Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial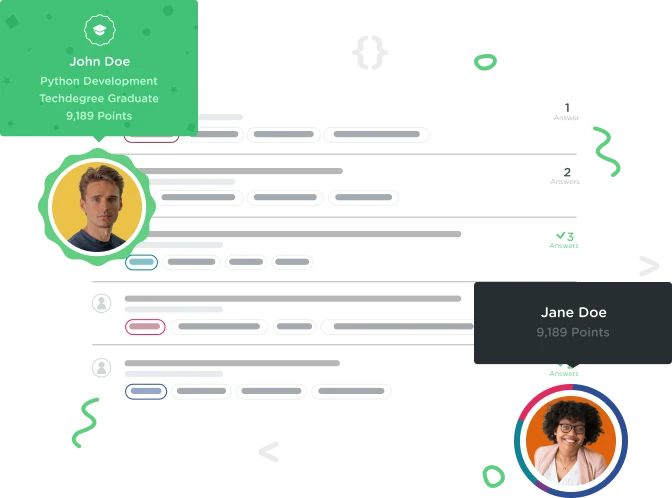

Ezekiel dela Peña
6,231 PointsFor Clarification (don't look here if you haven't answered the last quiz for this class)
<?php
class Fish {
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record) {
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
return "A $this->common_name is an $this->flavor flavored fish. The world record weight is $this->record_weight";
}
}
$bass = new Fish("Largemouth Bass", "Excellent", "22 pounds 5 ounces");
?>
so basically in using constructor for creating an object
it will get its value from (sort of getter)
function __construct($name, $flavor, $record) {
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
then throw the value to
public $common_name;
public $flavor;
public $record_weight;
Am I understanding this correctly? thanks in advance.
1 Answer
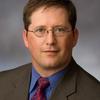
Ted Sumner
Courses Plus Student 17,967 PointsI am not sure you really understand, so I will give you this explanation. I am only using $name for simplicity.
Each time you call the class you have a new instance of the class. What is neat about it, though is that you can have multiple instances of the same class at the same time. The constructor assigns a value for each argument and keeps track of them for the individual instances. Thus you reduce the amount of code you need dramatically. Using the challenge code:
<?php
$carp = new Fish ('carp');
$shark = new Fish ("shark");
$tuna = new Fish ('tuna');
echo $tuna->getInfo() . "<br>";
echo $carp->getInfo() . "<br>";
echo $shark->getInfo();
// displays
// tuna
// carp
// shark
The program kept track of each variable based on the instance. The constructor assigns the value of the variable to the method based on what is passed to the class.
Ezekiel dela Peña
6,231 PointsEzekiel dela Peña
6,231 PointsI think I got it thanks for the explaination.