Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial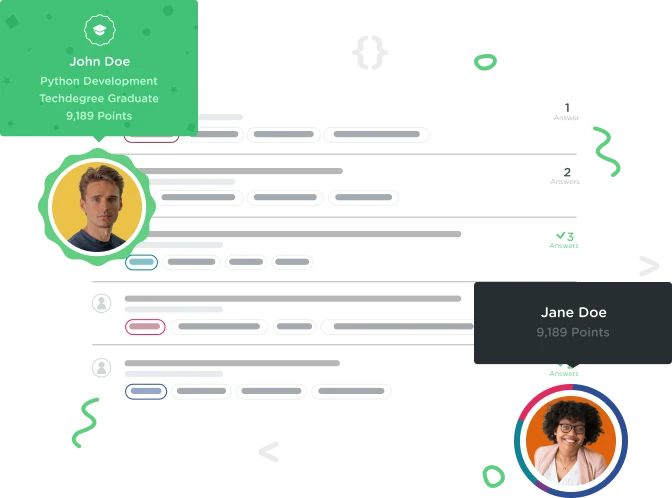

Ryan Bartusek
5,999 PointsFor-each loop trouble..
public int getTileCount(char tile) { int count = 0; for (char tile: mHand.toCharArray()) { if (mHand.indexOf(tile) >= 0) { count++; } } return count; }
I'm having a bit of trouble incrementing the count if there is a tile in the hand of the player.. Hint says I need to take in a char in the signature, and I can't imagine what else that would be other than the tile, but when I do the for-each loop it says it's already initilized. Not sure where to go from here.. thanks for taking a look. :)
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char tile) {
int count = 0;
for (char tile: mHand.toCharArray()) {
if (mHand.indexOf(tile) >= 0) {
count++;
}
}
return count;
}
}
1 Answer

Simon Coates
28,694 Pointsit accepts
public int getTileCount(char inTile) {
int count = 0;
for (char tile: mHand.toCharArray()) {
if (inTile == tile) {
count++;
}
}
return count;
}
your problems were that you were using indexOf rather than == (which is okay for comparing primitive data types), and you were trying to create two variables called tile in the local scope.
Ryan Bartusek
5,999 PointsRyan Bartusek
5,999 PointsAwesome. Thanks for the advice Simon!
Simon Coates
28,694 PointsSimon Coates
28,694 PointsA lot of people get tripped over by that one. i guess everyone thinks "how do i do it?" and then see the indexOf method in the class, assuming it's there as a hint. indexOf is pretty useful under normal circumstance but requires a little work (breaking the string into components or testing on a part of the string*) if needing to detect multiple instances of something. Using the character comparison here is simpler.