Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial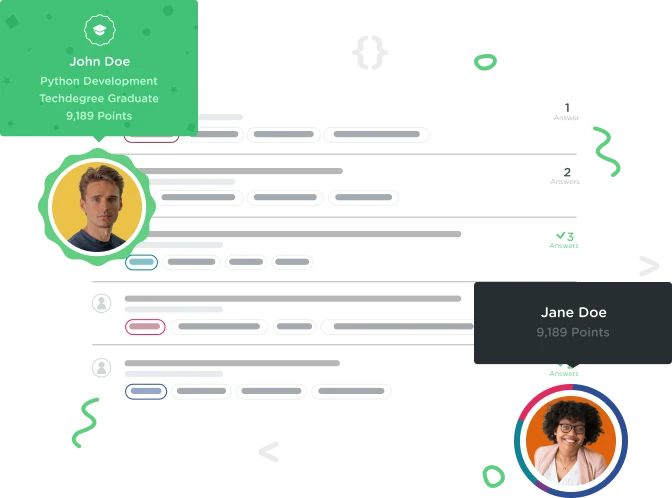

Tony Brackins
28,766 Pointsformat() argument after ** must be a mapping, not list
Create a function named string_factory that accepts a list of dictionaries and a string. Return a new list build by using .format() on the string, filled in by each of the dictionaries in the list.
Here's my code: Please help!
dicts = [
{'name': 'Michelangelo',
'food': 'PIZZA'},
{'name': 'Garfield',
'food': 'lasanga'},
{'name': 'Walter',
'food': 'pancakes'},
{'name': 'Galactus',
'food': 'worlds'}
]
string = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(dicts, string):
strings = []
for items in dicts:
string.format(**dicts)
return strings
print(string_factory(dicts, string))
6 Answers
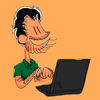
Juan Martin
14,335 PointsHello my friend!
Here you can see 2 possible ways of doing it:
First:
def string_factory(dictionary, strings):
string_list = []
for i in range(len(dictionary)):
string_list.append(strings.format(**dictionary[i]))
return string_list
Second:
def string_factory(dictionary, strings):
string_list = []
for element in dictionary:
string_list.append(strings.format(**element))
return string_list
Hope this helps!
Have an awesome night :)

Spencer Johnson
19,708 Pointsthis worked for me:
def string_factory(dicts, string):
formatted = []
for data in dicts:
new_dict = data
formatted.append(string.format(**new_dict))
return formatted
print(string_factory(dicts, string))

Maxim Andreev
24,529 PointsCan someone dumb down '**' please, I understand how to use **kwargs, and *args for functions but how is the double asterick being used in this case?
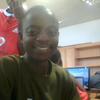
beven nyamande
9,575 Pointsdouble asterisks are for unpacking items in a dictionary

yanni jain
693 Pointsdicts = [ {'name': 'Michelangelo', 'food': 'PIZZA'}, {'name': 'Garfield', 'food': 'lasanga'}, {'name': 'Walter', 'food': 'pancakes'}, {'name': 'Galactus', 'food': 'worlds'} ]
string = "Hi, I'm {name} and I love to eat {food}!" def string_factory(str,dict): new_list= [ string.format(dicts[0]), string.format(dicts[1]), string.format(dicts[2]), string.format(dicts[3]) ]
return new_list
i dont know where i am wrong it is giving an iteration error in ( new_list=[ )

yanni jain
693 Pointsplease reply and correct me!!
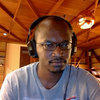
Daniel Muchiri
15,407 PointsThis worked for me:
def string_factory(dicts, string):
string_list = []
for a_dict in dicts:
string_list.append(string.format(**a_dict))
return string_list