Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial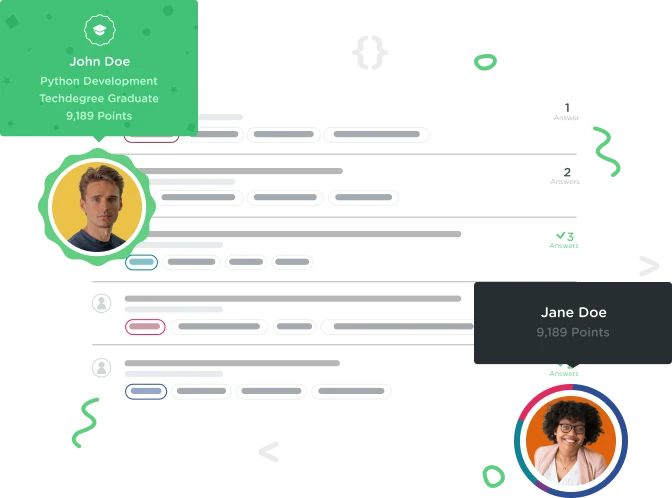
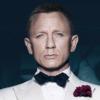
Aurelian Spodarec
10,801 PointsFor those learning JavaScript - Part 1
HI,
SO I'm learning JS, and so I'm just going to write it on treehouse, if I misunderstood something or whatever.
So I was making a timer, here it goes:
How do we make a timer that updates every second without refreshing the browser? We do it with JavaScript, because that's what is the idea behind JS.
So JS, has already a function build in (which we could make one too), that has already the objects or the values in.
If i got to mozilla JS developers https://developer.mozilla.org/pl/docs/Web/JavaScript/Referencje/Obiekty/Date
We have different umm, values I suppose, like day, year, months etc...
So what we do is we create a new variable, and we put in the function or rather an object with those paramaters saved in.
var today = new Date();
Now we store all the functions that the object has inside the Date(), i think.
To 'access' the day, month , basically, to access the values inside the Date object, we need to specify the date like
var day = today.getDate();
We created a new varibale for the day, since object today, has many variables, and we want a specific value from it, which is date, so we look in the Date object, and we take what we need, so that's date.
We do this with all the other, month year etc.
So now we got the variables.
We need html for that date, right?
Let's created HTML
<div id="clock"></div>
What's happening? We created a HTML makrup with id of clock.
Now, we need to put int the values inside that clock, right?
We need to select the element with JS, so we need to target the browser, which is document, and we need are going to look for an id (id stands for identification - it's unique ) like this:
document.getElementById("clock");
Now, we selected the element with the id of clock.
We want to put inside the values, so we need to use a JS something functino? to put in.
We need to put inside it, so it's 'inner' because inner is like inside. and we want to put it in HTML, so it's innerHTML, what do we want to put? Well the day, month, year hour minute, second.
document.getElementById("clock").innerHTML =
day+"/"+month+"/"+year+" |"+hour+":"+minute+":"+second;
The + is concatinating, if you did PHP, then in PHP you concatinate with a .dot.
What goes in between the "quotes" is what we want the HTML to look like.
That's great, we did a clock.
That's not the end though. It won't magically apear or work in the HTML.
We now need to make this in a function, so it's a reusable code.
So let's make a function timeCount
function timeCount() {
}
Each time when we call it, whatever is between the paranthesis, will be done. The function won't activate it's self alone though.
Let's put in there the code
function timeCount() {
var today = new Date();
var day = today.getDate();
var month = today.getMonth()+1;
var year = today.getFullYear();
var hour = today.getHours();
if(hour<10)hour = "0"+hour;
var minute = today.getMinutes();
if(minute<10)minute = "0"+minute;
var second = today.getSeconds();
if(second<10)second = "0"+second;
document.getElementById("clock").innerHTML =
day+"/"+month+"/"+year+" |"+hour+":"+minute+":"+second;
}
Great! Right? Well, sure, but it's not quite finished. We need to update this, and since one the clock time updates every second, JS has a specific function that updates something , starting from mili seconds, so 1000 is one second.
Let's use a setTimeout function and update our timeClock every 1 second. So it looks like:
function timeCount() {
var today = new Date();
var day = today.getDate();
var month = today.getMonth()+1;
var year = today.getFullYear();
var hour = today.getHours();
if(hour<10)hour = "0"+hour;
var minute = today.getMinutes();
if(minute<10)minute = "0"+minute;
var second = today.getSeconds();
if(second<10)second = "0"+second;
document.getElementById("clock").innerHTML =
day+"/"+month+"/"+year+" |"+hour+":"+minute+":"+second;
setTimeout("timeCount()", 1000);
}
Now, what do we want to update? the timeCount, so that's why we put it there, and update it, or refresh it every second, without the browser actually updating.
We can use setTimeout, to update the carousel, to change the slide.
Hope this helps! Let me know if you liked this and if you have anything to add, id be happy to learn new stuff! I believe that teaching is a great way of learning, because you go in deep. Now when I was trying to make a slider, now I understand how the setTimeout works, I know how to get the function etc.
Remember that a function is doing a specific task. E.g. WHat happens if you click read more, and you have the code e.g. expand on click. Or next button will cause the image to show up that is in the right.. lol etc.
Slowly but steady. Since I did JS before, I think.. I only think this will come easier, at least the very basics, to do basic stuff for web. I hope. I can remember how the stuff works, so that's good.
Plus mozilla is a great place, mozilla developers website for JS.
Here's the final version on codepen! http://codepen.io/Aurelian/pen/NdGYmM
1 Answer
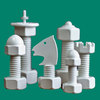
Steven Parker
230,274 PointsDon't forget setInterval()
It's similar to setTimeout() in that you pass it a function and a delay time in milliseconds, but you don't have to keep calling it. Once you have set an interval, it will keep calling the function over and over until you tell it to stop (using clearInterval()), so it makes a good alternative to setTimeout() for implementing a clock.
For more information, here's the MDN page for it.
Aurelian Spodarec
10,801 PointsAurelian Spodarec
10,801 PointsMen, JS is robust! : p setInterval, so if I was to make a carousel, I would use setInterval, and then clear it once the slide is one and activate it again?
Or this is just one of the way to do it?
I'm trying to get all the functions, and all the e.g. innerHTML stuff in my head, so I can quickly manipulate, slide and add action to JS, and slowly I understand how this is.
In one way, everything is a function, a button, slide, click, and everythins is a chain, if you click button, activate function nextImage, which is to move the image 100% left and the other image will apear, or something.
If you want to close, click the X, so click X and etc.. I think some jQuery before knowledge helps too, but I need to learn pure JS.
Hopefully I can have a knowledge in 20days to do the basic web stuff, modals, gallery thsi that.. I sort of could do that with jQuery but well, still need to learn, so now I spend about 6hours learning JS.
Aurelian Spodarec
10,801 PointsAurelian Spodarec
10,801 PointsBtw
WHen you write a function.e.g clock function, you need to set the setIntreval or setTimeout inside that function, and you need to say what you want to do , because it will update the function every few sec.
So function clock, and then setTimeout(clock), 3sec ,refresh or update that function every 3 sec.
What happen if that build in function was to be outside the clock function? would it still update? Let's see, setTimeout we select the clock to update 3 sec, but then, that would be global, so we want this function to work only in the setTimeout function. Yeah, i think that's it.
Aurelian Spodarec
10,801 PointsAurelian Spodarec
10,801 PointsSo if I was to make a carousel.