Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial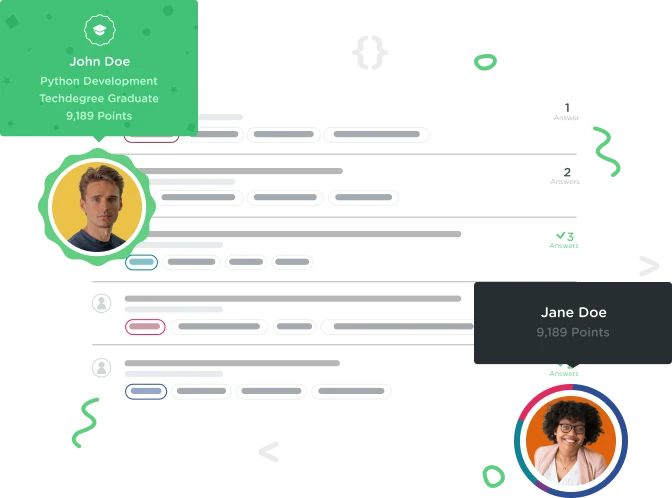

Joel Price
13,711 PointsFlies no longer move nor respond to collision.
Just as the title says, my flies no longer fly around or get destroyed when my frog runs into them. I can't figure out what's going on for the life of me. Any help is appreciated!
FlySpawner code
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FlySpawner : MonoBehaviour {
[SerializeField]
private GameObject flyPrefab;
[SerializeField]
private int totalFlyMinimum = 12;
private float spawnArea = 25f;
public static int totalFlies;
// Use this for initialization
void Start () {
totalFlies = 0;
}
// Update is called once per frame
void Update () {
//While the total number of flies is < the minimum...
while (totalFlies < totalFlyMinimum) {
//...then increment the total number of flies
totalFlies++;
//...create a random position for a fly
float positionX = Random.Range(-spawnArea, spawnArea);
float positionZ = Random.Range(-spawnArea, spawnArea);
Vector3 flyPosition = new Vector3 (positionX, 2f, positionZ);
//...and create a new fly.
Instantiate(flyPrefab, flyPosition, Quaternion.identity);
}
}
}
2 Answers

Mike Wagner
23,559 PointsI'm not sure, but is there other code that controls the fly movement? If not, then the issue here is that you are not re-assigning the transform of the fly to equal the updated flyPosition
value.
As for the destroying of flies, I can't remember if the fly itself or the frog was responsible for this action. If the flies are intended to handle their own destruction, then you have no code showing here that supports that functionality. You would need an OnTriggerEnter() call similar to this
void OnTriggerEnter(Collider other) {
Destroy(other.gameObject);
}
from the Unity Docs. You will need to check the collider of the object it collides with to see if it is the Player, and instead of destroying the player, you would destroy the fly, but I'll give you a chance to figure it out. I'll help more if you still need it.

Joel Price
13,711 Pointsmwagner - I'm pretty sure I've got all of those things set up, however after stepping away from it for a bit and coming back to it, now for whatever reason, the original fly I had before writing the FlySpawner script is working perfectly, but the spawned flies still have no collision reaction or flying around animation.

Mike Wagner
23,559 PointsI would make sure that there aren't any of the original fly in the scene, then put one of the prefab flies into the scene, making sure it has all the scripts it needs on it. Then I would make sure that the prefab is assigned to the game manager, making sure to apply any changes as necessary. It sounds like you might have assigned the original fly to the game manager instead of the prefab, or like you added the script to the original fly (or possibly to one of the in scene prefabs without applying the changes across all prefabs). i can't exactly see your project but that's my guess.
Joel Price
13,711 PointsJoel Price
13,711 Pointsyeah, the code for FlyMovement
but it was working before I added the code I linked in my post.
Mike Wagner
23,559 PointsMike Wagner
23,559 PointsJoel Price - There's nothing wrong with your code that I can see. I didn't copy it into my own version of this project, but it appears to be identical after a couple read-throughs and mine still functions as expected. Did you remember to assign the flyPrefab in the inspector? Is the FlySpawner script attached to the GameManager? Is the GameManager in the scene?