Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial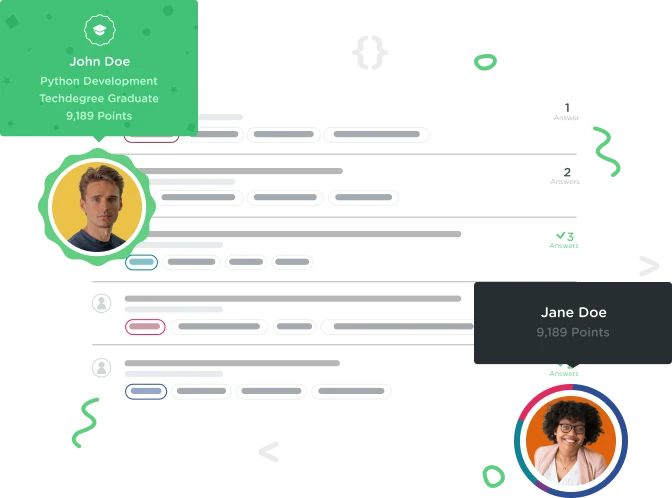
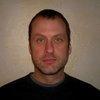
Bjorn Chambless
Treehouse Guest TeacherFor task 3, ordering by date, it seems an answer that should work is: bp.mCreationDate.compareTo(this.mCreationDate)
Modifying the compareTo() such that it returns (bp is cast obj):
bp.mCreationDate.compareTo(this.mCreationDate)
The interface kicks me back to task 1
package com.example;
import java.util.Date;
public class BlogPost implements Comparable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public int compareTo(Object obj){
BlogPost bp = (BlogPost)obj;
if (bp==this){
return 0;
}
//return bp.mCreationDate.compareTo(this.mCreationDate);
return 1;
}
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
Also tried:
public int compareTo(Object obj){ BlogPost bp = (BlogPost)obj; if (bp == this){ return 0; } else { return bp.mCreationDate.compareTo(mCreationDate); } //return 1; }
Seeing: "Oops! It looks like Task 1 is no longer passing" presumably due to commenting out "return 1". However, leaving it in produces an unreachable code error.
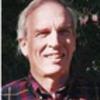
jcorum
71,830 PointsMark, just a note that the @Override annotation is not required.
4 Answers
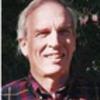
jcorum
71,830 PointsThis works:
public Date getCreationDate() {
return mCreationDate;
}
public int compareTo(Object obj){
BlogPost bp = (BlogPost)obj;
if (bp==this){
return 0;
}
return this.mCreationDate.compareTo(bp.mCreationDate);
//return 1;
}
To get it past Tasks 1 and 2 you need return 1;, which is why it's commented out, rather than deleted. To get it past Task 3 I had to change it to this.mCreationDate.compareTo(bp.mCreationDate) rather than the reverse: bp.mCreationDate.compareTo(this.mCreationDate)
I swear it worked the other way round earlier, as I passed the challenge. Anyway, this code just passed the challenge, as amended.
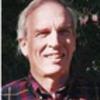
jcorum
71,830 PointsWhen I run your code in the challenge I get a different error message. I just copied your code from above:
public int compareTo(Object obj){
BlogPost bp = (BlogPost)obj;
if (bp==this){
return 0;
}
//return bp.mCreationDate.compareTo(this.mCreationDate);
return 1;
}
The error message is: Expected -1 but received 1...
You need to uncomment this line:
//return bp.mCreationDate.compareTo(this.mCreationDate);
and remove return 1;
Any compareTo() method must return a 0 if the objects are equal, and a -1 or 1 if they are not. The reason is that compareTo() is most often used for sorting, and the sorting algorithm needs to know if current BlogPost is before, equal to, or after the one passed in as an argument.
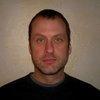
Bjorn Chambless
Treehouse Guest TeacherThanks for responding...
I tried that. My first solution was actually:
public int compareTo(Object obj){ BlogPost bp = (BlogPost)obj; return bp.mCreationDate.compareTo(this.mCreationDate); }
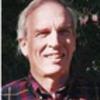
jcorum
71,830 PointsThen I don't understand your question, since that code works??
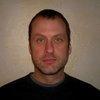
Bjorn Chambless
Treehouse Guest TeacherI just ran this solution(copied and pasted) for Task 3:
public int compareTo(Object obj){ BlogPost bp = (BlogPost)obj; return bp.mCreationDate.compareTo(this.mCreationDate); }
"Ooops! It looks like Task 1 is no longer passing"
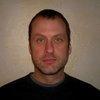
Bjorn Chambless
Treehouse Guest TeacherThanks, that worked.
Mark Miller
45,831 PointsMark Miller
45,831 PointsYou must add the annotation @Override one line before the method declaration line, for the method compareTo(), because you are creating an extended version of the method. Remember, you are also then calling the built-in version of the method after first testing for equality. Finally, make sure you uncomment your code line, and delete the 'return 1' because you can execute only one 'return' statement.