Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial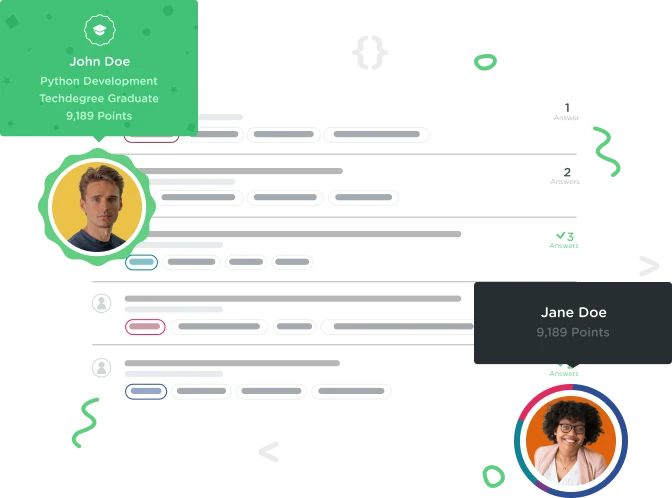

taahrqqhar
14,147 PointsFor some reason my route todos/create is acting as if its todos/{id} ?
For some reason my route todos/create is acting as if its todos/{id} ?
<?php
Route::get('/', 'TodoListController@index');
Route::get('todos', 'TodoListController@index');
Route::get('todos/{id}', 'TodoListController@show');
Route::get('todos/create', "TodoListController@create");
Route::get('db', function() {
$result = DB::table('todo_lists')->where('name', 'Your List')->first();
return $result->name;
});
Route::resource('todos', 'TodoListController');
<?php
public function create()
{
$list = new TodoList();
$list->name = "another list";
$list->save();
return "I am here by accident";
}
1 Answer
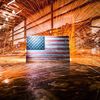
Andrew Shook
31,709 PointsJoe, the reason you are having this problem is because Laravel matches routes in the order in which you put them into the routes.php. In your code above you have your create route after your "id" route. So when Laravel is trying to match the route it hits the "id" route first and thinks the word "create" is the id of a todo. What you need to do is put your "id" route after your create, update, and delete routes like so:
<?php
Route::get('todos/create', "TodoListController@create");
Route::get('todos/{id}', 'TodoListController@show');
or move your resource route above both of the above routes and the resource route will handle all that for you.
taahrqqhar
14,147 Pointstaahrqqhar
14,147 Pointsit works if its /create but soon as I add todos/create it acts as if i was asking for todos/id.. I dont know why