Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial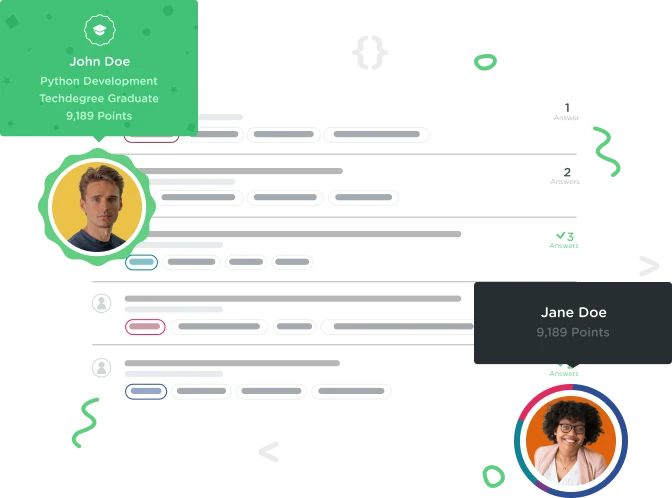

Joel Thompson
1,729 PointsFor some reason when using the __str__ print method it prints the HP and XP as tuples.
Using print(draco) returns:
'Color' Dragon, HP: (8,) XP: (6,)
I have looked through the code and can't seem to figure out where I'm going wrong. How can I ensure that it returns the values outside of a tuple?

Joel Thompson
1,729 Pointssorry I meant the color prints fine I was just leaving it variable.

Gavin Ralston
28,770 PointsDo you happen to have a copy of the function you could post?

Joel Thompson
1,729 Pointsclass Monster(Combat):
min_hp = 1
max_hp = 1
min_xp = 1
max_xp = 1
weapon = 'sword'
sound = 'roar'
def __init__(self, **kwargs):
self.hp = random.randint(self.min_hp, self.max_hp),
self.xp = random.randint(self.min_xp, self.max_xp),
self.color = random.choice(COLORS)
for key, value in kwargs.items():
setattr(self, key, value)
def __str__(self):
return '{} {}, HP: {}, XP: {}'.format(self.color.title(),
self.__class__.__name__,
self.hp,
self.xp)
def battlecry(self):
return self.sound.upper()
class Goblin(Monster):
max_hp = 3
max_xp = 2
sound = 'squeak'
class Troll(Monster):
min_hp = 3
max_hp = 5
min_xp = 2
max_xp = 6
sound = 'growl'
class Dragon(Monster):
min_hp = 5
max_hp = 10
min_xp = 6
max_xp = 10
sound = 'raaaaaaaaaawr'

Gavin Ralston
28,770 PointsWell, you're inheriting from the Combat class, which I removed and just had it inherit from object to test it
as for the init function, you could remove the commas between the attribute settings, as they're not necessary. It seems those commas are creating tuples when all you wanted was integers
def __init__(self, **kwargs):
self.hp = random.randint(self.min_hp, self.max_hp), # <--- you're creating tuples here with
self.xp = random.randint(self.min_xp, self.max_xp), # the unnecessary commas
self.color = random.choice(COLORS)

Joel Thompson
1,729 PointsThanks Gavin! Don't know how long I would have had to look for those commas. I appreciate the help.

Gavin Ralston
28,770 PointsNo problem.
I'm going to post this as an answer in case something like this comes up again.
1 Answer

Gavin Ralston
28,770 PointsIn the init function, remove the commas between the attribute settings, as they're not necessary, and if you recall from the videos, comma create tuples.
It seems those commas are creating tuples when all you wanted was integers
def __init__(self, **kwargs):
self.hp = random.randint(self.min_hp, self.max_hp), # <--- you're creating tuples here with
self.xp = random.randint(self.min_xp, self.max_xp), # the unnecessary commas
self.color = random.choice(COLORS)
Gavin Ralston
28,770 PointsGavin Ralston
28,770 PointsIt might help to see the code, because it's also returning the word "color" in title case instead of the color attribute of the object, I think.
Chances are good you wrapped the format arguments and "lucked out" by having the tuple contents match exactly the remaining arguments needed for the format method.