Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial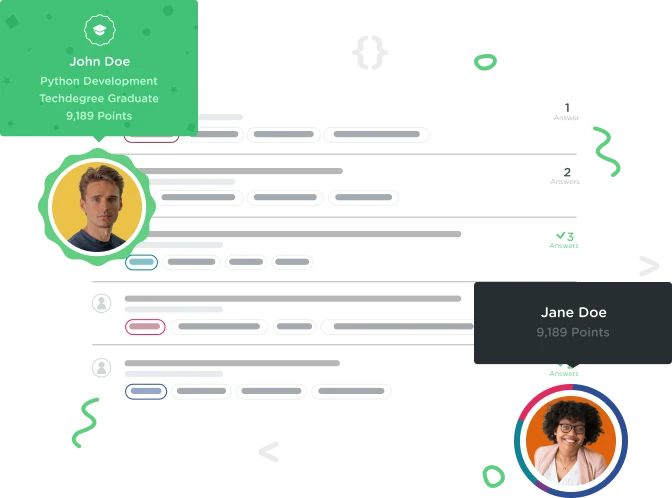

Michael McElyea
329 PointsFormat Exception
namespace Treehouse
{
class Program
{
static void Main()
{
int runningTotal = 0;
bool keepGoing = true;
while (keepGoing)
{
// Prompt the user for the minutes exercised
System.Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: ");
string entry = System.Console.ReadLine();
if(entry == "quit")
{
keepGoing = false;
}
else
{
try
{
int minutes = int.Parse(entry);
if(minutes <= 0)
{
System.Console.WriteLine(minutes + " is not a valid entry");
continue;
}
else if(minutes <= 10)
{
System.Console.WriteLine("Better than nothing right?");
}
else if(minutes <= 30)
{
System.Console.WriteLine("Keep it up Hot Stuff!");
}
else if(minutes <= 60)
{
System.Console.WriteLine("You must be traing to be a ninja warrior");
}
else
{
System.Console.WriteLine("Now you're just showing off");
}
// Add minutes exercised to total
runningTotal = runningTotal + minutes;
// Display total minutes
System.Console.WriteLine("You've exercised " + runningTotal + " minutes");
}
catch(FormatException)
{
System.Console.WriteLine("That is not a valid input.");
continue;
}
}
}
// Repeat until the user quits
System.Console.WriteLine("Goodbye");
}
} }
When I compile the code I get the following error:
"Program.cs(57,22): error CS0246: The type or namespace name FormatException' could not be found. Are you missing
System' using directive?
Compilation failed: 1 error(s), 0 warnings "
I noticed in a few of the other comments people have been modifying the catch parameter with an "e" or "ex". Can anyone explain the difference please and/or why I'm encountering this particular issue? Is it because I am defining class before Name Space?
1 Answer

Keli'i Martin
8,227 PointsIt looks like you don't have a using System;
directive at the top of your file. This means you'll have to include the System
namespace when using FormatException
. Try this:
catch(System.FormatException)
Hope this helps!
Michael McElyea
329 PointsMichael McElyea
329 PointsThanks, that is what I needed. Felt it had something to do with not defining system up front...
Hugo da Silva Ribas
7,666 PointsHugo da Silva Ribas
7,666 PointsYes. I also noticed that. Best thing to do, as Keli said, is to declare 'using System' on the top of the file since you'll be using the .Net framework all the time.
Here's a list of the System Namespace from .NET that will be using: https://msdn.microsoft.com/en-us/library/system(v=vs.110).aspx
Correct me if i'm wrong.
=)