Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial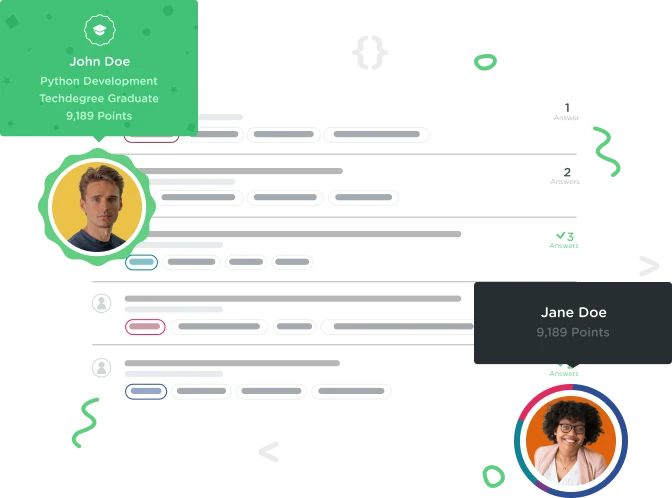

Andrew Mosley
12,328 Pointsfor loop exceeding max conditional?
I have an array of objects that store variables associated with each object.
I'm trying to loop through this array and perform a google maps direction service query on each object to wind up with a series of routes on a map.
The error I'm getting follows: "Uncaught TypeError: Cannot read property 'route' of undefined"
console.logging out the line in question reveals that my counter variable in the for loop is exceeding the limit of the length of the array. Makes sense why it returns undefined. I can't see why this counter should exceed the limit.
Code for the array and loop follows:
var allRoutes = [{
name: "80x",
path: path80x,
route: route80x,
poly: poly80x
},
{
name: "71x",
path: path71x,
route: route71x,
poly: poly71x
},
{
name: "331",
path: path331,
route: route331,
poly: poly331
},
{
name: "43",
path: path43,
route: route43,
poly: poly43
}];
for (var x=0;x<allRoutes.length;x++) {
for (var i=1;i<allRoutes[x].path.length;i++) {
DS.route({
origin: allRoutes[x].path.getAt(i-1),
destination: allRoutes[x].path.getAt(i),
travelMode: google.maps.DirectionsTravelMode.DRIVING
}, function(result, status) {
if (status==google.maps.DirectionsStatus.OK) {
for (var k=0, len=result.routes[0].overview_path.length;k<len;k++) {
allRoutes[x].route.push(result.routes[0].overview_path[k]);
}
}
});
}
allRoutes[x].poly.setPath(allRoutes[x].route);
}
So why is the counter variable 'x' looping through a fifth time?
2 Answers

Greg Kaleka
39,021 PointsAre you sure it's looping a fifth time? I copied and pasted (and changed your object's properties to strings, since I don't have those variables sitting around), and added console.log("x = " + x);
, and it looks to be working to me. I think something else is causing your issue.

Andrew Mosley
12,328 PointsI know it's looping five times because I'm console logging the error line to check what the value of x is when the error is produced. The value is showing 4.