Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial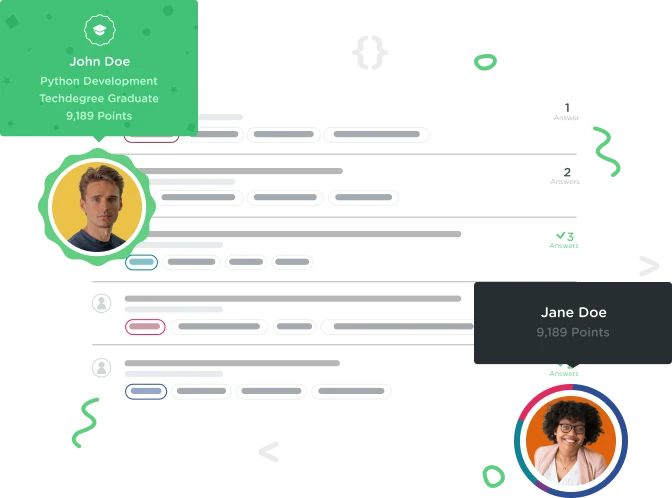

ziaul sarker
3,652 Pointsform validation using javaScript
form validation not working not sure what im doing wrong when i hit submit nothing happens im not sure what i am doing wrong please help
<!DOCTYPE html>
<html>
<head>
<title>form validation</title>
<link rel="stylesheet" type="text/css" href="css/form.css">
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<script src="js/form.js"></script>
</head>
<body>
<div id="container">
<header>
<h1>simple Javascript quiz</h1>
<p>test your knoledge in <strong>JavaScript</strong> fundimentals
</p>
</header>
<section>
<div id="results"></div>
<form name="quizForm" onSubmit="return submit()">
<h3>1. in which html element do we put javascript code</h3>
<input type="radio" name="q1" value="a" id="q1a">a.<js> <br>
<input type="radio" name="q1" value="b" id="q1b">b.<script><br>
<input type="radio" name="q1" value="c" id="q1c">c.<body><br>
<input type="radio" name="q1" value="d" id="q1d">d.<link><br>
<h3>2. which HTML element is used to refremce to an external javaScript file</h3>
<input type="radio" name="q2" value="a" id="q2a">a. src <br>
<input type="radio" name="q2" value="b" id="q2b">b. atr<br>
<input type="radio" name="q2" value="c" id="q2c">c. link<br>
<input type="radio" name="q2" value="d" id="q2d">d. java<br>
<h3>3. how do you put "alert" message with the string "hello"in javaScript</h3>
<input type="radio" name="q3" value="a" id="q3a">a. console.log("hello")<br>
<input type="radio" name="q3" value="b" id="q3b">b. document.write("hello")<br>
<input type="radio" name="q3" value="c" id="q3c">c. confirm("hello")<br>
<input type="radio" name="q3" value="d" id="q3d">d. alert("hello")<br>
<h3>4. javaScript is directly related to java programing</h3>
<input type="radio" name="q4" value="a" id="q4a">a.true <br>
<input type="radio" name="q4" value="b" id="q4b">b.false<br>
<h3>5. what is a variable in javaScript</h3>
<input type="radio" name="q5" value="a" id="q5a">a. a place to store data <br>
<input type="radio" name="q5" value="b" id="q5b">b. a "string"<br>
<input type="radio" name="q5" value="c" id="q5c">c. a number<br>
<input type="radio" name="q5" value="d" id="q5d">d. bollean value<br>
<br>
<br>
<br>
<input type="submit" value="submit">
<div class="fotter">©form submission by ziaul sarker</div>
</form>
</section>
</div>
</body>
</html>
function submit() {
var total = 5;
var score = 0;
// get user input
var q1 = document.forms["quizForm"]["q1"].value;
var q2 = document.forms["quizForm"]["q2"].value;
var q3 = document.forms["quizForm"]["q3"].value;
var q4 = document.forms["quizForm"]["q4"].value;
var q5 = document.forms["quizForm"]["q5"].value;
// validation
for (i=1; i < total; i++) {
if (eval("q" + i) == null || eval("q" + 1) === ""){
alert("you missed question " + i);
return false;
}
}
// set correct answers
var answers = ["b", "a", "d", "b", "a" ];
// check answer
for (i=1; i<total; i++) {
if (eval("q" + i) === answers[i - 1]){
score++;
}
}
// display results in html
var results = document.getElementById("results")
results.innerHTML = "<h3>you scored <span>" + answers + "</span> out of <span> " + total + " </span> </h3>";
return false;
}
1 Answer

Anders Prytz
26,193 PointsI will try to debug the form validation step by step in how I found errors:
- Change the name of the function to something else than submit. As submit is a reserved work, the function will never run (https://www.w3schools.com/js/js_reserved.asp). call it, e.g. checkform or something completely different :)
- In the validation of the empty answers you are using q+i and q+1. You will need to use i on both.
- In both for loops you are ending one to early.(for (i=1; i < total; i++) ) will only create i less than total (e.g. 4), but you will need to validate ut to 5. You will need to loop one more time.
- In the printout of the result you are printing the answers and not the score.
Hope this helps :)