Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial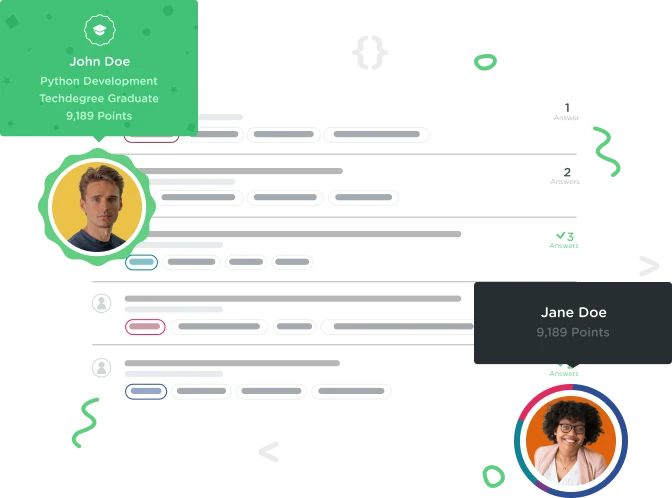
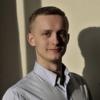
Juraj Majerik
11,624 PointsFor loop vs. For/In loop
Hi! What's the difference between writing a for loop like this:
for (let i = 0; i < lis.length; i += 1) {
...
}
...and this?
for (let i in lis) {
...
}
I thought they would work the same way, but when using the second example, the following error shows up in the console: "Uncaught TypeError: Cannot set property 'display' of undefined at HTMLInputElement.filterCheckBox.addEventListener." Although when running the app in the browser, everything seems to work just fine.
Thanks!
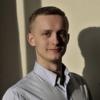
Juraj Majerik
11,624 PointsThanks Zack!
3 Answers
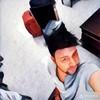
Ari Misha
19,323 PointsHiya there! There are very subtle differences between for loop , for...in loop and for...of loop. Knowing them differences will defo make you a better dev.
- The for loop in Vanilla JavaScript was used when you need loop over a sequence. Mostly an Array , right? As JavaScript got evolved, for loop gave some surprising and unexpected results while iterating over a sequence. It was all coz of Closures and Scope and pollution of Global Scope. This is how for loop looks like:
arr = []
for (var i = 0; i < arr.length; i += 0) {
//....
}
- Comes for...of loop to rescue, ES6 provides you a lots of fixes for the scope leakage of aliases inside the loop. ES6 introduced let , const and arrow function to keep local alias bind to the local scope. Now, for...of loop only iterates over iterables. If you're coming from Python or Ruby, you might already be familiar with iterables. And for a sequence to be an iterable , it should implement @@Iterator method. To sum it all up, for..of loop is recommended and it prevents leakage of scope , unlike for loop. And both loops outputs the same result. To know more about generators , iterables and iterators, visit this link.
arr = [1,2,3,4,5]
for (let num of arr) {
console.log (num)
}
// 1
// 2
// 3
// 4
// 5
- The for...in loop is similar to for...of loop, but there is a gotcha with for..in loop. The for..in loop outputs the indexes or keys if iterating over an iterable. Here is the example and compare it to the example above:
arr = [1,2,3,4,5]
for ( let num in arr) {
console.log (num)
}
// 0
// 1
// 2
// 3
// 4
I hope this helped!
~ Ari
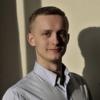
Juraj Majerik
11,624 PointsThanks Ari!

Raja Kannan
6,590 PointsHi Juraj,
Difference between for/in loop and for loop:
For/in loop: *The for/in statement loops through the properties of an object. *The block of code inside the loop will be executed once for each property.
For loop: *The for statement creates a loop that is executed as long as a condition is true. *The loop will continue to run as long as the condition is true. It will only stop when the condition becomes false
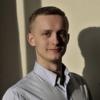
Juraj Majerik
11,624 PointsThanks Raja!

Boyan Anakiev
5,536 PointsThanks Ari , for the detailed response.
Zack Lee
Courses Plus Student 17,662 PointsZack Lee
Courses Plus Student 17,662 Points"Javascript's for..in is designed to iterate over the properties of an object. Producing the key of each property. Using this key combined with the Object's bracket syntax, you can easily access the values you are after."
https://stackoverflow.com/questions/5263847/javascript-loops-for-in-vs-for I googled your question and this was the first thing that showed up. Hope it helps.