Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial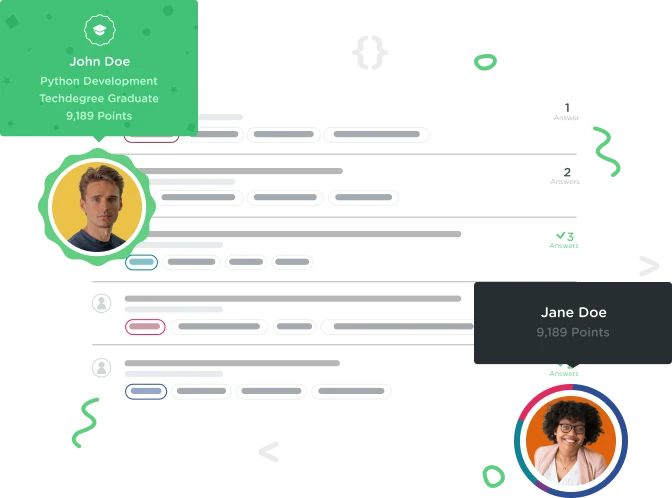
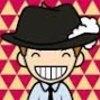
Aaron Selonke
10,323 Pointsforeach-if-statment w/ Linq conditional giving unexpected results
This is a part of a Tic-Tac-Toe game. the checkmate method returns a string that will be used by another fucntion (not shown here) to make a tic-tack-toe move.
Here is the board, the player has two pieces lined up on the top row
public static char[] board = { '+', '+', 'E',
'A', 'S', 'D',
'Z', 'X', 'C' };
private static string checkmate()
{
List<char[]> dalist = new List<char[]> {
new char[3] { board[3], board[4], board[5] },
new char[3] { board[0], board[4], board[8] },
new char[3] { board[2], board[4], board[6] },
new char[3] { board[1], board[4], board[7] },
new char[3] { board[0], board[1], board[2] },
new char[3] { board[6], board[7], board[8] },
new char[3] { board[0], board[3], board[6] },
new char[3] { board[2], board[5], board[8] }
};
The method returns a single letter as a string that corresponds to a move on the board.
The method analyses the board by creating a list of the 8 possible winning patterns in tic-tack-toe, and testing them against what is already on the board
the logic four components that are worked in order as if-else conditionals in the foreach-loop. For the current the method, it should return 'E' and breakout of the loop at the first if condition . Instead the program goes through the whole loop and returns that final else statement? Why is it not identifying the match of the first if-condition?
The loop should loop through each of the 8 list item until there is a match.
1) checkmate which will return the winning move if there is one available
2) if there is not a checkmate move this move will check if opponent has a checkmate move and block the path
3)link two If there is not a checkmate to win or to block this will place a second piece to link in a winning path
4) Place initial randomly on one of the four corners or middle for first two rounds
foreach (var item in dalist)
{
if (item.Where(x => x == '+').Count() == 2)
return new string(item.Where(x => x != '+').ToArray());
else if (item.Where(x => x == '-').Count() == 2)
return new string(item.Where(x => x != '-').ToArray());
else if (item.Where(x => x == '+').Count() == 1)
return item.Where(x => x != '+').First().ToString();
else
{
Random random = new Random();
int rList = random.Next(0, 3);
int rPosition = random.Next(0, 2);
return dalist.ElementAt(rList).GetValue(rPosition).ToString();
}
}
return "AA";
}
1 Answer
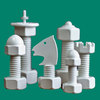
Steven Parker
230,274 PointsYou say that given these starting conditions, checkmate "should return 'E' and breakout of the loop at the first if condition".
But the first item in dalist list is { board[3], board[4], board[5] }
, which is 'A', 'S', 'D'
. This means none of the tests looking for '+'
or '-'
will pass, and the function will return from inside the final else. So it's doing exactly what it should for these conditions.
If you want it to "loop through each of the 8 list item until there is a match", you'll need to make some changes. For one thing, in the current code, every test condition leads to a return, and so does the else. This means it is not possible for it to process anything but the first item, no matter what the item contains. For the loop to get beyond the first item, there must be some path through the inner loop that does not reach a return
.
I hope that helps you get it going, and happy coding! -sp