Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial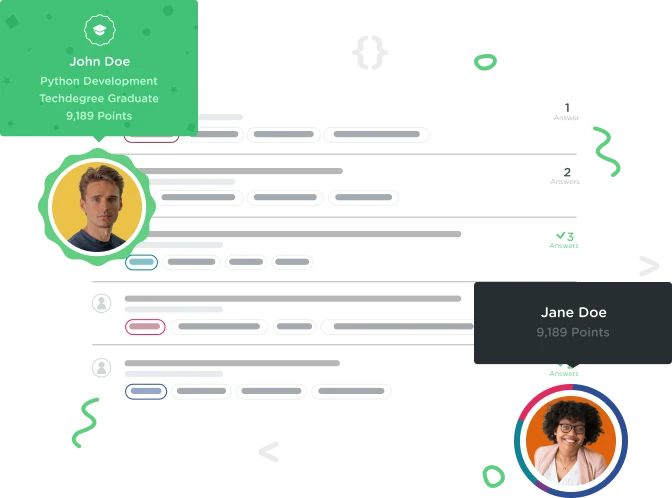

AR Aljanabi
Front End Web Development Techdegree Student 10,794 PointsFor Each Loop challenge code question
I stuck on the challenge code of for each loop in java..... So please can u explain to me what should i do
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(){
int counter = 0;
for (char tile: mHand.toCharArray()){
if (mHand.indexOf(tile) >= 0){
counter++;
}
}
return counter;
}
}
1 Answer

Rafael Cortes
1,174 PointsI noticed right off the top that you forgot to pass the tile to the method. So your first line should look like this.
public int getTileCount(char tile) {
After that everything else is written correctly as far as Java is concerned. Yet if memory serves me correctly about this challenge. You were asked to find a certain tile an number of times. So here is how to do that.
public int getTileCount(){
int counter = 0;
for (char tile: mHand.toCharArray()){
if (mHand.indexOf(tile) >= 0){
counter++;
}
}
return counter;
}
Like I said everything is correct except for one thing. The condition in the for loop's if statement. You already created an Array for mhand and the condition that you have is stepping through the Array created. By using the indexOf() method you are forcing the if statement to step through the Array. The "counter++" is counting each index of the Array because that tile could be in more then one place.
Here is what your condition is doing. When you called mHand.indexOf(tile) you are taking one tile and comparing to all the other tiles. But you called mHand. So that is like taking the same array and copying it to compare it. So it is like a feedback loop returning just the array. It works but it does nothing.
Here is the answer.
First: make sure that you are passing a character to the method. ie: go back to the top of this post to see what I am talking about. Next: Remember that the task was asking you to find a tile in the hand. So therefor we have to COMPARE the tile we pass to the tiles. Here is my rewrite of the first line in the for loop.
for (char tiles : mHand.toCharArray()) {
What I did here was create an Array called "tiles", why because an Array is a group of something and using name that are plural make sense. Now I can take the "tile" that I passed in and compare it to the the "tiles" Array. Here is what the code should look like.
if (tile == tiles) {
counter++;
}
Here is the complete code for you to review. Look at it closely and you can start to see how that program is working.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char tile){
int counter = 0;
for (char tiles: mHand.toCharArray()){
if (tile == tiles){
counter++;
}
}
return counter;
}
}
Here is what is happening in the code. When the for loop runs it creates the array. Then the if statement compares the tile with the first index of the array. It if is true then the counter increments. If false, no increments. But we are still in the for loop which has a limited number of indexes. So what the if statement is doing is that it is comparing the tile that was passed with each index. Each time it finds a matches, it increments the counter.
I hope this long winded post explains how for each loops work and help you move further along with your studies.

Michelle Bramlett
1,253 PointsThank you for your thorough explanation. Very Helpful!
Rylan Hilman
3,618 PointsRylan Hilman
3,618 PointsThe first error the compiler returns with this is "Bummer! Did you forget to create the method getTileCount that accepts a char?". So the purpose of the new method is to take a char and check the String to see how many of that specific char exist. So we change it to
public int getTileCount( char targetTile )
Second, the purpose of the for-each loop is to take one piece at a time of the array, work on that one piece, and do the same for every piece in the array in sequence. What you have there inside the if() statement is still checking the entire array by using indexOf on the String, not the single char that the for-each loop is taking out. To access the single item, it's what's on the left side of the colon, so the "char tile". And when comparing a char and a char, unless it's case-sensitive or something like that, a simple equality can suffice.
if ( targetTile == tile )