Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial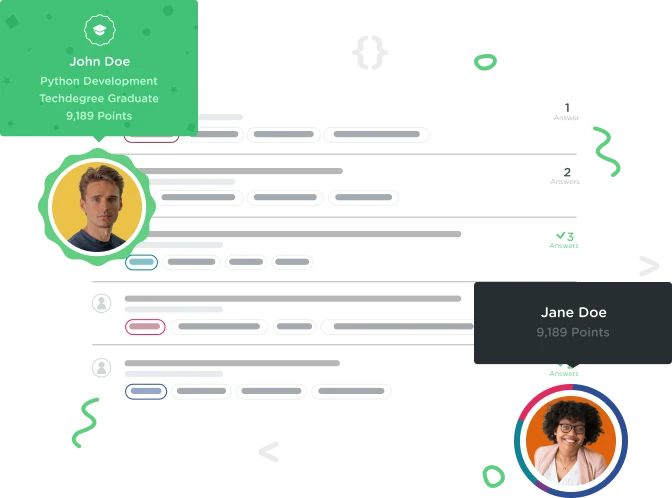
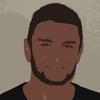
jsdevtom
16,963 Pointsfor ... in ... loop shipping over some items - Python
I've added a few print functions for debugging. These functions show me that the for ... in ... loop skips an item in the list after the prior item has been removed. This makes sense because the current index of the for ... in ... loop will be assigned to the next item after the current item is removed. Thus the index will skip the next item.
How can I remove an item and then get the for loop to continue from the current index again?
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Code for first challenge
the_list.insert(0, the_list.pop(the_list.index(1)))
# Code for second challenge
removed = [] # For debugging
print("the_list before remove section is: {}".format(the_list)) # For debugging
print("The last item in the list is a list?: " + str(type(the_list[-1]) is list)) # For debugging
for item in the_list:
print("Current item in loop is: '{}'".format(item))
if type(item) is str or type(item) is bool or type(item) is list:
removed.append(the_list.pop(the_list.index(item)))
print("The_list after the list remove section is: {}".format(the_list)) # For debugging
print("Removed: {}".format(removed)) # For debugging
1 Answer
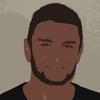
jsdevtom
16,963 PointsSolved this by simply appending the found items and then using this list comprehension: the_list = [a for a in the_list if a not in removed]
.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Code for first challenge
the_list.insert(0, the_list.pop(the_list.index(1)))
# Code for second challenge
removed = [] # For debugging
print("the_list before remove section is: {}".format(the_list)) # For debugging
print("The last item in the list is a list?: " + str(type(the_list[-1]) is list)) # For debugging
for item in the_list:
print("Current item in loop is: '{}'".format(item))
if type(item) is str or type(item) is bool or type(item) is list:
# removed.append(the_list.pop(the_list.index(item)))
removed.append(item)
the_list = [a for a in the_list if a not in removed]
print("The_list after the list remove section is: {}".format(the_list)) # For debugging
print("Removed: {}".format(removed)) # For debugging