Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial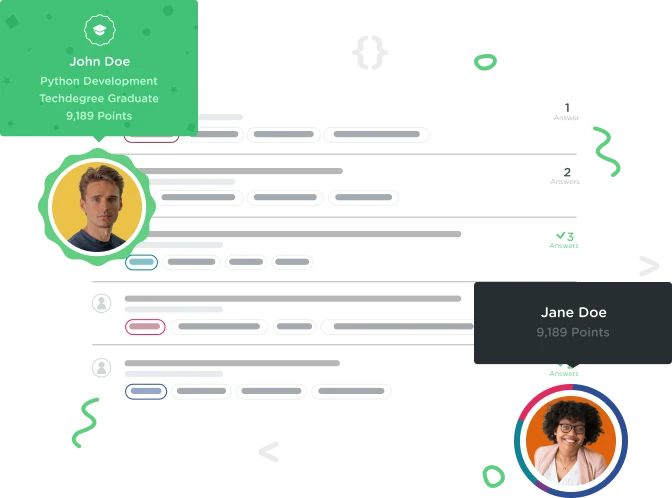

Haoz Bach Ly
3,709 PointsFormat of function inside addEventListener
Well my question is that why I can't use the changeColor function in the addEventListener as the same form as in the alert command.
As you can see, when I had logged 'button.addEventListener('click', changeColor());' instead of the below code, there will be an error message appearing in the console: 'Uncaught TypeError: Failed to execute 'addEventListener' on 'EventTarget': The callback provided as parameter 2 is not a function.'
Here all of my scripts
//
const head = document.getElementById("myHeading");
const button = document.getElementById('button_1');
let textField = document.getElementById('textInput');
//
function changeColor() {
return head.style.color = textField.value;
}
//
button.addEventListener('click', changeColor);
alert(changeColor());
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<button id = 'button_1'>Color</button>
<input type = 'text' id = 'textInput'>
<script src = 'script2.js'></script>
</body>
</html>
3 Answers
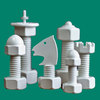
Steven Parker
229,732 PointsWhen you set up a callback, you want to pass the function itself and not the result of calling it. So use the function name but don't put the parentheses after it:
button.addEventListener('click', changeColor);
Now the function will run when the button is clicked, where before it ran (just once) while the handler was being set up.. This is what you had in the original example.
I did not see any cause for error in the original example, but the "alert" doesn't do anything useful since it invokes the change method before the user has a chance to fill in the text box. Perhaps you intended to use the alert to confirm the color change? For that you might put it in the callback function and use the color as the message:
function changeColor() {
head.style.color = textField.value;
alert(head.style.color);
}
Also note that the "return" is not needed in the callback;

Jazz Jones
8,535 PointsIt worked for me without any errors when I copied it to my workspaces. But I recommend putting alert into the changeColor function like this:
function changeColor() {
alert(head.style.color = textField.value);
return head.style.color = textField.value;
}

Haoz Bach Ly
3,709 PointsActually, I mean this:
//
const head = document.getElementById("myHeading");
const button = document.getElementById('button_1');
let textField = document.getElementById('textInput');
//
function changeColor() {
return head.style.color = textField.value;
}
//?????????
button.addEventListener('click', changeColor());
alert(changeColor());
And beside, could you recommend any property to change the font size heading using DOM?

Jazz Jones
8,535 PointsTake out the parenthesis in the callback of the event handler. The first one you posted was correct.
button.addEventListener('click', changeColor);
to change fontsize in javascript is basically the same as the changeColor function except style.fontSize = "insert value here"

Haoz Bach Ly
3,709 PointsOk, but I need to enclose the unit next to the number. For example:
//
const head = document.getElementById("myHeading");
const button = document.getElementById('button_1');
const button_2 = document.getElementById('button_2');
const button_3 = document.getElementById('button_3');
const allParagraph = document.getElementsByTagName('p');
let textField = document.getElementById('textInput');
let chooseStyle = document.getElementById('otherStyle');
//
head.style.size = chooseStyle.value;
function changeColor() {
return head.style.color = textField.value;
}
//
button.addEventListener('click', changeColor);
button_2.addEventListener('click', () => head.style.color = 'black');
//
allParagraph[1].style.color = 'red';
button_3.addEventListener('click', () => allParagraph[1].style.fontSize = ''+ chooseStyle.value +'px');
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<p id = 'second'>Second paragraph</p>
<button id = 'button_1'>Color</button>
<button id = 'button_2'>Reset</button>
<button id = 'button_3'>Size apply</button>
<input type = 'text' id = 'textInput'>
<input type = 'text' id = 'otherStyle'>
<script src = 'script2.js'></script>
</body>
</html>
Thanks for help!
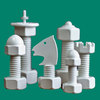
Steven Parker
229,732 PointsI'm not sure what you mean by "enclose the unit next to the number". The third button handler code is successfully adding the unit "px" to the entered number.
Perhaps you should start a new question for this issue.
Haoz Bach Ly
3,709 PointsHaoz Bach Ly
3,709 PointsI have just used the alert for demonstrating the difference.