Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial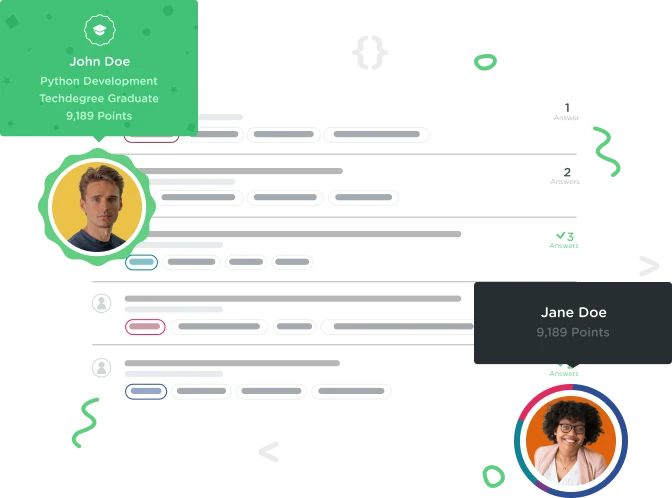
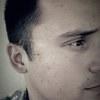
Brendon Brooks
8,802 PointsForeign Key Error
For some reason I keep on getting an error that states that the foreign key in which I'm using does not exist? Did I miss a step here?
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
Students = new List<Student>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<Student> Students { get; set; }
}
}
namespace Treehouse.CodeChallenges
{
public class CourseStudent
{
public int Id { get; set; }
public int CourseId { get; set; }
[ForeignKey("CourseId")]
public int StudentID { get; set; }
[ForeignKey("StudentId")]
public decimal Grade { get; set; }
public Course Course { get; set; }
public Student Student { get; set; }
}
}
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class Student
{
public Student()
{
Courses = new List<Course>();
}
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public ICollection<Course> Courses { get; set; }
}
}
3 Answers
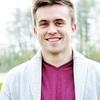
Simon Owerien
12,829 Pointspublic class CourseStudent
{
public int Id { get; set; }
public int CourseId { get; set; }
[ForeignKey("CourseId")]
public int StudentID { get; set; }
[ForeignKey("StudentId")]
public decimal Grade { get; set; }
public Course Course { get; set; }
public Student Student { get; set; }
}
You are using the DataAnnotations on the wrong line. You put C#-Attributes ahead of the variable you want to apply the attribute to:
[ForeignKey("CourseId")]
public int CourseId { get; set; }
[ForeignKey("StudentId")]
public int StudentID { get; set; }
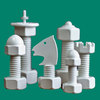
Steven Parker
231,268 PointsI passed this challenge without using the data annotations.
If you did need them, you'd also need a "using System.ComponentModel.DataAnnotations;
", but I tried that and it didn't help. So just don't use the annotations.
Addendum: the course asked for a field named "StudentId" (with a lower-case "d"), but you wrote "StudentID" (with a capital "D").
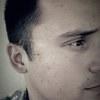
Brendon Brooks
8,802 PointsSame here. I've tried both methods and they are not passing
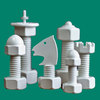
Steven Parker
231,268 PointsDon't forget to fix the spelling of "StudentId".
Steven Parker
231,268 PointsSteven Parker
231,268 PointsI almost mentioned that too, but fixing it still won't pass the challenge.