Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial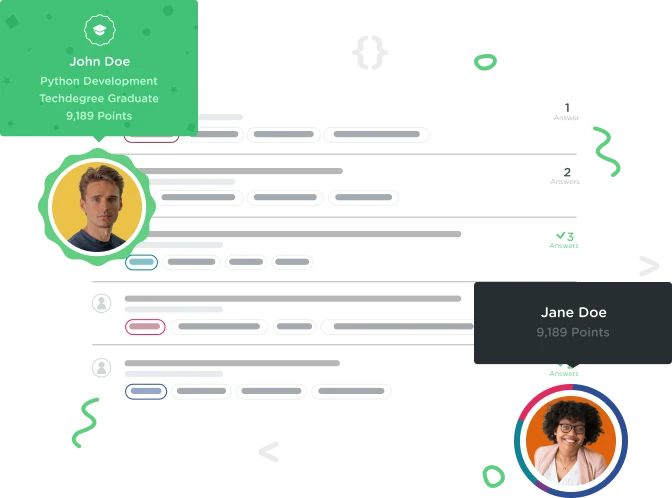
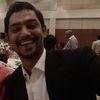
nafiz hassan
11,482 PointsFor example, with the string "Treehouse", sillycase would return "treeHOUSE".
For example, with the string "Treehouse", sillycase would return "treeHOUSE". Don't worry about rounding your halves, but remember that indexes should be integers. You'll want to use the int() function or integer division, //.
def sillycase(i):
half = len(i)//2
for x in i:
o = str(i[x]).lower()
i[x] = o
count = 0
count += 1
if count <= half:
break
for half in i:
o = str(i[x]).lower()
i[x] = o
return i
4 Answers
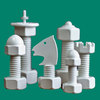
Steven Parker
230,274 PointsYou're working way to hard.
This challenge is intended to give you practice with slices, even though you can pass other ways. But if you use them, you can solve this in just a few lines (and no loops).
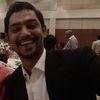
nafiz hassan
11,482 PointsIt's a learning process steven. If you could pull me back a little and enlighten me would be very nice of you coz I seem to be a little lost here.
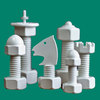
Steven Parker
230,274 PointsI added some extra hints in a comment on my previous answer ... see if those help.
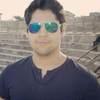
Jonathan Prada
7,039 Pointsshorter and chained
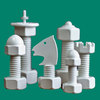
Steven Parker
230,274 PointsI"m not sure what you mean by this. The shorter solution doesn't involve "chaining" anything.
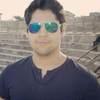
Jonathan Prada
7,039 Pointsdef sillycase(s): half = len(s)//2 return "{}{}".format(s[:half].lower(), s[half:].upper())
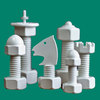
Steven Parker
230,274 PointsThat's one way to do it. But you can make it more concise by not using the format.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsSo for example, let's say you wanted just the first half of a string named "i". You've already computed "half" the length, so now you could apply a slice: "
i[:half]
". Then if you wanted, you could apply a case-changing function to that. You could also concatenate that whole thing to another slice (perhaps with the arguments arranged to return a different part of the string) with another case-changing function.Then if you were to return that entire expression, you'd have everything you need for the function to do in just two lines of code.