Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial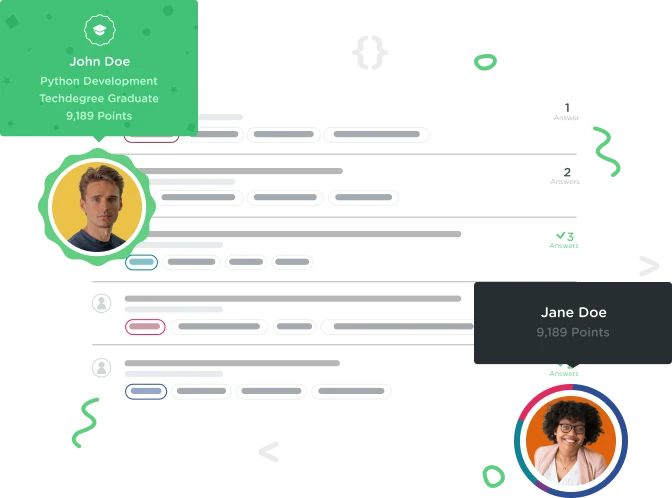

Sam Pereless
5,375 PointsFor some reason when I type into the input field to add a to do event, it shows up blank.. can you help me out as to why
//Problem: User interaction doesnt provide desired results.
//Solution: add interactivity so user can manage daily tasks.
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//New task Liste Item
var createNewTaskElement = function(taskString) {
//Create List Item
var listItem = document.createElement("li");
//input (checkbox)
var checkBox = document.createElement("input"); //checkbox
//label
var label = document.createElement("label");
// input (text)
var editInput = document.createElement("input");
//button.edit
var editButton = document.createElement("button");
//button.delete
var deleteButton = document.createElement("button");
//Each elements, needs modifying
checkBox.type = "checkbox";
editInput.type = "text";
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
deleteButton.className = "delete";
//Each element needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//Add a new Task
var addTask = function() {
console.log("Add task...");
//Create a new list item with text from #new-task:
var listItem = createNewTaskElement(taskInput.value);
//Append listItem to incompleteTasksHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Edit an existing Task
var editTask = function () {
console.log("Edit task...");
var listItem = this.parentNode;
var editInput = listItem.querySelector("input[type=text]");
var label = listItem.querySelector("label");
var containsClass = listItem.classList.contains("editMode");
//if the class of the parent is .editMode
if(containsClass) {
//switch from .editMode
//label text become the input's value
label.innerText = editInput.value;
}else {
//switch to .editMode
//input value becomes the label's text
editInput.value = label.innerText;
}
//Toggle .editMode on list item
listItem.classList.toggle("editMode");
}
//Delete an existing Task
var deleteTask = function () {
console.log("Delete task...");
var listItem = this.parentNode;
var ul = listItem.parentNode;
// Remove the parent list item from the ul
ul.removeChild(listItem);
}
//Mark a task as complete
var taskCompleted = function () {
console.log("Task Incomplete...");
//Append the task list item to the completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//Mark a task as incomplete
var taskIncomplete = function () {
console.log("Task Complete...");
//Append the task list item to the uncomplete-tasks
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to the checkbox
checkBox.onchange = checkBoxEventHandler;
}
//Set the click handler to the addTask function
addButton.onclick = addTask;
//cycle over incompleteTasksHolder ul list items
for(var i=0; i<incompleteTasksHolder.children.length; i++){
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
for(var i=0; i<completedTasksHolder.children.length; i++){
//bind events to list item's children (taskIncomplete)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
4 Answers
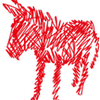
Matteo Mainardi
7,372 Pointshi Sam Pereless, I'd the same problem, but there is a solution: the input value is there, as you can check with console.log(taskInput.value); , but Firefox has problems with the property innerText, so you need to use textContent instead:
editButton.textContent = "Edit";
deleteButton.textContent = "Delete";
label.textContent = taskString;
you also need to substitute the properties in editTask, if you don't want an undefined value when switching to edit mode:
editInput.value = label.textContent;
label.textContent = editInput.value;
hope this helps

Iain Simmons
Treehouse Moderator 32,305 PointsI'm a little late to the party, but it's not actually the innerText property that is the issue here.
It's the fact that Andrew has unfortunately not closed the querySelect argument properly (1:08 in the video):
var editInput = listItem.querySelector("input[type=text");
Should be:
var editInput = listItem.querySelector("input[type=text]");
i.e. it needs to have the closing square bracket for the attribute selector.
I was surprised that Chrome and IE11 didn't throw any errors regarding the incorrect syntax.

amirsalehi
24,667 PointsHi Andrew Chalkley I have the same problem when use Firefox. I downloaded the project_stage_3_end folder and I think there is a bug within the code. I found this in Line 65 (app.js) and fixed it but still get an empty task in my ToDo list.
"""
var editInput = listItem.querySelector("input[type=text");
"""
Could you please try to run the stage 3 code with FIreFox.
Many thanks.

Sam Pereless
5,375 PointsTHANK YOU!

notf0und
11,940 PointsDon't forget to select best answers :)
Kenneth Shim
17,969 PointsKenneth Shim
17,969 Pointsthis helped me. Thanks. :)