Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial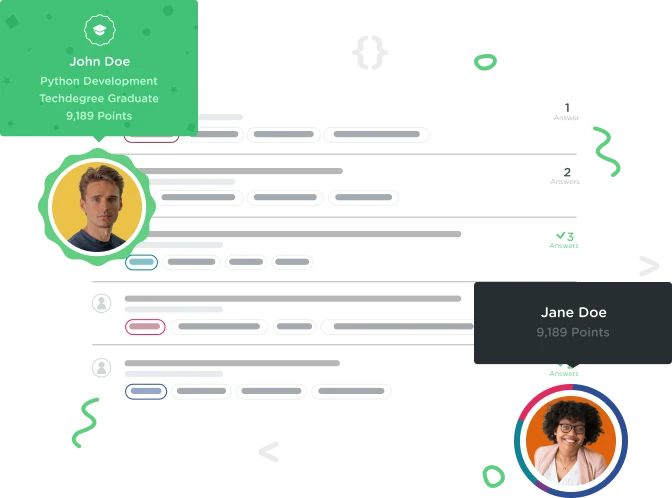

Paul Dunahoo
5,390 PointsFollowup question to "Conditionals - Switch"
The Conditionals - Switch video gives a better way of having multiple 'else if'-like statements. However, it still seems a little redundant.
This is the code in the video:
int main()
{
char a = 'a';
char b = 'b';
char g = 'g';
char letter = 'z';
switch (letter) {
case 'a':
printf("letter %c is %c\n", letter, a);
break;
case 'b':
printf("letter %c is %c\n", letter, b);
break;
case 'g':
printf("letter %c is %c\n", letter, g);
break;
default:
printf("letter %c not found\n", letter);
break;
}
return 0;
}
But is there a way to do something more like this (a combination of arrays and switch):
int main()
{
char a = 'a';
char b = 'b';
char g = 'g';
int randomize letter {'a', 'b', 'g'}
char letter = 'z';
switch (letter) {
case randomLetter:
printf("letter %c is %c\n", letter, randomLetter);
break;
default:
printf("letter %c not found\n", letter);
break;
}
return 0;
}
Call me silly, but this would make sense.
P.S. I know that my "randomize" method isn't proper or properly written, but I think you can get the idea of what I'm talking about.
1 Answer
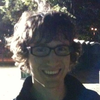
Ben Rubin
Courses Plus Student 14,658 PointsEach case in a switch
statement needs to be a single value. You can't use an array as the condition, nor can you do something like
switch (value)
{
case value > 10:
// this doesn't work
break;
default:
// blah blah
break;
}
If you want to do what you're suggesting, an if
` statement is what you're looking for.
However, you CAN do something like this:
switch (letter)
{
case 'a':
case 'b':
case 'g':
printf("letter is either a, b, or g.");
break;
default:
printf("letter is not a, b, or g");
break;
}
The reason the above code works is that once you hit a matching case, your program will continue to execute everything within the switch
until it hits a break
command. That can cause problems if you forget to put in a break, but you can also use that to your advantage like this.
Paul Dunahoo
5,390 PointsPaul Dunahoo
5,390 PointsThanks!