Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial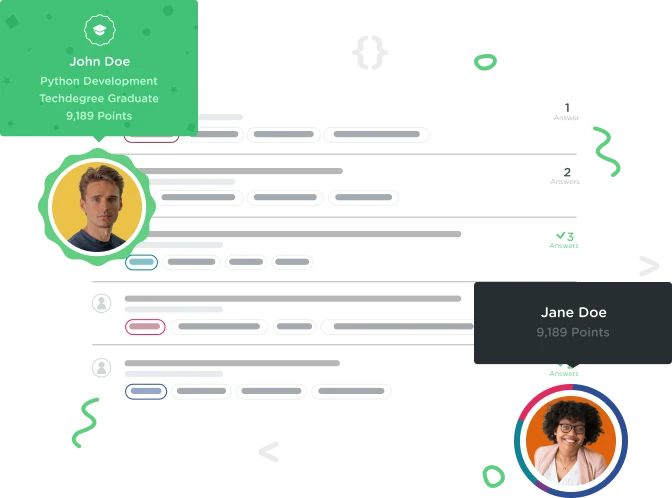
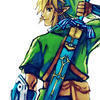
Dhruv Patel
8,287 PointsFor each/for in?
Just like Java has its for...each loop and Python has its for...in loop does JavaScript have its own equivalent to both or are we restricted to using the for(var i = 0;.....) instead... I believe JavaScript did have something known as a for each....in loop before but that too, no longer exists. What would us as JavaScript developers have to use instead?
3 Answers
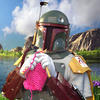
Lindsay Sauer
12,029 PointsJavaScript has for...in:
var person = {fname:"John", lname:"Doe", age:25};
for (var x in person) {
console.log(person[x]);
}
Edit: And if you're using the jQuery library, you can do something like:
$.each( person, function( key, value ) {
console.log( key + ": " + value );
});
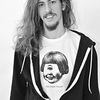
eck
43,038 PointsUsing for(var x in y){}
, as mentioned by Lindsay, is probably the best way to loop through an object without using a 3rd party library like jQuery.
There currently is another way with native JS to accomplish the same task though, using Object.keys:
var obj = { a: 1, b: 2, c: 3 };
Object.keys(obj).forEach(function(key){
console.log(obj[key]);
});
I am also aware of an experimental method, Object.entries, which can turn an object into an array. At the time of writing this it is not yet supported in browsers though.
If you do decide you want to use a library to loop through an object I recommend taking a look at lodash, which is a very helpful library that provides many functional methods and utilities.
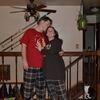
Julie Myers
7,627 PointsJavaScript comes with a variety of loops. Below are the three most common loops and basically what they are for.
/*
For Loop:
In JavaScript a for loop is best used for repeating task(s) and looping through
an array.
*/
var myArray = ["a", "b", "c", "d"];
for(var i = 0; i < myArray.length; i++) {
console.log(i);
}
/*
For...In Loop
A for...in loop is used to iterate through an object.
*/
var agents = {
'005': "Michael Harp",
'006': "John Smith",
'007': "James Bond"
};
for (key in agents) {
if ('007' === key) {
console.log('Bond, ' + agents[key] + ' has been found!');
} else {
console.log('Standard spy, ' + agents[key] + ' has been found');
}
}
/*
Array.prototype.forEach();
The forEach() method executes a provided function once per array element.
*/
// Create an array.
var numbers = [10, 11, 12];
// Call the addNumber callback function for each array element.
var sum = 0;
numbers.forEach(function addNumber(value) {
sum += value;
}
);
document.write(sum);
// Output: 33
For more info on loops go to http://developer.mozilla.org