Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial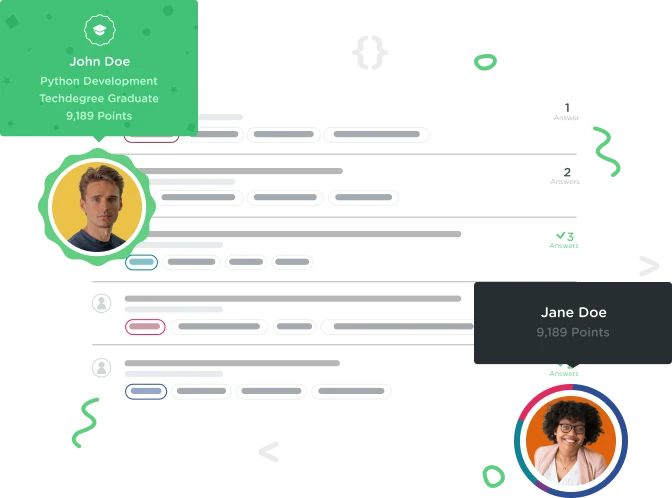

Mo Perl
1,195 Pointsfor-each loop
how do you do the for each loop
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(
}
2 Answers

Simon Coates
28,694 Pointsthe foreach syntax is
for(data_type variable : array | collection){}
the data type is char. suggest calling the variable aLetter. The collection/array is retrieved by calling a method on the string (toCharArray). So the loop bit is:
public int getTileCount(char inLetter){
int count = 0;
for(char aLetter: mHand.toCharArray()){
//ADD CODE TO INCREMENT HERE (it compares aLetter and inLetter using ==, and an if statement)
}
return count;
}
OR if you just want the complete code, you should be able to find this using the forum.
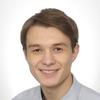
Sergey Podgornyy
20,660 Points- You need to create a method called getTileCount. Your method needs to return an integer (the count), and it needs to take in a char (the letter to check for.)
- You would want to initialize the count as 0, and before your conditional logic.
- You need to use a for loop to loop through the tiles.
- After initializing the counter variable, you need to write the for loop (so that you can 'run' through each tile.) Then, you need to check if the particular tile matches. If the test is met, you would increment the counter, because there would be a match.
- You need to return the count when it is ready to be returned (after all tiles are checked.)
public int getTileCount(char t){
int count = 0;
for (char tile : mHand.toCharArray()){
if (tile == t) {
count++;
}
}
return count;
}