs[i].TongueLength;
}
return total/frogs.Length;
}
}
}
Steven Parker
241,783 PointsSteven Parker
241,783 PointsHow about making a good-faith attempt, sharing that code, and we help you fix it? That would provide a much better learning opportunity for you.