Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial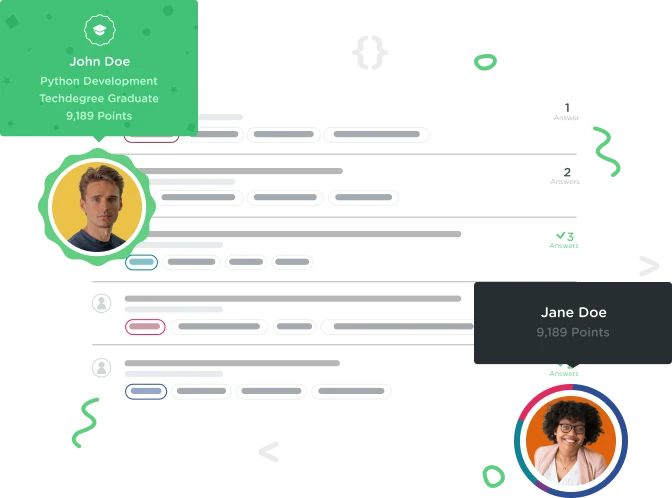

Sammy Elvis
214 PointsFor in Loop
Can we also use for in loop to access the properties and solve the question in this video?
3 Answers
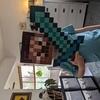
Gabbie Metheny
33,778 PointsI don't believe you can use for...in to iterate over an array of objects, like you have in this challenge, but you can use for...of:
for (let student of students) {
console.log(student.name);
}
// output:
// Dave
// Jody
// Jordan
// John
// Trish
or forEach():
students.forEach(student => console.log(student.name));
// output:
// Dave
// Jody
// Jordan
// John
// Trish
You'll have to play around to make this course more ES6-friendly, but it's good practice! Let me know if you try it!

Kevin Ohlsson
4,559 Pointsthis does not wrap in div and fails to capture name and set to h1 tag
for (let i = 0; i < 5; i++) {
let stuObj = (students[i])
console.log(stuObj.name)
for (let prop in stuObj) {
if (stuObj.name) {
document.write((`<h1> ${prop} : ${stuObj[prop]} </h1>`)) //catch name and wrap in h1 tag, fails
}
document.write((`<p>${prop} : ${stuObj[prop]} </p>`))
}
}
without trying to catch stuObj.name, still no
for (let i = 0; i < 5; i++) {
let stuObj = (students[i])
console.log(stuObj.name)
for (let prop in stuObj) {
document.write((`<p>${prop} : ${stuObj[prop]} </p>`))
}
}
first access each object and assign to variable then use for in loop to run through each key:value pair in each object, document.write with template literals. I dont know how to catch with if statement and i dont know how to print to dom effectively
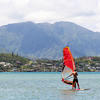
Rihards Selga
5,791 PointsThis works for me. First, put it in for loop to catch the name in h1, then delete the name from object and then do a for in loop to list each key value pair.
for (var i=0; i < students.length; i++) {
var message = '<h1>Student: ' + students[i].name + '</h1>';
delete students[i].name;
for (var key in students[i]) {
message += '<p>' + key + ': ' + students[i][key] + '</p>';
}
document.write(message);
}