Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial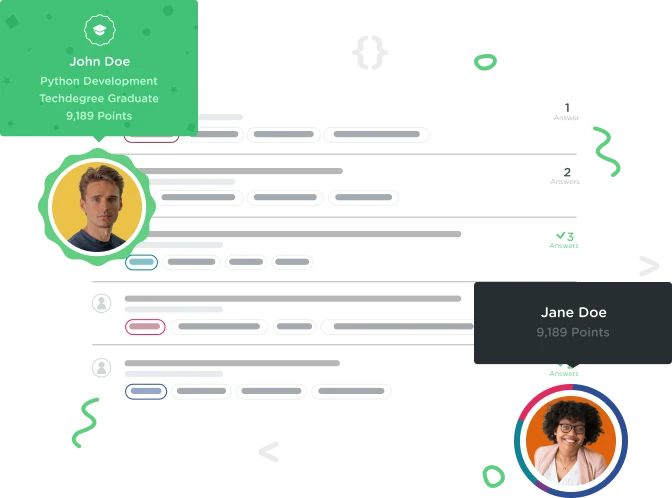
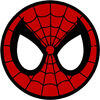
Taylor Hall
Front End Web Development Techdegree Graduate 14,496 Pointsfor in loop The 'prop' part.
Hey guys I hope you are all well. I don't really understand how this bit of code works from the for in loop video:
const person = { name: 'Edward', nickname: 'Duke', city: 'New York', age: 37, isStudent: true, skills: ['JavaScript', 'HTML', 'CSS'] };
for ( let prop in person ) {
console.log(${prop}: ${person[prop]}
);
I don't understand why the word 'prop' makes this loop work the way it does. Thank you
2 Answers
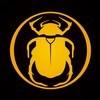
rydavim
18,814 PointsOkay, so prop
is the variable name they're using to describe the properties of the person object. You could call this something else if you wanted, but it's good practice to name variables in a way that relates to what they're meant to refer to.
const person = { // person is the object
name: 'Edward', // name is a property of person
nickname: 'Duke', // nickname is also a property
city: 'New York', // and city...
age: 37, // etc.
isStudent: true,
skills: ['JavaScript', 'HTML', 'CSS']
};
for ( let prop in person ) { // for each property in the person object (name, nickname...)
console.log(`${prop}: ${person[prop]}`); // do this
}
So your loop is iterating over all of the properties in person
and logging out the key (name
) and value (Edward
). The MDN page for for...in
loops is a great reference.
Hopefully that helps, but let me know if you still have questions! Good luck, and happy coding!
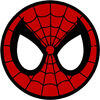
Taylor Hall
Front End Web Development Techdegree Graduate 14,496 PointsHey rydavim,
Thank you for taking the time to reply to my question. Ok I think I understand that it basically can be named anything but I still don't really understand how the code understands to return the key value in the object.
Also: ${person[prop]}`); - is this prop part referring to the value of the person object keys?
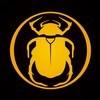
rydavim
18,814 PointsIf you check out the MDN for...in documentation page you'll see that for...in
is being used to loop through the properties of an object with string keys. So, what does this mean?
Example:
const dog = {
name: 'Fido',
breed: 'Mutt',
age: 3
};
So the dog
object has three properties. name
, breed
, and age
are the keys that point to these property values. Try copy-pasting the dog
object above into your browser console. You should be able to find this either by accessing the developer tools in your browser, or by right-clicking > inspect element > console tab.
If we try a console.log(dog.name);
it should output Fido. This is because you're referencing the name
property. Let's use that to loop something similar to the challenge code.
for (let prop in dog) {
console.log(prop);
};
Remember that this is a loop, so that block of code will run three times - one for each string key (prop). What do you think this will output? The loop is running through each property key, so you should get...
name
breed
age
How about if we want to log out both the key and the value?
for (let prop in dog) {
console.log(`${prop}: ${dog[prop]}`);
};
dog[prop]
is using the current key to grab the value of the related property. So in this case you're logging out each key, followed by the value of that property.
name: Fido
breed: Mutt
age: 3
You can think of for...in
as being similar to a shorthand to loop over every property in an object. This way you don't have to mess with things like length
or each
.
Remember that the ${}
notation is not directly related to the use of for...in
. See the MDN page on template literals if you're not sure why you're using that for string formatting here.
Hopefully that helps expand on things, but let me know if I'm not understanding your question correctly. Good luck!