Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial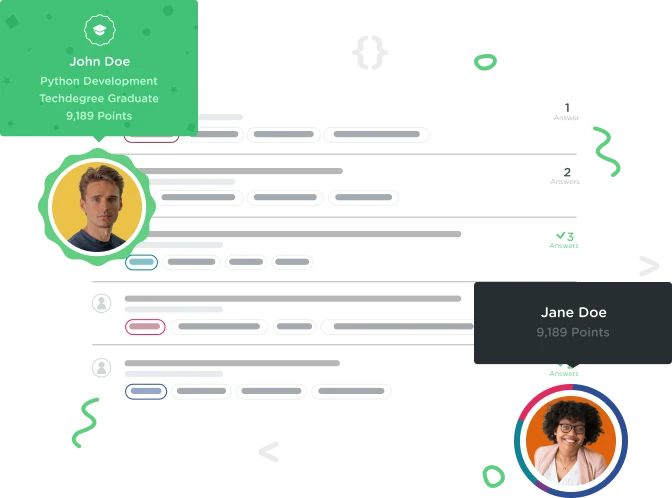

daniwao
13,125 PointsForm resubmission Issue
I have a simple form made that I'm submitting to another php page. The issue that I'm having is that when I get redirected to the new page, if I click refresh, the form gets submitted again. I know that Randy had some type of lesson on this but I can't find the video.
Here's my code where the form is getting created and submitted.
<?php header('Location: index.php'); die(); ?> <body>
<h1>BLOGGING</h1>
<form action="index.php" method="post">
Title: <input type="text" name="title"><br/>
Blog: <textarea rows="10" cols="60" name="post"></textarea><br/><br/>
<input type="submit" style="margin-left: 40%";>
</form>
</body>
Here's my index.php page that is receiving the form and then outputting it.
<div id="main" class="container-fluid"> <h1>My Simple Blog</h1> <div id="blogPosts"> <!-- include "includes.php" --> <?php include "includes.php";
if (mysqli_connect_errno()) {
echo "Failed to connect to the database.";
}
//escape variables for security reasons
$title = mysqli_real_escape_string($connection, $_POST['title']);
$post = mysqli_real_escape_string($connection, $_POST['post']);
$sql="INSERT INTO blog_posts (title, post) VALUES ('$title', '$post')";
if (!mysqli_query($connection, $sql)){
die ('Error: ' . mysqli_error($connection));
}
echo "Entry has been recorded.";
mysqli_close($connection);
$blogPosts = GetBlogPosts();
foreach ($blogPosts as $post) {
echo "<div class='post'>";
echo "<h2>" . $post["title"] . "</h2>";
echo "<p>" . $post["post"] . "</h1>";
echo "<span class='footer'>Posted By: " . $post["author_id"] . " Posted On: " . $post["date_posted"] . "</span>";
echo "</div>";
}
?>
</div>
</div>
1 Answer

Michael Collins
433 PointsI'm not sure I'd want to prevent re-submit. However, if I did, then probably what I'd do is ....
- On the originating page, create a hidden field with a randomly generated token:
<input type="hidden" name="form_token" value="9ba8429f096021148c6c81fcb2b3a640" />
Your token generation function looks like this
<?php
function get_form_token() {
return md5(microtime());
}
?>
Then, on the form processing page, you need to write a couple of functions to Save and Retrieve the form token to see if it's been submitted previously
function save_form_token($token) {
// code to save token
// you can write it to file
// or you can save it to MySQL DB
}
function token_match() {
// Retrieve all form tokens
// Loop through all of the to see if you have a match
foreach ($tokens as $t) {
if ($t == $token) {
return TRUE;
}
}
return FALSE;
}
function form_submitted() {
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
return TRUE;
} else {
return FALSE;
}
}
$form_token = $_REQUEST['form_token'];
if (form_submitted()) {
if (token_match()) {
// Stop processing, this exact form has already been submitted.
exit;
}
save_form_token($form_token);
// Do your normal form processing here.
}