Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial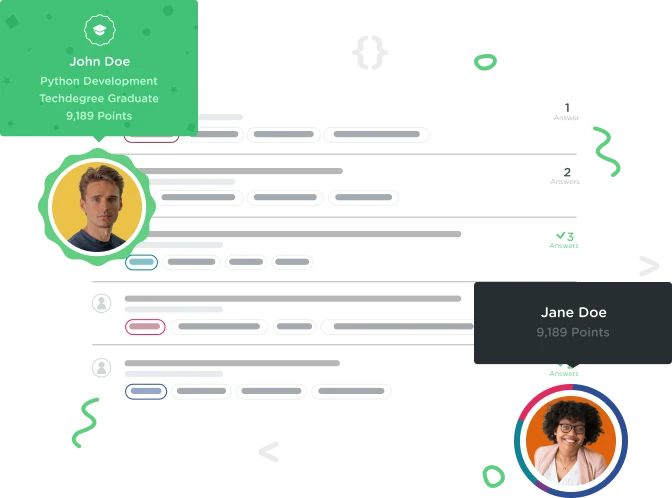

Dennis C
5,010 PointsFooter doesn't get selected for some reason
When I'm running my code and trying to console.log the footer, it's giving me 'this is footer: null', although I think I'm selecting it correctly. Also I don't quite understand where the text in request.responseText is coming from (it seems to be just an empty request).
var request = new XMLHttpRequest();
console.log("This is request: ", request);
const footer = document.getElementById('footer');
console.log('this is footer:', footer);
request.onreadystatechange = function () {
if (request.readyState === 4) {
document.getElementById("footer").innerHTML = request.responseText;
}
};
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with JavaScript</title>
<script src="app.js"></script>
</head>
<body>
<div id="main">
<h1>AJAX!</h1>
</div>
<div id="footer"></div>
</body>
</html>
5 Answers
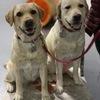
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Dennis,
To start, let's remember the four steps of creating an AJAX request:
-
Create the XMLHttpRequest object:
var request = new XMLHttpRequest();
-
Define the callback function (this is the function that the request object will call when it has finished the request):
request.onreadystatechange = function () { if (request.readyState === 4) { document.getElementById("footer").innerHTML = request.responseText; } };
Open a request (where you define what you want the request to do)—this is what you are being asked to do for part 1 of the challenge
Send the request.
At the time you ask for the value of footer, it is null: your JavaScript loads before the rest of the HTML loads, so at the time your JavaScript asks for the footer, there is no element with the ID of footer. You can prove this to yourself by moving your script tag to the bottom of the body, where it won't load until the text of the page has loaded. If you do that, the JavaScript will find your footer.
As for the text in request.responseText
, right now it's not coming from anywhere because you are not sending the request, and if there's no request, there can be no response.
When you do open the request, that will define what will be responded: in this case you are going ask to GET
the contents of footer.html
, thus the responseText
will be the text of footer.html
.
But remember, opening the request will just define what the request looks like, to actually initiate the request you'll need to send it (there's a second part to the challenge, so you might do that there...)
Cheers,
Alex

Dennis C
5,010 PointsHey Alex. Sorry for the late response. Great answer. Thank you very much. Makes much more sense now. I corrected the code, put the script before the closing body tag, and it makes more sense, but I've ran into another problem: Cross-Origin Request Blocked: The Same Origin Policy disallows reading the remote resource at https://api.segment.io/v1/i. (Reason: CORS request did not succeed), so the request never gets the readyState = 4. Here is my code: ```var request = new XMLHttpRequest(); console.log("This is request: ", request); const footer = document.getElementById('footer'); console.log('this is footer:', footer); request.onreadystatechange = function () { if (request.readyState === 4) { console.log('readyState = 4'); document.getElementById("footer").innerHTML = request.responseText; request.open("GET", "footer.html");
} };```

Dennis C
5,010 Pointsvar request = new XMLHttpRequest();
console.log("This is request: ", request);
const footer = document.getElementById('footer');
console.log('this is footer:', footer);
request.onreadystatechange = function () {
if (request.readyState === 4) {
console.log('readyState = 4');
document.getElementById("footer").innerHTML = request.responseText;
request.open("GET", "footer.html");
} };
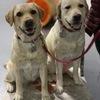
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Dennis,
Firstly, you don't need to make any changes to the HTML file, you're just being asked to add something to the JavaScript file. I only mentioned the location of the script tags as the reason why your console.log
statements were outputting null
(remember that you don't need any console.log
statements to pass this challenge either) and that you could prove this fact to yourself by seeing what happened with the script at the bottom of the body.
The problem with your script now is that while the syntax of your request.open
is correct, you've put it in the wrong place.
Remember that the request.onreadystatechange
is the callback function, that is, it is the function that will be executed once the request has completed in order to 'call back' to you with the response.
As such, it doesn't make sense to put the request.open
inside that method, this line is telling the request where to find the data you want, so the request needs this information before it does anything with the callback method.
Try moving the request.open
line out of the method and down to the bottom of your script.
Hope that helps
Cheers
Alex

Dennis C
5,010 PointsGot it. Now it works. Thank you very much for your help.