Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial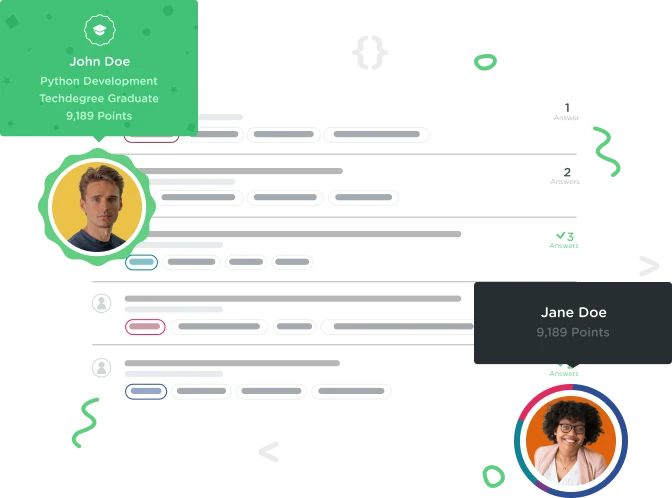
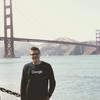
Ben Ahlander
7,528 Pointsfor (var key in object)
I don't really understand the difference between these two loops? Do you only use the “for(var key in object)” loop if there is one object in the object literal? (not sure i worded that correctly. if not, please correct my wording) or am i using the second loop wrong?
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Students</title>
<link rel="stylesheet" href="css/styles.css">
<!-- Latest compiled and minified CSS -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<!-- jQuery library -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<!-- Latest compiled JavaScript -->
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<h1>Students</h1>
<div class="row">
<div class ="col-sm-6" id="output"></div>
<div class="col-sm-6"id="output2"></div>
</div>
<script src="js/students.js"></script>
</body>
</html>
var students = [
{
name: 'Ben Ahlander',
track: 'JavaScript',
achivments: 'gold star',
points: '100',
computer: 'mac'
},
{
name: 'Ani Ahlander',
track: 'JavaScript',
achivments: 'gold star',
points: '100',
computer: 'mac'
},
{
name: 'Eli Ahlander',
track: 'JavaScript',
achivments: 'gold star',
points: '100',
computer: 'mac'
},
{
name: 'Ava Ahlander',
track: 'JavaScript',
achivments: 'gold star',
points: '100',
computer: 'mac'
},
{
name: 'Rick Ahlander',
track: 'JavaScript',
achivments: 'gold star',
points: '100',
computer: 'mac'
}
];
var message = '';
var student = '';
var message2 = '';
var student2 = '';
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function print2(message) {
var outputDiv = document.getElementById('output2');
outputDiv.innerHTML = message;
}
for (var i = 0; i < students.length; i++){
student = students[i];
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Achivments: ' + student.achivments + '</p>';
message += '<p>Points: ' + student.points + '</h2>';
message += '<p>Computer: ' + student.computer + '</p>';
print(message);
}
for(var key in students){
message2 = '<h2>Student: ' + students[key].name + '</h2>';
message2 += '<p>Track: ' + students[key].track + '</p>';
message2 += '<p>Achivments: ' + students[key].achivments + '</p>';
message2 += '<p>Points: ' + students[key].points + '</p>';
message2 += '<p>Computer: ' + students[key].computer + '</p>';
print2(message2);
}
3 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Ben,
Your problem is in this line here:
message2 = '<h2>Student: ' + students[key].name + '</h2>';
You used the assignment operator which is going to reset the message2 variable to the current students name and you'll lose what was previously in there.
If you use the +=
operator instead then it will continually add each new student to what you previously had.
Also, you should not use a for...in
loop to iterate over arrays. The order of retrieval is not guaranteed.
It is recommended that you use a normal for loop like you did. Or you could use a for...of
loop instead.
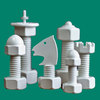
Steven Parker
229,771 PointsBoth loops should be very similar in this example.
The big difference is that the first one keeps adding to the message ("+=
") and the second one starts the message over each time ("=
"). So the first loop shows all students, but the second one keeps replacing the output and shows only one.
Neither of these loops deal with the objects in the array directly.
But the looping part is much alike since the array is not "sparse" and contains no non-numeric keys. Otherwise, only the "for" loop (the first one) would display the undefined elements of the sparse array, and only the "for...in
" loop (second) would display the items with non-numeric keys.
To experiment with these concepts, you could try both kinds of loops on an array like this:
test = ["zero", "one", "two"]
test[4] = "four"; // this makes the array "sparse" with index 3 undefined
test["extra"] = "surprise!"; // this adds a non-numeric key

Pablo Villegas
2,922 Points"Basically" equivalent: take a look at https://stackoverflow.com/questions/500504/why-is-using-for-in-with-array-iteration-a-bad-idea?lq=1 for different behaviors. One relies on the enumerable properties of students
and the other is just accessing an array by index. In this case, both produce the same output.
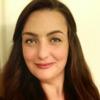
Jennifer Nordell
Treehouse TeacherHi there, Pablo! I changed your comment to an answer to allow for upvoting and possible selection for Best Answer. Thanks for helping out in the Community!
Ben Ahlander
7,528 PointsBen Ahlander
7,528 PointsThe problem is that the first loop is display all the info correctly. The second loop is only displaying the last object.