Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial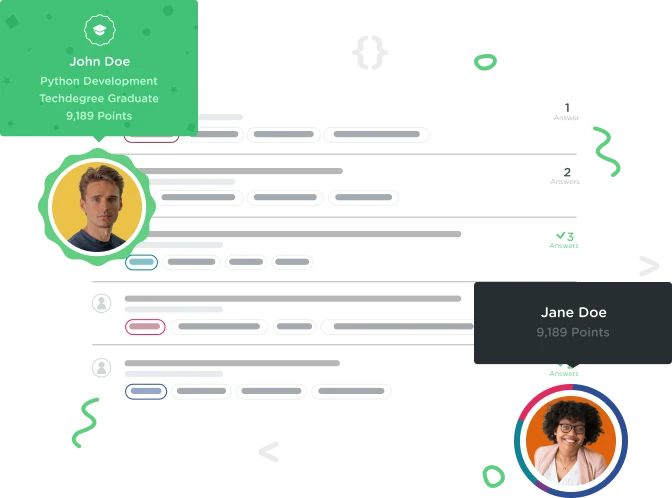
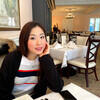
Hanwen Zhang
20,084 PointsFor loop question in querySelector, help please
The for loop cycles over list items and applies a color to each item using the values stored in the colors array. For example, the first color in the array ( #C2272D) is applied to the first list item, the second color (#F8931F) to the second list item, and so on. Complete the code by setting the variable listItems to refer to a collection. The collection should contain all list items in the <ul> element with the ID of rainbow.
var listItems = document.querySelectorAll('#rainbow');
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
4 Answers
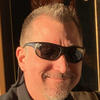
Peter Vann
36,427 PointsHi Hanwen!
You could also use a descendent selector with querySelectorAll like this (it passed this way for me):
let listItems = document.querySelectorAll('#rainbow li'); // still targets the list items collection
const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(let i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
And although var works, it is now customary and considered better practice to declare i with let and the other variables with let and const (but var will certainly work also).
More info:
https://www.w3schools.com/css/css_combinators.asp
https://www.freecodecamp.org/news/var-let-and-const-whats-the-difference/
I hope that helps.
Stay safe and happy coding!

Jassim Alhatem
20,883 PointsHi, Hanwen Zhang,
The challenge is basically asking you to select the list items. What you're doing is selecting all elements that have the id 'rainbow'. What you should do instead is:
- Select the id 'rainbow'.
- Select the children.
.children
Which is an HTML collection, that contains all of the child elements of the node upon which it was called:document.querySelector("#rainbow")
. That query on its own will return the ul element. But adding the.children
property does what I mentioned.
The program handles the rest. Here's the solution.
var listItems = document.querySelector("#rainbow").children;
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
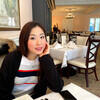
Hanwen Zhang
20,084 PointsThank you Jassim
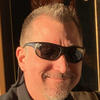
Peter Vann
36,427 PointsAh Jassim, my old nemesis... We meet again!?! Bw-ah-ha-ha-ha!?! LOL
J/K, of course...
Actually, yea I came to find the querySelector and querySelectorAll can be very useful for pinpoint targeting of elements using combinators.
With getElementByID or getElementsByClassName you are limited to targeting the parents and then you have to do a lot of daisy-chaining of child/sibling selector functions, which often gets confusing and convoluted.
I hope your weekend is going well!
Stay safe and happy coding!

Jassim Alhatem
20,883 Pointslol. you too bro xD and thanks for the info.
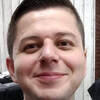
Sergio Andrés Herrera Velásquez
Courses Plus Student 23,765 PointsThank you, this discussion was really helpful, I was really stuck on this.
Jassim Alhatem
20,883 PointsJassim Alhatem
20,883 PointsI actually never knew you could do that, sick!
Hanwen Zhang
20,084 PointsHanwen Zhang
20,084 PointsThank you Peter