Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial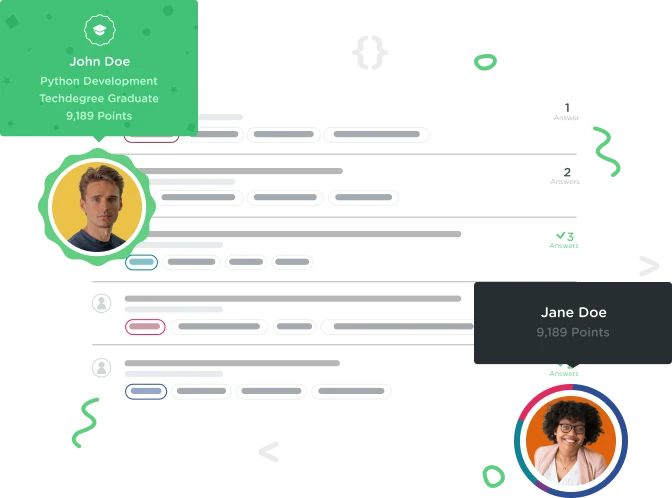
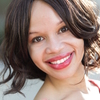
Lorraine Wheat
6,083 PointsFor the statement "Math.floor(Math.random() * (6 - 1 + 1)) + 1;" why am I subtracting 1 from 6 and then adding 1?
Can't I just multiply it by 6?
7 Answers
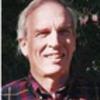
jcorum
71,830 PointsYou could.
Your line of code, when written as a formula, amounts to the following:
Math.floor(Math.random() * x) + lowValue), where x = highValue - lowValue + 1
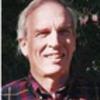
jcorum
71,830 PointsNot sure I understand your question. To get a random number between 1 and 6 you can do this:
Math.floor((Math.random() * 6) + 1);
You need to add the 1 because the random() function returns a number between 0 (inclusive) and 1 (exclusive).
The formula you are questioning is for cases where the lower bound is not 1.
So if you want a random number between 50 and 75 you need
Math.floor((Math.random() * (75 - 50 + 1) + 50);
Here you are multiplying the random number by 26 (which gives a random number between 0 and 25, and then you are adding 50 to that number, giving a random number between 50 and 75.
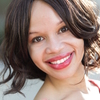
Lorraine Wheat
6,083 PointsThat better explains it. But then my question would be why wouldn't I just do Math.floor((Math.random()*26) + 50);
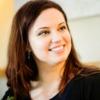
Emily Carey
7,954 Pointshttps://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/random
According to MDN, it's a case of whether you want to make the max number INclusive or EXclusive: 1.) Returns a random integer between min (included) and max (excluded)
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min)) + min;
}
2.) Returns a random integer between min (included) and max (included)
function getRandomIntInclusive(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
So the formula that they provide for this challenge already sets you up to have the maximum number included in the possible outcomes of the random number generated, as the +1 indicates. - I was thrown at first too.. better formula to have been given first off would have been the one stated on MDN, then the min and max and +1 are clear in why each are being used.
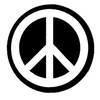
john larson
16,594 PointsEmily, maybe you could teach how to read the MDN documentation, it's Greek to me.
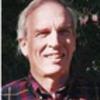
jcorum
71,830 PointsThe formula is built to work for any upper and lower values, not just 1 for the lower value. If you wanted a random number between 50 and 75 you wouldn't be able to just multiply by 75.
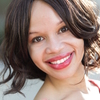
Lorraine Wheat
6,083 PointsBut doesn't 6-1+1 = 6? And does not it evaluate first what is inside the parantheses before it is multiplied by Math.random()? How does the 6-1+1 give it the versatility?
Mehran Moradi
11,140 PointsYour questions and Answers were useful. Thanks all

nagamani palamuthi
6,160 Pointscan anyone explain to me how mdn came up with this formula? i dont get why we subtract to get the range max-min+1 I saw the MDN and thnx. to Emily for reminding to look there. still not clear how they arrive at that formula given in MDN
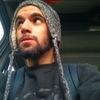
Cosimo Scarpa
14,047 PointsThe code need to be something like this:
function getRandom (lower, upper) {
return Math.floor((Math.random() * (upper - lower + 1) + lower);
}
console.log( getRandom(10, 20) );
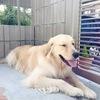
Julio Akiyama
9,068 PointsI guess I understood. Thanks to jcorum. Forget about the (6 -1 +1 ). Let's change the 6 to up and -1 to down, then we have (up - down +1). The 6 and 1 could be any other number, but the +1 need to always be there, cause it is the formula. Is it right?
Lorraine Wheat
6,083 PointsLorraine Wheat
6,083 PointsThank you. That was really helpful. I definitely had to see it broken down visually to understand it.
john larson
16,594 Pointsjohn larson
16,594 Pointsj, your explanations are concise and understandable... thank you