Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial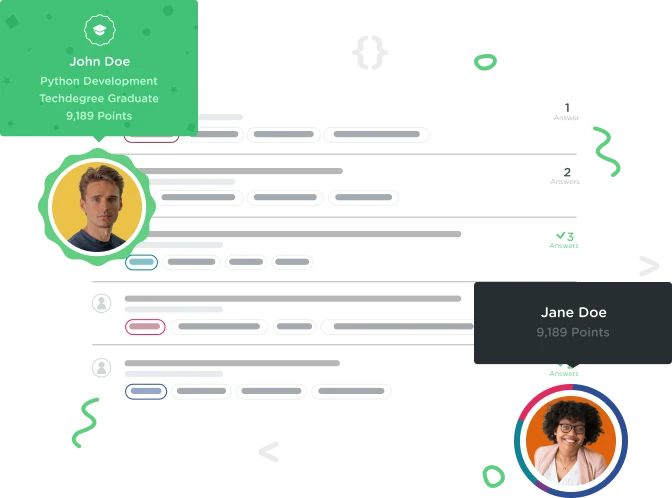

Charlie Gallentine
934 PointsFor/each loops and char arrays. Where is my thinking going wrong on this exercise?
I'm lost. Thanks for any help!
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public String getTileCount(char tile){
for(char tile : mHand.toCharArray()) {
System.out.println("We have: " + tile);
}
return mHand;
}
}
3 Answers

anil rahman
7,786 Points public int getTileCount(char tile){ --pass in a char
int count = 0; -- set counter
for(char letter:mHand.toCharArray()){ -- for each letter in the hand
if(letter == tile){ -- and if letter in the hand matches the tile
count++; --increase count
}
}
return count;--return the count variable
}
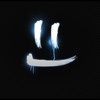
Grigorij Schleifer
10,365 PointsHi Charlie,
you need an integer variable to count the characters in mHand. In the beginning set it to 0. Use the for loop but call your char variable different than the argument name. If that char is equals to the argument char. Increment the count. Aftre the loop is done, return the counter.
You code can look like this:
public int getTileCount (char tile) {
int count = 0;
for (char tiles : mHand.toCharArray()) {
if (tile == tiles) {
count++;
}
}
return count;
}
Makes sense?
Grigorij

Lukas Dahlberg
53,736 PointsIn this challenge, you are looping through the already existing mHands string to find out how many matches there are to any given tile and then returning the integer count (not the the System.out.println() of each).
public Integer getTileCount(char tile) {
Integer count = 0;
for (char letter : mHand.toCharArray()) { if (tile == letter) { count++; } } return count; }
Ivan Kazakov
Courses Plus Student 43,317 PointsIvan Kazakov
Courses Plus Student 43,317 PointsHello Charlie,
your method should return a number of times the 'mHand' contains given 'tile'. So the return type should be int.
In this case to return an integer it's good to declare one first, and set an initial value of 0:
int count = 0;
Then you'd perform a char-by-char comparison of 'mHand' chars with the 'tile', using for/each loop, where you would increment 'count' for each matching evaluation:
then you return the value of a 'count' integer variable, which may vary from 0 to the length of an 'mHand' string (if all of its chars are equal to the inspected tile:
return count;