Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial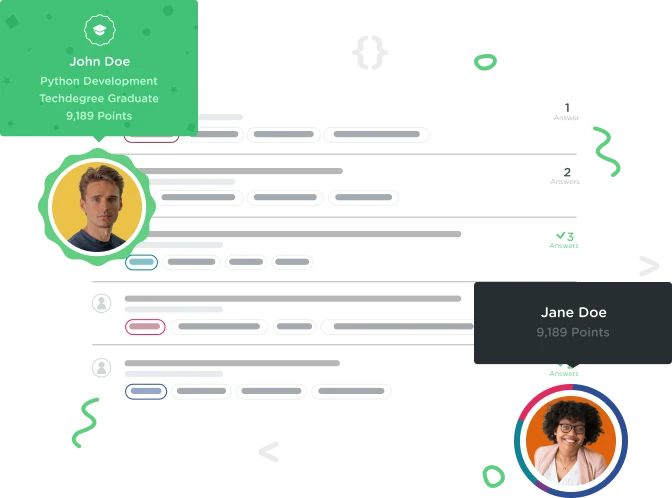

walter fernandez
4,992 PointsFor this challenge, we'd like to know in a range of values from 1 to 100, how many numbers are both odd, and a multiple
help
var results: [Int] = []
for n in 1...100 {
// Enter your code below
// End code
}
1 Answer
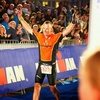
Steve Hunter
57,712 PointsHi Walter,
In this challenge you are provided with a loop that iterates from 1 to 100 - at each iteration, the current value is held within the loop's variable, n
. So, n
starts out as 1, then 2, 3, etc.
You want to add the value of n
into the results
array (also provided for you), if
the value of n
at the time is both odd and a multiple of 7.
You can check for oddness by using the mod operator, %
and the number 2. If a number is even number % 2
will equal zero. If the number is odd, that operation will generate a non-zero result. (It'll be 1, but let's just stick to non-zero, or not
(!
) zero). So that's the first test sorted, we want to see if n % 2 != 0
, in other words, does the result of n % 2
generate a non-zero result.
Next we need to see if the value of n
is a multiple of 7. We can use the same process. The mod operator, %
, and the figure 7 will tell us if number divided by seven generates a remainder. If no remainder is generated, a zero result, then the number is a multiple of 7. That means our test is the same as with the odd test, but by using the value of 7 and checking for a zero result, not a non-zero one. So, that looks like n % 7 == 0
.
We have our two tests. Both need to apply at the same time, so test 1 AND test 2, must be true for the current value of n
to be added to the results
array. We can AND two tests together using a double ampersand, &&
.
This gives us the test:
if n % 2 != 0 && n % 7 == 0 {
// add n to the array
}
OK so far?
Now the easy bit. How do we add an element to an array? We use the append()
method and dot-notation. The array is called results
and the parameter we pass into append()
is n
.
That gives us:
if n % 2 != 0 && n % 7 == 0 {
// add n to the array
results.append(n)
}
Let me know how you get on.
Steve.